Serialization is the process of transforming an item into a format (XML, JSON, SOAP, or binary) that is suitable for easy storage or transmission. The opposite procedure, known as deserialization, transforms the serialized data back into an object.
Types of Serialization Process
- XML Serialization
- JSON Serialization
- Binary Serialization
- SOAP Serialization
1. XML Serialization
XML serialization in .NET involves the process of transforming an object into an XML format, which facilitates easy storage, transfer, or sharing across different systems. In contrast to binary or SOAP serialization, XML serialization primarily focuses on the public fields and properties of an object, thereby providing a more straightforward and transparent method for data representation.
Features
- Transform an object into XML format.
- The predominant form of serialization is utilized for interoperability.
- Public properties and fields undergo serialization, while private ones remain excluded.
- Frequently employed in web services, configuration files, and for storage that is easily readable by humans.
Step 1. Create a class for XML serialization like the one below.
EmployeeDetails.cs
public class EmployeeDetails
{
public string Name { get; set; }
public int Age { get; set; }
public string Address { get; set; }
public string Hobbies { get; set; }
public string EmployeeType { get; set; }
}
Step 2. Serialize the class EmployeeDetails.cs and save it according to the specified path.
public partial class MainWindow : Window
{
EmployeeDetails employee;
// Specify the file path where the XML file will be saved
string filePath = @"C:\Users\peter\Desktop\Peter\SerailizedPath\"; // Change this to your desired location
public MainWindow()
{
InitializeComponent();
employee = new EmployeeDetails
{
Name = "Peter",
Age = 30,
Address = "London",
EmployeeType = "Permanent",
Hobbies = "Cricket"
};
}
private void XmlSerialization_Click(object sender, RoutedEventArgs e)
{
// Create the XmlSerializer for the EmployeeDetails type
XmlSerializer serializer = new XmlSerializer(typeof(EmployeeDetails));
// Serialize the object to the specified location
using (StreamWriter writer = new StreamWriter(Path.Combine(filePath, "Employee.xml")))
{
serializer.Serialize(writer, employee);
}
}
}Step 3. DeSerialize the class EmployeeDetails.cs like below.
private void XmlDESerialization_Click(object sender, RoutedEventArgs e)
{
XmlSerializer serializer = new XmlSerializer(typeof(EmployeeDetails));
using (StreamReader reader = new StreamReader(Path.Combine(filePath, "Employee.xml")))
{
EmployeeDetails employeeDetails = (EmployeeDetails)serializer.Deserialize(reader);
MessageBox.Show($"Name: {employeeDetails.Name}, Age: {employeeDetails.Age}, Address: {employeeDetails.Address}, EmployeeType: {employeeDetails.EmployeeType}, Hobbies: {employeeDetails.Hobbies}");
}
}
2. JSON Serialization
In C#, JSON serialization is the process of transforming an object into a JSON string, while deserialization involves converting a JSON string back into an object. The System.Text.Json or Newtonsoft.Json libraries can be utilized for these operations.Features
- Transform an object into JSON format.
- It is commonly utilized for web APIs, configuration files, and efficient data storage.
- JSON serialization is supported by both the System.Text.Json and Newtonsoft.Json libraries.
Step 1. Create a class for JSON serialization like the one below.
EmployeeDetails.cs
public class EmployeeDetails
{
public string Name { get; set; }
public int Age { get; set; }
public string Address { get; set; }
public string Hobbies { get; set; }
public string EmployeeType { get; set; }
}
Step 2. Serialize the EmployeeDetails.cs class into JSON format and save it to the specified location based on your requirements.
