What is Ajax?
Ajax stands for Asynchronous JavaScript and XML, the data transmitted can be JSON, XML, HTML, JavaScript, plain text, etc. Regardless of the type of data, Ajax can send and receive it. Ajax uses a built-in object of all modern browsers called XMLHttpRequest. This object is used to exchange data back and forth between your Web page and a Web server, as shown below.
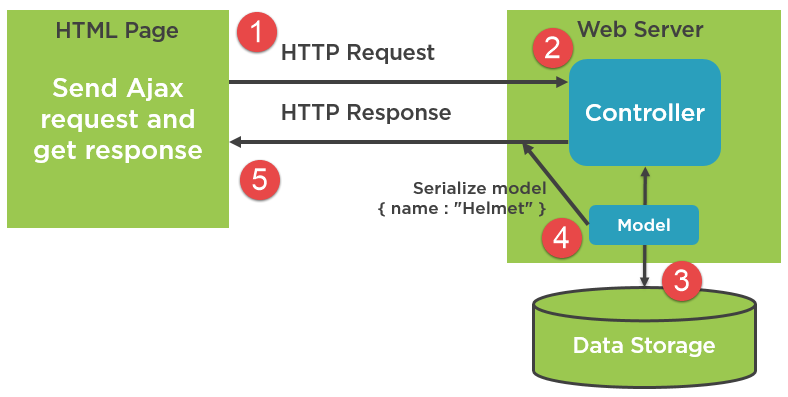
Types of AJAX Call
Ajax Methods |
Description |
get() |
Sends HTTP GET request to load the data from the server. |
Post() |
Sends HTTP POST request to submit or load the data to the server. |
Put() |
Sends HTTP PUT request to update data on the server. |
Delete() |
Sends HTTP DELETE request to delete data on the server. |
STEP 1
Open VS 2022 click on create project as shown below,
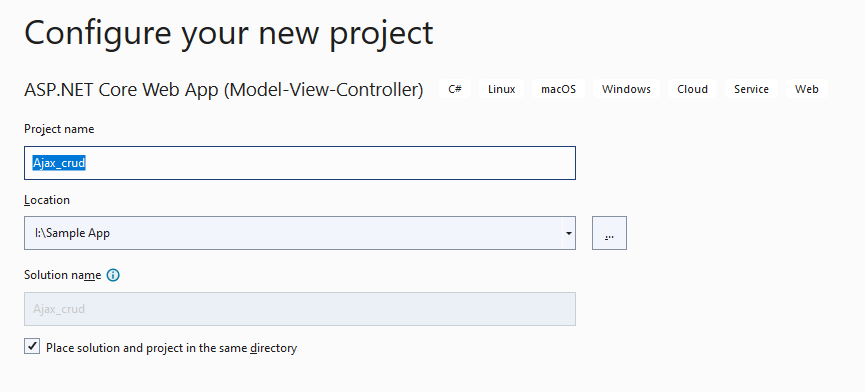
Step 2
Choose the below mentioned template
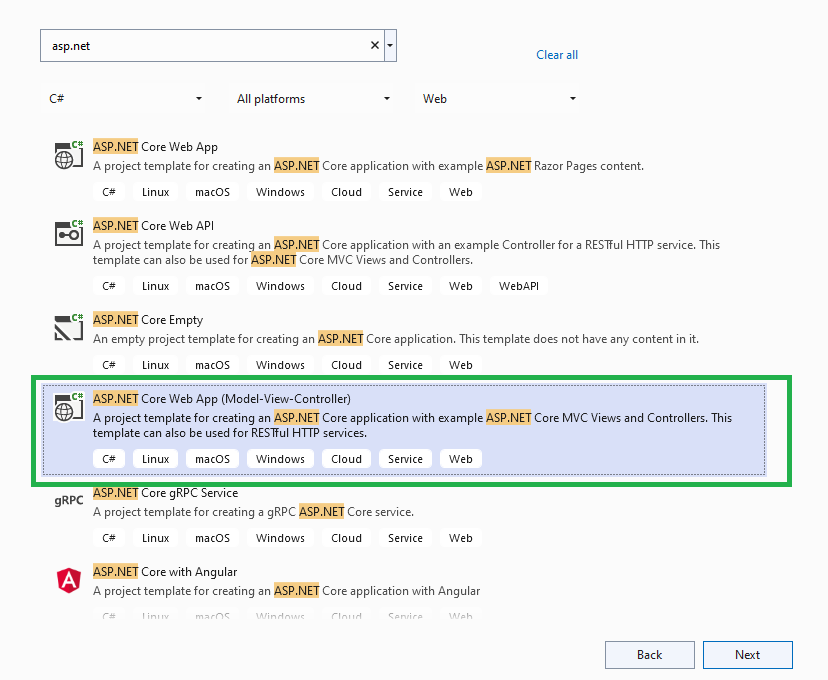
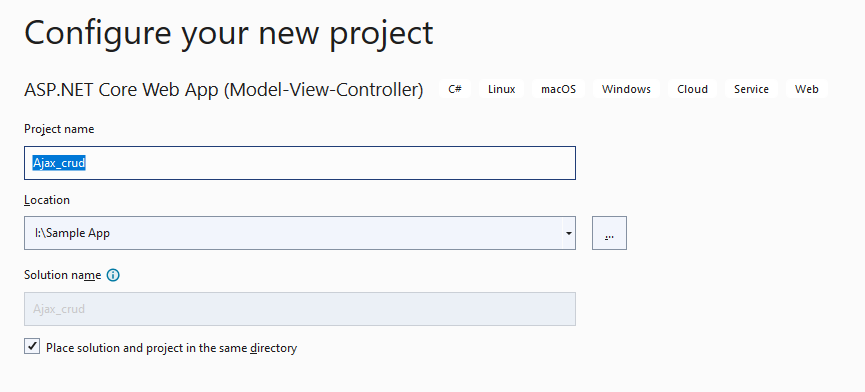
Step 3
Now open the Model folder and add the 2 classes
JsonResponseViewModel
Student Model
This model will be used to communicate during ajax request. Below is the source code defination for both the models
[Serializable]
public class JsonResponseViewModel
{
public int ResponseCode { get; set; }
public string ResponseMessage { get; set; } = string.Empty;
}
public class StudentModel
{
public int Id { get; set; }
public string Name { get; set; } = string.Empty;
public string Email { get; set; } = string.Empty;
}
Step 4
Now add the GET|POST|PUT|DELETE function for the server so that we can perform operations
public class HomeController: Controller {
private readonly ILogger < HomeController > _logger;
public static List < StudentModel > students = new List < StudentModel > ();
public HomeController(ILogger < HomeController > logger) {
_logger = logger;
students = new List < StudentModel > ();
students.Add(new StudentModel() {
Id = 1, Email = "[email protected]", Name = "Ankush"
});
students.Add(new StudentModel() {
Id = 2, Email = "[email protected]", Name = "Rohit"
});
students.Add(new StudentModel() {
Id = 3, Email = "[email protected]", Name = "Sunny"
});
students.Add(new StudentModel() {
Id = 4, Email = "[email protected]", Name = "Amit"
});
}
public IActionResult Index() {
return View(students);
}
[HttpGet]
public JsonResult GetDetailsById(int id) {
