The Entity Framework (EF) Core is a lightweight, extendable, open-source, cross-platform version of the well-known Entity Framework data access technology. We'll look at the Code First technique in EF Core using a.NET 7 API in this article. This process begins with the definition of your model classes; EF Core then generates the database and its structure based on these models.
Why do we require EF Core?
Microsoft's EF Core is an open-source lightweight ORM that provides a mechanism to connect with relational databases using programming concepts. Here are some of the reasons why it is advantageous.
- Object-Relational Mapping: EF Core connects relational databases to object-oriented programming. It lowers the amount of boilerplate code required to convert database tables and columns into object properties. This is handled automatically by EF Core.
- Language Integrated Query (LINQ): LINQ is a strong querying language included with.NET. It enables developers to write database queries in C# syntax. LINQ converts our C# queries into efficient database queries.
- Testability is promoted by the design of EF Core, which allows developers to fake or alter the database context during unit testing. This allows developers to build isolated tests that do not rely on a physical database, making testing more efficient and reliable.
Let's begin
We will create a new project.
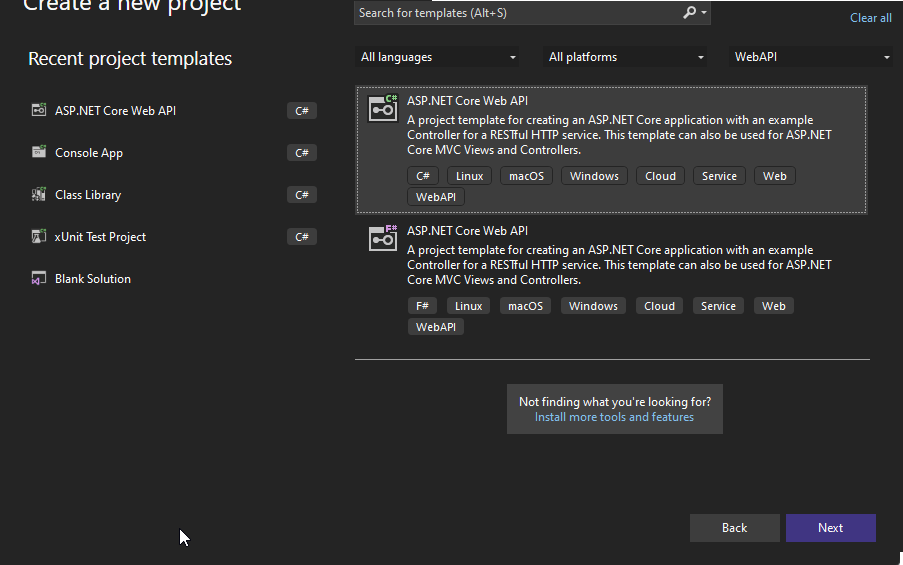
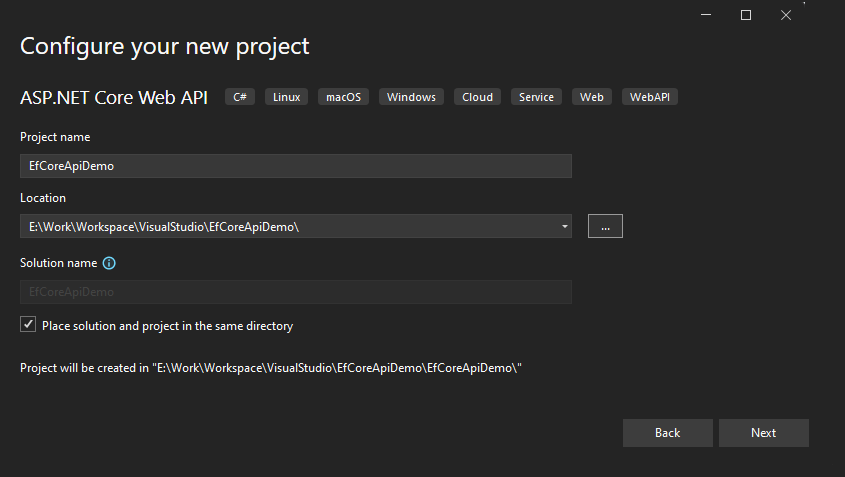
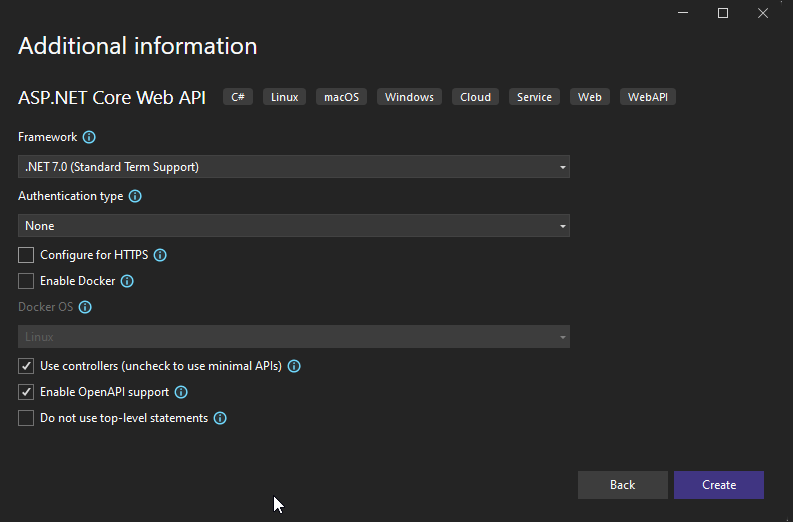
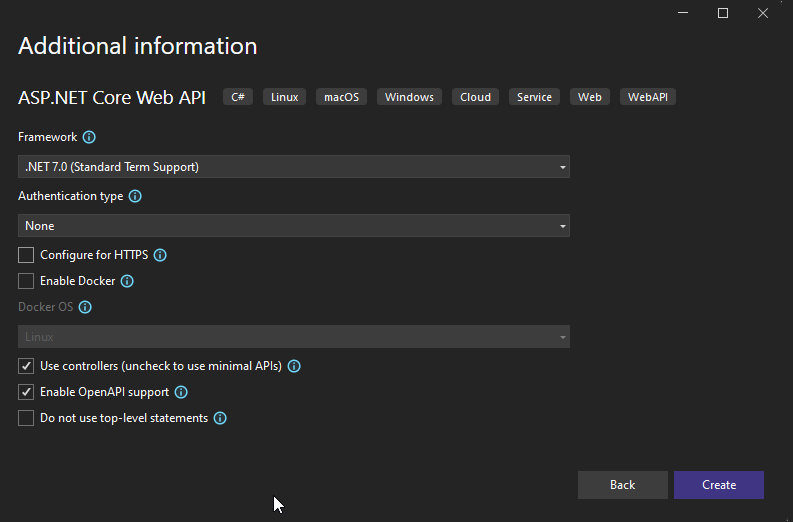
After project creation, we will have a structure like this.
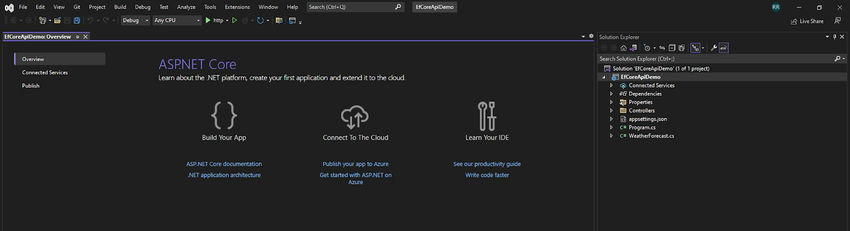
Next, we will install the required nuget packages.