public partial class MainWindow : Window
{
EmployeeDetails employee;
// Specify the file path where the json file will be saved
string filePath = @"C:\Users\peter\Desktop\Peter\SerailizedPath\"; // Change this to your desired location
public MainWindow()
{
InitializeComponent();
employee = new EmployeeDetails
{
Name = "Peter",
Age = 30,
Address = "London",
EmployeeType = "Permanent",
Hobbies = "Cricket"
};
}
private void JsonSerialization_Click(object sender, RoutedEventArgs e)
{
string jsonString = JsonConvert.SerializeObject(employee, Formatting.Indented);
File.WriteAllText(Path.Combine(filePath, "Employee.json"), jsonString);
Debug.WriteLine("Object serialized to employee.json");
}
private void JsonDESerialization_Click(object sender, RoutedEventArgs e)
{
string jsonString = File.ReadAllText(Path.Combine(filePath, "Employee.json"));
EmployeeDetails employee = JsonConvert.DeserializeObject<EmployeeDetails>(jsonString);
MessageBox.Show($"Name: {employee.Name}, Age: {employee.Age}, Address: {employee.Address}, EmployeeType: {employee.EmployeeType}, Hobbies: {employee.Hobbies}");
}
}
3. Binary Serialization
Binary serialization refers to the method of transforming an object into a binary format, enabling it to be saved in a file, transmitted across a network, or exchanged between applications. After the object has been serialized into binary, it can subsequently be deserialized, restoring it to its original state.
Features
- Transform an object into a binary representation.
- Optimized for size, though not easily interpretable by humans.
- It necessitates that the object is annotated with the [Serializable] attribute.
- Frequently employed for saving objects to storage or for communication between applications.
Step 1. Create a class for Binary serialization like the one below.EmployeeDetails.cs
[Serializable]
public class EmployeeDetails
{
public string Name { get; set; }
public int Age { get; set; }
public string Address { get; set; }
public string Hobbies { get; set; }
public string EmployeeType { get; set; }
}
Step 2. Serialize the EmployeeDetails.cs class in binary format and save it to the specified location based on your requirements.
public partial class MainWindow : Window
{
EmployeeDetails employee;
// Specify the file path where the Binary file will be saved
string filePath = @"C:\Users\Peter\Desktop\Peter\SerailizedPath\"; // Change this to your desired location
public MainWindow()
{
InitializeComponent();
employee = new EmployeeDetails
{
Name = "Peter",
Age = 30,
Address = "London",
EmployeeType = "Permanent",
Hobbies = "Cricket"
};
}
[Obsolete]
private void BinarySerialization_Click(object sender, RoutedEventArgs e)
{
BinaryFormatter formatter = new BinaryFormatter();
using (FileStream stream = new FileStream(Path.Combine(filePath, "Employee.dat"), FileMode.Create))
{
formatter.Serialize(stream, employee);
}
Debug.WriteLine("Object serialized to employee.dat");
}
}
Step 3. DeSerialize the class EmployeeDetails.cs like below.
[Obsolete]
private void BinaryDESerialization_Click(object sender, RoutedEventArgs e)
{
BinaryFormatter formatter = new BinaryFormatter();
using (FileStream stream = new FileStream(Path.Combine(filePath, "employee.dat"), FileMode.Open))
{
EmployeeDetails person = (EmployeeDetails)formatter.Deserialize(stream);
MessageBox.Show($"Name: {person.Name}, Age: {person.Age}, Address: {person.Address}, EmployeeType: {person.EmployeeType}, Hobbies: {person.Hobbies}");
}
}
4. SOAP Serialization
It refers to the method of transforming an object into the XML format defined by the Simple Object Access Protocol (SOAP), facilitating its transmission across a network, particularly within web services. While SOAP was widely utilized in earlier web service architectures, its prevalence has diminished in recent years, largely due to the increasing adoption of RESTful APIs and JSON serialization.
Features
- Utilizes the SOAP (Simple Object Access Protocol) format.
- Traditionally employed in web services.
Step 1. Add the “SoapFormatter” Nuget package as shown below.
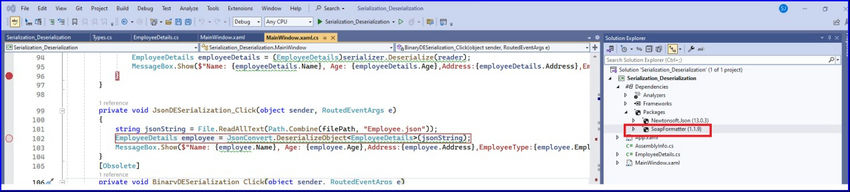
Step 2. Create a class for soap serialization like the one below.
