The Random class's GetItems() method is one of the potent new features introduced in.NET 8. Working with randomness should be simpler, more effective, and intuitive with this approach. This post will go over the uses, functionality, and improvements that the GetItems() method may provide to your.NET projects.
Table of Contents
- Introduction to the GetItems() Method
- Basic Usage
- Practical Applications
- Comparing Traditional Methods with GetItems()
- Best Practices
- Conclusion
Overview of the GetItems() Procedure
The Random class in.NET 8 now has a new method called GetItems(). It lets you choose a predetermined number of objects at random from a collection. This can be very helpful in situations where you need to add some unpredictability to your application or shuffle data or create random samples.
Standard Usage
Using the GetItems() function is simple. This is the fundamental syntax:
public static T[] GetItems<T>(this Random random, IList<T> list, int count);
- random: An instance of the Random class.
- list: The collection from which items are to be selected.
- count: The number of random items to select.
Here’s a simple example to illustrate its usage.
Random random = new Random();
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int[] randomNumbers = random.GetItems(numbers, 3);
foreach (var number in randomNumbers)
{
Console.WriteLine(number);
}
In this example, GetItems() selects three random numbers from the numbers list.
Practical Applications
Random Sampling in Surveys
Suppose you're conducting a survey and need to randomly select participants from a list. The GetItems() method makes this easy:
List<string> participants = new List<string> { "Alice", "Bob", "Charlie", "David", "Eve" };
string[] selectedParticipants = random.GetItems(participants, 2);
Console.WriteLine("Selected Participants:");
foreach (var participant in selectedParticipants)
{
Console.WriteLine(participant);
}
Random Shuffling of Cards
In game development, shuffling a deck of cards is a common requirement. Using GetItems(), you can shuffle cards effortlessly:
List<string> deck = new List<string> { "2H", "3H", "4H", ..., "KS", "AS" };
string[] shuffledDeck = random.GetItems(deck, deck.Count);
Console.WriteLine("Shuffled Deck:");
foreach (var card in shuffledDeck)
{
Console.WriteLine(card);
}
Comparing Traditional methods with GetItems()
Before GetItems(), achieving similar functionality required more verbose and less readable code. Here’s how you might have done it traditionally:
Random random = new Random();
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
List<int> selectedNumbers = new List<int>();
HashSet<int> usedIndices = new HashSet<int>();
while (selectedNumbers.Count < 3)
{
int index = random.Next(numbers.Count);
if (usedIndices.Add(index))
{
selectedNumbers.Add(numbers[index]);
}
}
foreach (var number in selectedNumbers)
{
Console.WriteLine(number);
}
Using GetItems(), the same task is simplified.
int[] randomNumbers = random.GetItems(numbers, 3);
foreach (var number in randomNumbers)
{
Console.WriteLine(number);
}
Best Practices
- Validate Parameters: Ensure the count parameter does not exceed the size of the list to avoid exceptions.
- Seed Control: For reproducible results, initialize the Random class with a fixed seed.
- Performance Considerations: For very large collections, be mindful of performance implications when using GetItems() frequently.
Conclusion
The GetItems() method in .NET 8 is a welcome addition for developers who frequently work with random data selections. By providing a concise and efficient way to select random items from a collection, it simplifies code and enhances readability. Whether you’re developing games, conducting surveys, or implementing any feature requiring randomness, GetItems() is a tool that can significantly streamline your development process.
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
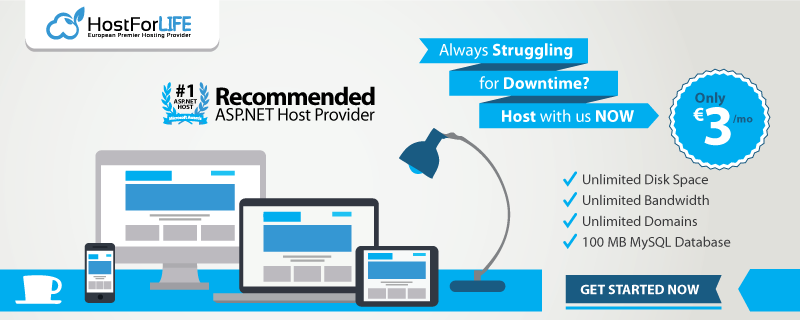