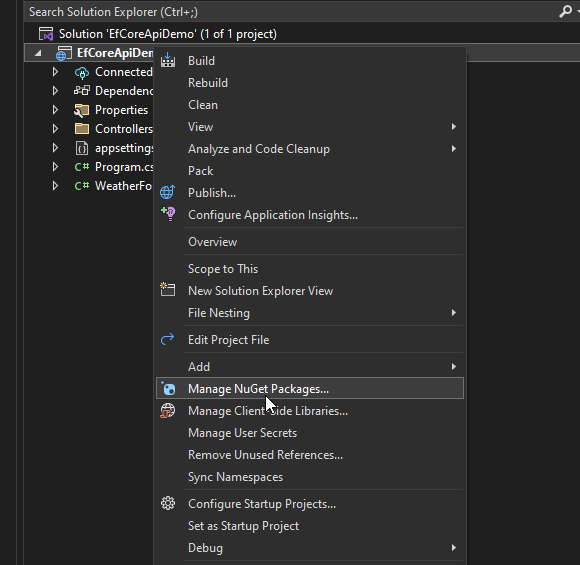
Install the following packages,
Microsoft.EntityFrameworkCore
Microsoft.EntityFrameworkCore.Design
Microsoft.EntityFrameworkCore.SqlServer
Now we will create a folder called 'Models' in the solution, and inside it, we will add another folder called Entities which will have entity classes from which our database will be created.
We will create a class "Department.cs" with the following properties.
public class Department
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public bool IsActive { get; set; }
public DateTime CreatedDate { get; set; }
public DateTime UpdatedDate { get; set; }
}
Now we will create a class called "Employee.cs".
public class Employee
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public int DepartmentId { get; set; }
public bool IsActive { get; set; }
public DateTime CreatedDate { get; set; }
public DateTime UpdatedDate { get; set; }
}
Now we will create an "EmployeeDbContext.cs".
public class EmployeeDbContext : DbContext
{
public EmployeeDbContext(DbContextOptions<EmployeeDbContext> options) : base(options)
{
}
public DbSet<Department> Departments { get; set; }
public DbSet<Employee> Employees { get; set; }
}
As we can see, this EmployeeDbContext class extends from the DbContext class that comes in the "Microsoft.EntityFrameworkCore" framework, and then we have the DbSets for the Departments and Employees as per the entities we created.
Now we will configure the DB context in the "Program.cs".
We will add the following code.
builder.Services.AddDbContext<EmployeeDbContext>(options =>
{
options.UseSqlServer("Server=RAVI;Database=EFCoreCodeFirstDB;Trusted_Connection=True;MultipleActiveResultSets=true;TrustServerCertificate=True;");
});
After that, we will run the migrations commands that will help us maintain the DB state.
Run the following command in the solution directory.
“dotnet ef migrations add InitialCreate”.
After running it, the output will look something like this.

In the solution explorer, you will see the following folder.
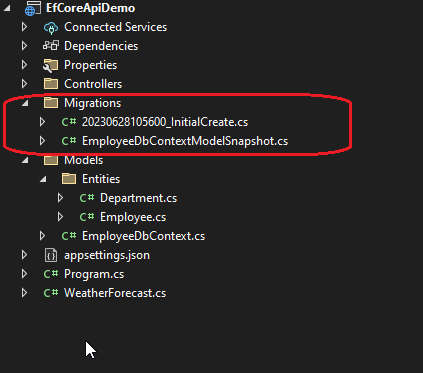
Note. If the above command throws the error, please run the following command first.
“dotnet tool install --global dotnet-ef”
After this, we will run the following command.
“dotnet ef database update”
You should see the following output.
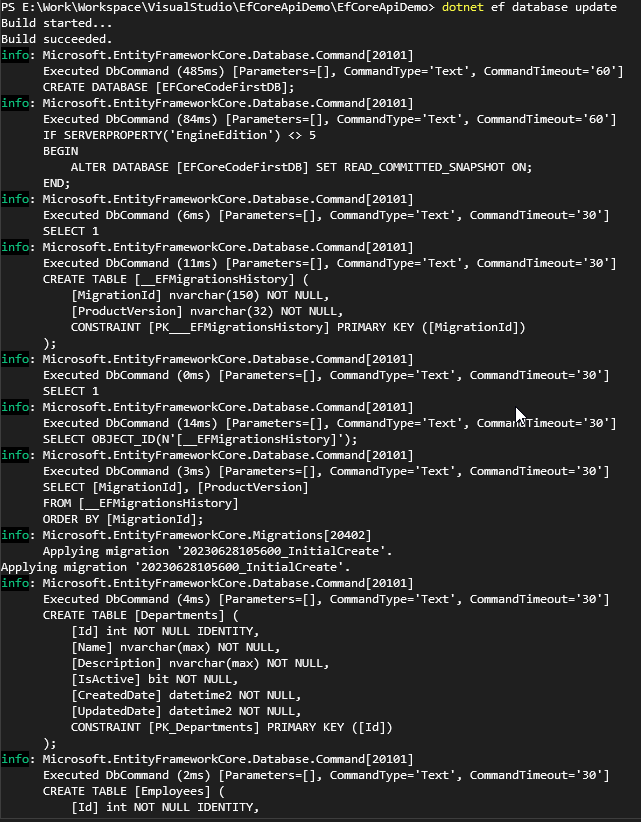
After this, you can see your SqlServer to see that your database has been created along with your tables.