var student = students.Where(d => d.Id.Equals(id)).FirstOrDefault();
JsonResponseViewModel model = new JsonResponseViewModel();
if (student != null) {
model.ResponseCode = 0;
model.ResponseMessage = JsonConvert.SerializeObject(student);
} else {
model.ResponseCode = 1;
model.ResponseMessage = "No record available";
}
return Json(model);
}
[HttpPost]
public JsonResult InsertStudent(IFormCollection formcollection) {
StudentModel student = new StudentModel();
student.Email = formcollection["email"];
student.Name = formcollection["name"];
JsonResponseViewModel model = new JsonResponseViewModel();
//MAKE DB CALL and handle the response
if (student != null) {
model.ResponseCode = 0;
model.ResponseMessage = JsonConvert.SerializeObject(student);
} else {
model.ResponseCode = 1;
model.ResponseMessage = "No record available";
}
return Json(model);
}
[HttpPut]
public JsonResult UpdateStudent(IFormCollection formcollection) {
StudentModel student = new StudentModel();
student.Id = int.Parse(formcollection["id"]);
student.Email = formcollection["email"];
student.Name = formcollection["name"];
JsonResponseViewModel model = new JsonResponseViewModel();
//MAKE DB CALL and handle the response
if (student != null) {
model.ResponseCode = 0;
model.ResponseMessage = JsonConvert.SerializeObject(student);
} else {
model.ResponseCode = 1;
model.ResponseMessage = "No record available";
}
return Json(model);
}
[HttpDelete]
public JsonResult DeleteStudent(IFormCollection formcollection) {
StudentModel student = new StudentModel();
student.Id = int.Parse(formcollection["id"]);
JsonResponseViewModel model = new JsonResponseViewModel();
//MAKE DB CALL and handle the response
if (student != null) {
model.ResponseCode = 0;
model.ResponseMessage = JsonConvert.SerializeObject(student);
} else {
model.ResponseCode = 1;
model.ResponseMessage = "No record available";
}
return Json(model);
}
}
Step 5
Switch to the view and add the AJAX Calls functions
@model IEnumerable<ajaxSample.Models.StudentModel>
@{
ViewData["Title"] = "Home Page";
}
@section Scripts{
<script>
$(document).ready(function() {
// GET BY ID
$(".btn-get-student").on("click", function() {
var formData = new FormData();
var studentid = $(this).attr("data-studentid");
var url = '@Url.Action("GetDetailsById", "Home")' + '/' + studentid;
$.ajax({
type: 'GET',
url: url,
