
January 9, 2023 06:36 by
Peter
Analyzers are tools that analyze source code and provide feedback to developers in the form of warnings and suggestions for improvement. In ASP.NET Core, analyzers can help you identify and fix issues in your code before you deploy your application. In this article, we'll explore how to use analyzers in ASP.NET Core and provide some examples of how they can be used to improve your code.
Using Analyzers in ASP.NET Core
To use analyzers in ASP.NET Core, you first need to install the necessary NuGet packages. There are many NuGet packages available that contain analyzers for different purposes, such as security, performance, and best practices.
To install the NuGet packages for analyzers, you can use the following command,
dotnet add package Microsoft.AspNetCore.Mvc.Analyzers
Once you have installed the necessary NuGet packages, the analyzers will automatically run whenever you build your project. If the analyzers find any issues in your code, they will display warnings in the Error List window in Visual Studio.
Examples of Analyzers
Here are some examples of analyzers that you can use in ASP.NET Core,
Security Analyzers
The ASP.NET Core Security analyzers can help you identify potential security vulnerabilities in your code. For example, if you are using cookies to store sensitive information, the analyzer will warn you if you have not set the HttpOnly flag on the cookie. This flag prevents the cookie from being accessed by client-side script, which can help protect against cross-site scripting (XSS) attacks.
Here's an example of a warning generated by the Security analyzer,
warning ASP0005: It is recommended to set the 'HttpOnly' flag on cookies.
To fix this issue, you can set the HttpOnly flag on the cookie by using the following code,
Response.Cookies.Append("myCookie", "myValue", new CookieOptions { HttpOnly = true });
Performance Analyzers
The ASP.NET Core Performance analyzers can help you identify potential performance issues in your code. For example, if you are using the HttpClient class to make HTTP requests, the analyzer will warn you if you are not disposing of the HttpClient instance when you are finished with it.
Here's an example of a warning generated by the Performance analyzer,
warning ASP0006: 'HttpClient' should be disposed.
'HttpClient' is IDisposable and should be disposed before all references to it are lost.
To fix this issue, you can dispose of the HttpClient instance by using a using statement, like this,
using (var client = new HttpClient()){
// Make the HTTP request
}
Here's an example of how you can use Microsoft.AspNetCore.Mvc.Analyzers analyzer to catch issues in a Razor view,
@model MyViewModel
@{
ViewData["Title"] = "My Page";
}
<h1>@ViewData["Title"]</h1>
<p>Welcome to my page!</p>
In this example, the analyzer might report a suggestion to use the ViewBag property instead of ViewData, as ViewBag is a more convenient way to pass data from the controller to the view. The analyzer might also report a warning if you try to access an undefined key in the ViewData dictionary, such as ViewData["UnknownKey"].
Analyzers can be extremely useful for catching issues in your code as you write it, saving you time and effort in debugging and testing. There are many different analyzer packages available for ASP.NET Core, each with its own set of rules and checks. You can choose the analyzers that best fit your needs and enable them in your project to ensure the highest quality of your code.
I hope this helps you understand analyzers in ASP.NET Core in .NET 6 and how you can use them to improve the quality of your code. Let me know if you have any questions!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
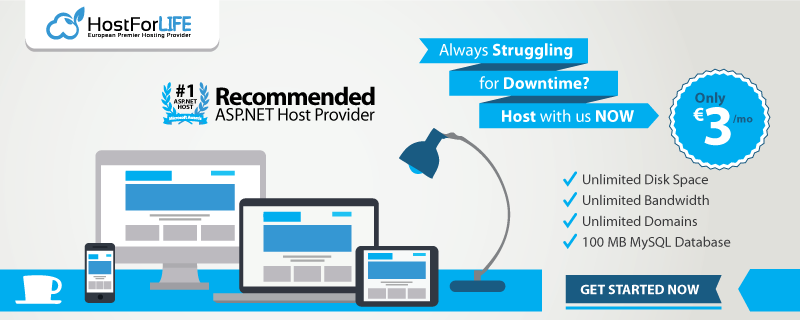