In this post, we will look at the best techniques for increasing the speed of your.NET core application.
It is critical to optimize the performance of your.NET Core application to ensure that it provides a responsive and efficient user experience. Here are some suggested practices for optimizing the performance of your.NET Core application:
1. Make Use of Profiling Tools
Profiling tools like as Visual Studio Profiler, JetBrains dotTrace, and PerfView can assist you in locating bottlenecks and performance issues in your code.
Using a stopwatch to measure method performance is an example.
using System;
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// Code to profile
stopwatch.Stop();
Console.WriteLine($"Elapsed Time: {stopwatch.ElapsedMilliseconds} ms");
}
}
2. Caching
Implement caching for frequently accessed data to reduce database load and improve response times. .NET Core has a variety of caching methods, including as in-memory caching and distributed caching using libraries such as Memory Cache or Redis.
Example: Using MemoryCache for in-memory caching.
using System;
using Microsoft.Extensions.Caching.Memory;
class Program
{
static void Main(string[] args)
{
IMemoryCache cache = new MemoryCache(new MemoryCacheOptions());
// Store data in cache
cache.Set("myKey", "myValue", TimeSpan.FromMinutes(10));
// Retrieve data from cache
if (cache.TryGetValue("myKey", out string value))
{
Console.WriteLine($"Cached Value: {value}");
}
}
}
3. Database Optimization
Optimize your database queries using proper indexing, query optimization techniques, and stored procedures.
Implement connection pooling to reuse database connections and reduce overhead.
Example: Using proper indexing in Entity Framework Core.
using System;
using System.Linq;
using Microsoft.EntityFrameworkCore;
class Program
{
static void Main(string[] args)
{
var options = new DbContextOptionsBuilder<MyDbContext>()
.UseSqlServer(connectionString)
.Options;
using (var context = new MyDbContext(options))
{
// Apply indexing to optimize queries
var results = context.Orders.Where(o => o.CustomerId == 123).ToList();
}
}
}
4. Asynchronous Programming
Use asynchronous programming (async/await) to offload CPU-bound work and improve responsiveness, especially in I/O-bound scenarios.
Utilize asynchronous database drivers and libraries for improved database performance.
Example: Using async/await to perform I/O-bound tasks asynchronously.
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main(string[] args)
{
using (HttpClient client = new HttpClient())
{
HttpResponseMessage response = await client.GetAsync("https://example.com");
string content = await response.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
}
}
5. Use Entity Framework Core Wisely
If you're using Entity Framework Core for database access, be mindful of the queries it generates. Use eager loading, projections, and optimizations like compiled queries.
Example: Using compiled queries in Entity Framework Core.
using System;
using System.Linq;
using Microsoft.EntityFrameworkCore;
class Program
{
static void Main(string[] args)
{
var options = new DbContextOptionsBuilder<MyDbContext>()
.UseSqlServer(connectionString)
.Options;
using (var context = new MyDbContext(options))
{
var compiledQuery = EF.CompileQuery((MyDbContext db, int customerId) =>
db.Orders.Where(o => o.CustomerId == customerId).ToList());
var results = compiledQuery(context, 123);
}
}
}
6. Memory Management
Minimize object allocations and memory usage. Use value types where appropriate and be cautious with large object graphs.
Utilize the Dispose pattern or use statements for resources like database connections or streams to ensure timely cleanup.
Example: Disposing resources using the Dispose pattern.
using System;
class MyResource : IDisposable
{
private bool disposed = false;
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!disposed)
{
if (disposing)
{
// Release managed resources
}
// Release unmanaged resources
disposed = true;
}
}
~MyResource()
{
Dispose(false);
}
}
7. HTTP Caching
Leverage HTTP caching mechanisms (ETags, Last-Modified headers) to reduce unnecessary data transfers in web applications.
Example: Adding caching headers to HTTP responses.
using Microsoft.AspNetCore.Mvc;
public class MyController : Controller
{
[HttpGet]
[ResponseCache(Duration = 300)] // Cache for 5 minutes
public IActionResult Index()
{
// Generate and return response
}
}
8. Minimize Round-Trips
Reduce the number of HTTP requests and database round-trips. Combine multiple requests when possible.
Example: Combining multiple database queries into a single query.
using System;
using System.Linq;
using Microsoft.EntityFrameworkCore;
class Program
{
static void Main(string[] args)
{
var options = new DbContextOptionsBuilder<MyDbContext>()
.UseSqlServer(connectionString)
.Options;
using (var context = new MyDbContext(options))
{
var orders = context.Orders
.Include(o => o.Customer)
.Include(o => o.Products)
.Where(o => o.CustomerId == 123)
.ToList();
}
}
}
9. Content Delivery Networks (CDNs)
Offload static assets (CSS, JavaScript, images) to CDNs for faster delivery to users.
Example: Using a CDN for delivering static assets.
<!-- Link to static asset on CDN -->
<link rel="stylesheet" href="https://cdn.example.com/styles.css">
10. Compression
Enable GZIP or Brotli compression for HTTP responses to reduce data transfer size.
Example: Enabling GZIP compression for HTTP responses.
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.ResponseCompression;
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.EnableForHttps = true;
options.Providers.Add<GzipCompressionProvider>();
});
}
11. Logging and Tracing
Use logging levels wisely. Avoid excessive logging in production.
Implement distributed tracing to monitor performance across microservices.