contentType: false,
processData: false,
cache: false,
data: formData,
success: function(response) {
if (response.responseCode == 0) {
var student = JSON.parse(response.responseMessage);
$("#email").val(student.Email);
$("#name").val(student.Name);
$("#hdn-student-id").val(student.Id);
}
else {
bootbox.alert(response.ResponseMessage);
}
},
error: errorCallback
});
});
//SAVE
$("#btn-insert-student").on("click", function() {
var formData = new FormData();
formData.append("name", $("#name").val());
formData.append("email", $("#email").val());
$.ajax({
type: 'POST',
url: '@Url.Action("InsertStudent", "Home")',
contentType: false,
processData: false,
cache: false,
data: formData,
success: successCallback,
error: errorCallback
});
});
// UPDATE
$("#btn-update-student").on("click", function() {
var formData = new FormData();
formData.append("id", $("#hdn-student-id").val());
formData.append("name", $("#name").val());
formData.append("email", $("#email").val());
$.ajax({
type: 'PUT',
url: '@Url.Action("UpdateStudent", "Home")',
contentType: false,
processData: false,
cache: false,
data: formData,
success: successCallback,
error: errorCallback
});
});
//DELETE
$("#btn-delete-student").on("click", function() {
var formData = new FormData();
formData.append("id", $("#hdn-student-id").val());
$.ajax({
type: 'DELETE',
url: '@Url.Action("DeleteStudent", "Home")',
contentType: false,
processData: false,
cache: false,
data: formData,
success: successCallback,
error: errorCallback
});
});
function resetForm() {
$("#hdn-student-id").val("");
$("#name").val("");
$("#email").val("");
}
function errorCallback() {
bootbox.alert("Something went wrong please contact admin.");
}
function successCallback(response) {
if (response.responseCode == 0) {
resetForm();
bootbox.alert(response.responseMessage, function() {
//PERFORM REMAINING LOGIC
});
}
else {
bootbox.alert(response.ResponseMessage);
}
};
});
</script>
}
<div class="text-center">
<h1 class="display-4">Welcome To ASP.NET CORE AJAX Demo</h1>
<hr />
</div>
<div class="row">
<div class="col-sm-12">
<form>
<input type="hidden" id="hdn-student-id" />
<div class="row g-3">
<div class="col-sm-6">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" placeholder="Enter your email">
<div class="invalid-feedback">
Please enter a valid email address for shipping updates.