EmployeeDetails.cs
public class EmployeeDetails
{
public string Name { get; set; }
public int Age { get; set; }
public string Address { get; set; }
public string Hobbies { get; set; }
public string EmployeeType { get; set; }
}
Step 3. Serialize the EmployeeDetails.cs class into Soap format and save it to the specified location based on your requirements.
public partial class MainWindow : Window
{
EmployeeDetails employee;
// Specify the file path where the Soap file will be saved
string filePath = @"C:\Users\peter\Desktop\Peter\SerailizedPath\"; // Change this to your desired location
public MainWindow()
{
InitializeComponent();
employee = new EmployeeDetails
{
Name = "Peter",
Age = 30,
Address = "London",
EmployeeType = "Permanent",
Hobbies = "Cricket"
};
}
private void SoapSerialization_Click(object sender, RoutedEventArgs e)
{
SoapFormatter formatter = new SoapFormatter();
using (FileStream stream = new FileStream(Path.Combine(filePath, "Employee.soap"), FileMode.Create))
{
formatter.Serialize(stream, employee);
}
Debug.WriteLine("Object serialized to employee.soap");
}
}
Step 4. DeSerialize the class EmployeeDetails.cs like below.
private void SoapDESerialization_Click(object sender, RoutedEventArgs e)
{
SoapFormatter formatter = new SoapFormatter();
using (FileStream stream = new FileStream(Path.Combine(filePath, "employee.soap"), FileMode.Open))
{
EmployeeDetails person = (EmployeeDetails)formatter.Deserialize(stream);
MessageBox.Show($"Name: {person.Name}, Age: {person.Age}, Address: {person.Address}, EmployeeType: {person.EmployeeType}, Hobbies: {person.Hobbies}");
}
}
Note. The results of all the aforementioned formats can be observed by executing the application as demonstrated below. Various serialization methods cater to distinct use cases influenced by requirements for performance, interoperability, and readability.
Serialization methods
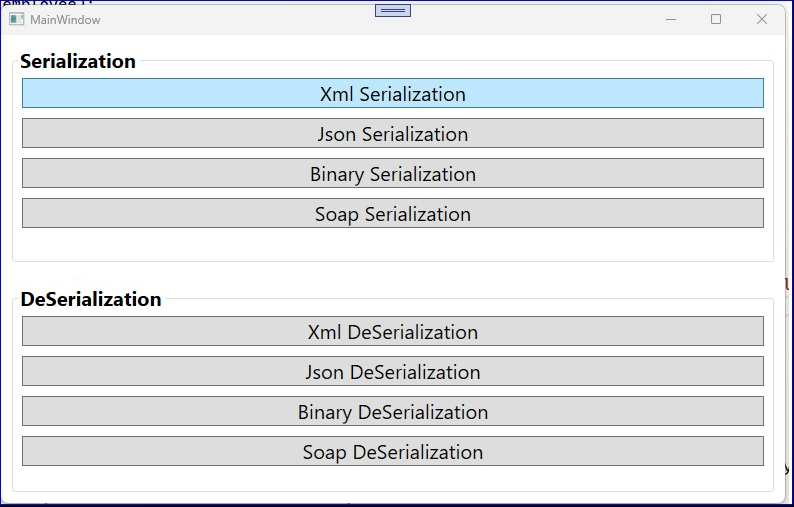
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
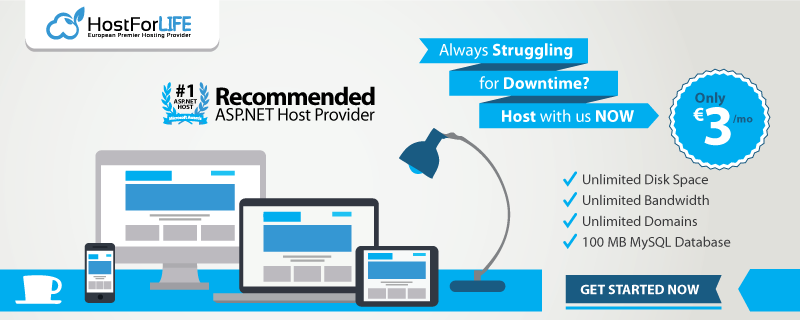