Example: Using a distributed tracing library for monitoring.
using Microsoft.ApplicationInsights;
using Microsoft.ApplicationInsights.Extensibility;
var configuration = TelemetryConfiguration.Active;
var telemetryClient = new TelemetryClient(configuration);
using (var operation = telemetryClient.StartOperation<RequestTelemetry>("MyOperation"))
{
// Perform operation
operation.Telemetry.Success = true;
}
12. Code Analysis and Reviews
Regularly review your codebase for performance issues. Use tools like ReSharper or SonarQube for static code analysis.
code analysis and reviews are essential practices for identifying and addressing code quality, maintainability, and performance issues in your .NET Core application. Code analysis involves using automated tools and manual inspection to review your codebase for potential problems, while code reviews involve the collaborative evaluation of code changes by your development team.
Here's a more detailed explanation of code analysis and reviews:
Code Analysis
Code analysis involves using specialized tools to automatically scan your codebase for potential issues, violations of coding standards, and best practices. These tools can help identify bugs, security vulnerabilities, performance bottlenecks, and other code quality concerns.
Benefits of Code Analysis
Consistency: Code analysis enforces coding standards, ensuring a consistent codebase across your application.
Early Detection: It helps catch issues early in the development process, reducing the likelihood of bugs reaching production.
Efficiency: Automated tools can quickly identify common issues, saving time during manual reviews.
Maintainability: Code analysis improves the long-term maintainability of your application by identifying areas that might become problematic.
Example (Using Roslyn Code Analysis)
The Roslyn compiler platform includes built-in analyzers that can be used to perform code analysis in Visual Studio. You can install additional analyzers from NuGet packages.
Example: Applying code analysis recommendations with ReSharper.
// Example of a code analysis warning
public class ExampleClass
{
public void DoSomething(string input)
{
if (input == null) // CA1062: Validate arguments of public methods
{
throw new ArgumentNullException(nameof(input));
}
// Code logic
}
}
13. Parallelism and Concurrency
Utilize parallelism and multithreading for CPU-bound tasks using Parallel class or Task Parallel Library (TPL).
Be cautious with thread synchronization to avoid deadlocks and contention.
Example: Using parallelism for CPU-bound tasks.
Parallel.For(0, 10, i =>
{
// Perform parallelized work
});
14. Resource Optimization
Optimize images and assets for the web to reduce load times.
Minimize the number of external dependencies and libraries.
Example: Optimizing images for the web.
<!-- Optimized image tag -->
<img src="images/my-image.jpg" alt="My Image">
15. Benchmarking and Load Testing
Perform benchmarking and load testing to identify performance bottlenecks and determine how your application performs under different loads.
Benchmarking involves measuring the performance of specific components or functions, while load testing simulates various levels of user traffic to evaluate how well your application handles the load. Both practices help identify bottlenecks, scalability issues, and potential improvements.
Here's a more detailed explanation of benchmarking and load testing:
Benchmarking
Benchmarking involves running specific tests on critical parts of your application to measure their performance. The goal is to establish a baseline and identify areas for improvement. For example, you might benchmark a specific algorithm, database query, or code snippet to compare different implementations and determine which one performs better.
Steps for Benchmarking
- Identify the specific component, function, or operation you want to benchmark.
- Design a set of controlled tests that exercise the component under different scenarios.
- Measure and record performance metrics such as execution time, memory usage, CPU utilization, etc.
- Analyze the results and identify opportunities for optimization.
Example: Using a load testing tool to simulate traffic.
Benchmarking an Algorithm
public class AlgorithmBenchmark
{
public void RunBenchmark()
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// Run the algorithm multiple times and measure the time taken
for (int i = 0; i < 10000; i++)
{
// Call the algorithm being benchmarked
SomeAlgorithm.Perform();
}
stopwatch.Stop();
Console.WriteLine($"Time taken: {stopwatch.ElapsedMilliseconds} ms");
}
}
16. Deployment and Infrastructure
Use containerization (Docker) to ensure consistent deployment environments.
Leverage cloud services and auto-scaling for handling varying traffic loads.
Example: Deploying an application using Docker.
17. Regular Updates
Keep your dependencies, frameworks, and libraries up to date to benefit from performance improvements and bug fixes.
Example: Keeping NuGet packages up to date.
18. Code Profiling and Performance Monitoring
Continuously monitor your application's performance in production using tools like Application Insights, New Relic, or Dynatrace.
Example: Integrating Application Insights for monitoring.
using Microsoft.ApplicationInsights;
using Microsoft.ApplicationInsights.Extensibility;
var configuration = TelemetryConfiguration.Active;
var telemetryClient = new TelemetryClient(configuration);
// Track custom telemetry events
telemetryClient.TrackEvent("CustomEventName");
Here are some additional tips
- Use the latest version of .NET Core.
- Use a lightweight framework.
- Use a good IDE.
- Write clean and well-structured code.
- Use a debugger to find and fix performance problems.
- Monitor the application's performance and make changes as needed.
Remember that the specific optimizations needed for your application may vary based on its nature and requirements. It's important to profile, measure, and test your application to ensure that the changes you make have a positive impact on its performance.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
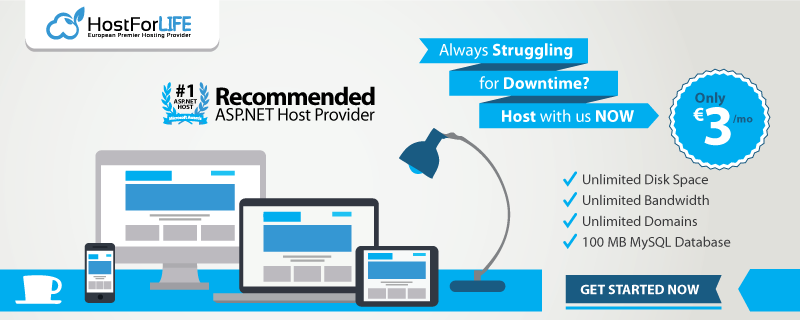