</div>
</div>
<div class="col-sm-6">
<label for="lastName" class="form-label">Name</label>
<input type="text" class="form-control" id="name" placeholder="Enter Your Name" value="" required="">
<div class="invalid-feedback">
Name is required.
</div>
</div>
</div>
<table class="table">
<tbody>
<tr>
<td> <a href="javascript:void(0)" class="btn btn-primary" id="btn-insert-student">Save Student</a></td>
<td>
<a href="javascript:void(0)" class="btn btn-info" id="btn-update-student">Update Student</a>
</td>
<td>
<a href="javascript:void(0)" class="btn btn-danger" id="btn-delete-student">Delete Student</a>
</td>
</tr>
</tbody>
</table>
</form>
</div>
<br />
</div>
<div class="row">
<div class="col-md-12">
<table class="table table-bordered">
<thead>
<tr>
<td>#</td>
<td>Name</td>
<td>Email</td>
<td>Action</td>
</tr>
</thead>
<tbody id="student-list">
@{
foreach (var student in Model)
{
<tr>
<td>@student.Id</td>
<td>@student.Name</td>
<td>@student.Email</td>
<td>
<a href="javascript:void(0)" data-studentid="@student.Id" class="btn btn-success btn-get-student">Get Student</a>
</td>
</tr>
}
}
</tbody>
</table>
</div>
</div>
RUN the code and you will see the below mentioned O/P,
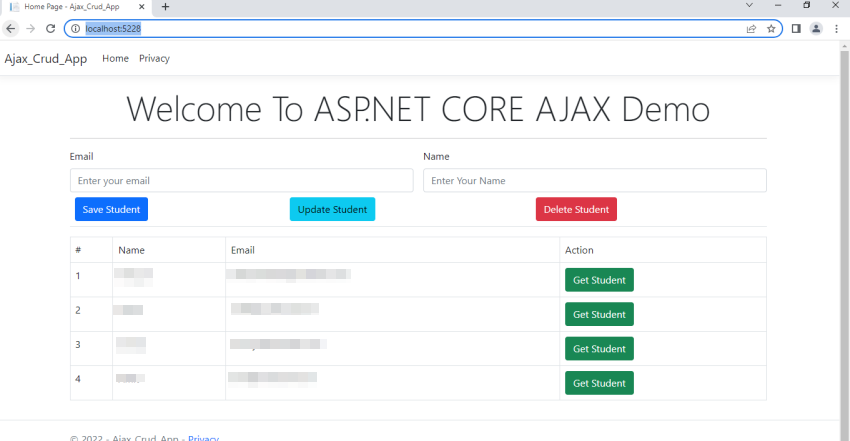
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
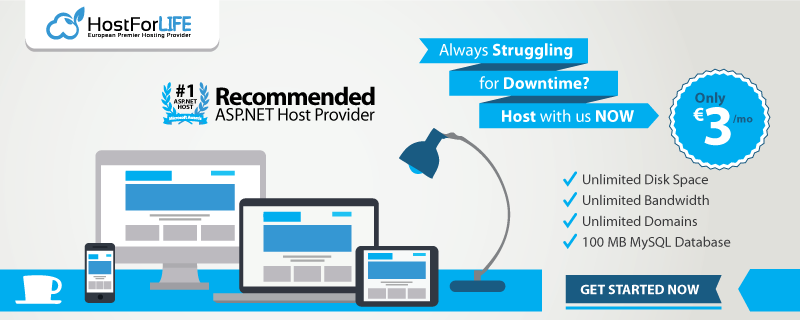