
June 13, 2023 10:22 by
Peter
In the. NETCore, a HostedService, is a type of class that embodies a background task or service running asynchronously within an application. Its purpose is to initiate when the application starts and conclude when the application ceases. HostedService is an integral component of the generic host in .NET Core, enabling various functionalities like executing background processes, managing job schedules, and monitoring system resources.
The tools which I have leveraged for this tutorial are below.
VS 2022 Community Edition (64-bit)
.Net 7.0
Console App
The entire source code can be downloaded from GitHub.
Here's an example of creating a HostedService in .NET Core.
using System;
using System.Threading;
using System.Threading.Tasks;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
public class MyHostedService : IHostedService, IDisposable
{
private readonly ILogger<MyHostedService> _logger;
private Timer _timer;
public MyHostedService(ILogger<MyHostedService> logger)
{
_logger = logger;
}
public Task StartAsync(CancellationToken cancellationToken)
{
_logger.LogInformation("MyHostedService is starting.");
_timer = new Timer(DoWork, null, TimeSpan.Zero, TimeSpan.FromSeconds(5));
return Task.CompletedTask;
}
private void DoWork(object state)
{
_logger.LogInformation("Doing some work...");
// Perform your background processing or task here
}
public Task StopAsync(CancellationToken cancellationToken)
{
_logger.LogInformation("MyHostedService is stopping.");
_timer?.Change(Timeout.Infinite, 0);
return Task.CompletedTask;
}
public void Dispose()
{
_timer?.Dispose();
}
}
In this instance, the class MyHostedService is implemented with the IHostedService interface, which necessitates the implementation of the StartAsync and StopAsync methods. The ILogger is injected into the service's constructor for the purpose of logging.
The StartAsync method is invoked upon application startup. Within this method, you can initialize and commence any background tasks or processing. In this example, a Timer is instantiated to simulate a recurring task occurring every 5 seconds.
The StopAsync method is called when the application is shut down. Its purpose is to gracefully halt any ongoing work and perform resource cleanup. In the provided example, the Timer is stopped.
The Dispose method is implemented to properly release any resources utilized by the service.
To utilize the MyHostedService, it is necessary to register it within the dependency injection container of your application. Here's an illustration of how to configure and execute the HostedService in a console application.
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
var host = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
services.AddHostedService<MyHostedService>();
})
.ConfigureLogging(logging =>
{
logging.ClearProviders();
logging.AddConsole();
})
.Build();
await host.RunAsync();
In this scenario, the AddHostedService method is employed to enlist MyHostedService as a hosted service within the dependency injection container.
Upon executing the console application, MyHostedService will initiate and operate in the background until the application is halted.
Conclusion
Please take note that this example relies on Microsoft.Extensions.Hosting and Microsoft.Extensions.Logging namespaces, which are frequently utilized in .NET Core applications. Ensure you include the necessary NuGet packages (Microsoft.Extensions.Hosting and Microsoft.Extensions.Logging.Console) in your project.
This sample serves as a fundamental illustration of a HostedService in .NET Core. It can be customized and expanded to cater to your specific requirements.
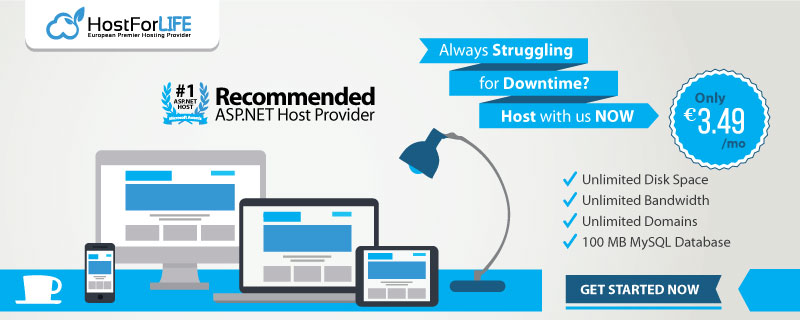