A bitwise enum is an enum type that combines multiple enum values into a singular value using bitwise operations. Each enum value has its own bit indicator, which is represented by a power of 2. By designating these flags, we can represent multiple enum values using bitwise operators to combine or mask them.
How do we define Bitwise Enums?
To define a bitwise enum, the [Flags] attribute must be added to indicate that the enum values can be combined using bitwise operations. Here's an instance:
[Flags] enum DaysOfWeek {
None = 0, Monday = 1, Tuesday = 2, Wednesday = 4, Thursday = 8, Friday = 16,
Saturday = 32, Sunday = 64
};
In the above code, we define a DaysOfWeek enum where each day of the week is assigned a unique power of 2. The None value is assigned 0, indicating no days are selected.
Combining Bitwise Enums
To combine multiple enum values, we use the bitwise OR (|) operator. Here's an example,
DaysOfWeek selectedDays = DaysOfWeek.Monday | DaysOfWeek.Wednesday | DaysOfWeek.Friday;
The above code combines the Monday, Wednesday, and Friday enum values using the bitwise OR operator.
Checking for Enum Values
To check if a specific enum value is set in a bitwise enum, we use the bitwise AND (&) operator. Here's an example:
Console.WriteLine("selected Days", selectedDays);
if ((selectedDays & DaysOfWeek.Monday) != 0) {
Console.WriteLine("Monday is selected.");
}
selectedDays ^= DaysOfWeek.Wednesday;
In the above code, we check if the Monday value is set in the selectedDays variable using the bitwise AND operator.
Removing Enum Values
To remove a specific enum value from a bitwise enum, we use the bitwise XOR (^) operator. Here's an example:
selectedDays ^= DaysOfWeek.Wednesday;
Console.WriteLine(selectedDays);
In the above code, we remove the Wednesday value from the selectedDays variable using the bitwise XOR operator.
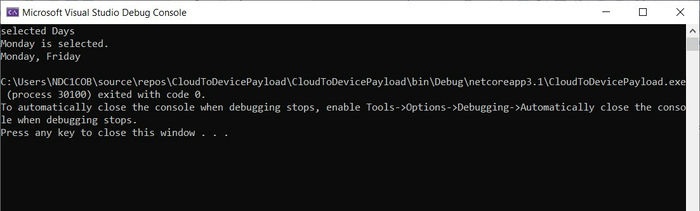
In C#, bitwise enums offer a potent method for effectively encoding and modifying flag-based enumerations. We can mix, check, and remove particular enum values within a compact representation by giving them distinctive bit flags and using bitwise operations. Bitwise enums provide ease and flexibility for expressing flag-based situations such as days of the week, permissions, or other flag-based circumstances. If you have a firm grasp of bitwise enums, you can use this functionality in your C# projects to deal with challenging flag-based scenarios.
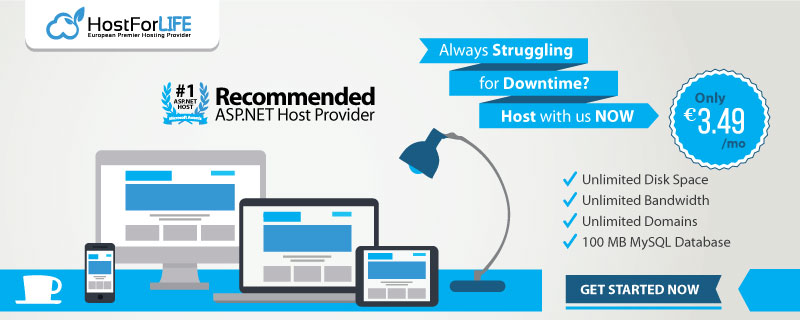