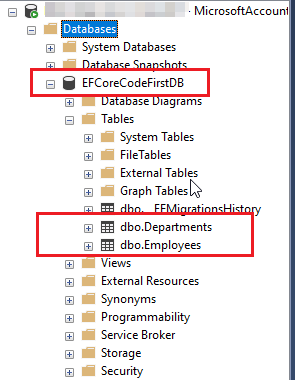
Now we will add a controller called "DepartmentController.cs".
[Route("api/[controller]")]
[ApiController]
public class DepartmentController: ControllerBase {
private readonly EmployeeDbContext _dbContext;
public DepartmentController(EmployeeDbContext dbContext) {
_dbContext = dbContext;
}
[HttpGet]
public IActionResult Get() {
var departments = _dbContext.Departments.ToList();
return Ok(departments);
}
[HttpPost]
public IActionResult Post([FromBody] Department department) {
_dbContext.Departments.Add(department);
_dbContext.SaveChanges();
return Ok();
}
[HttpPut]
public IActionResult Put([FromBody] Department department) {
var dept = _dbContext.Departments.FirstOrDefault(d => d.Id == department.Id);
if (dept == null)
return NotFound();
dept.Name = department.Name;
dept.Description = department.Description;
dept.UpdatedDate = DateTime.Now;
_dbContext.SaveChanges();
return Ok(dept);
}
}
In our DepartmentController.cs, we have three REST endpoints; one is HttpGet which will get us the list of all departments. The second endpoint is HTTP POST which will save the departments in the DB, and the last one is HTTPPUT which will update the department in the DB.
Similarly, we will have an employee controller as well, which will save, update and get employees.
[Route("api/[controller]")]
[ApiController]
public class EmployeeController: ControllerBase {
private readonly EmployeeDbContext _dbContext;
public EmployeeController(EmployeeDbContext dbContext) {
_dbContext = dbContext;
}
[HttpGet]
public IActionResult Get() {
var employees = _dbContext.Employees.ToList();
return Ok(employees);
}
[HttpPost]
public IActionResult Add([FromBody] Employee employee) {
_dbContext.Employees.Add(employee);
_dbContext.SaveChanges();
return Ok();
}
[HttpPut]
public IActionResult Update([FromBody] Employee employee) {
var emp = _dbContext.Employees.FirstOrDefault(d => d.Id == employee.Id);
if (emp == null)
return NotFound();
emp.FirstName = employee.FirstName;
emp.LastName = employee.LastName;
emp.DepartmentId = employee.DepartmentId;
emp.UpdatedDate = DateTime.Now;
_dbContext.SaveChanges();
return Ok(emp);
}
}
Now let's run our API, and then we will hit the POST endpoint of the department's controller.
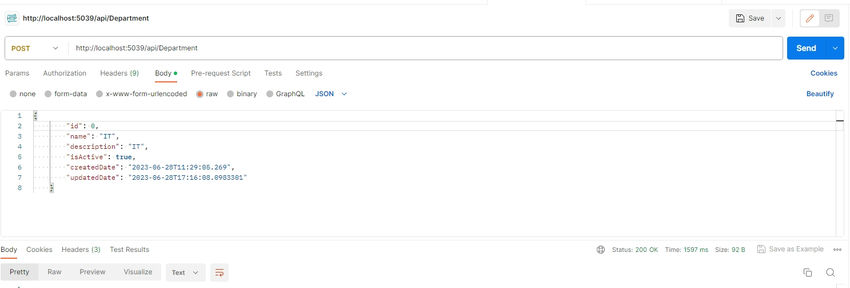
Now we will try to get all the departments by hitting the Get endpoint of departments.
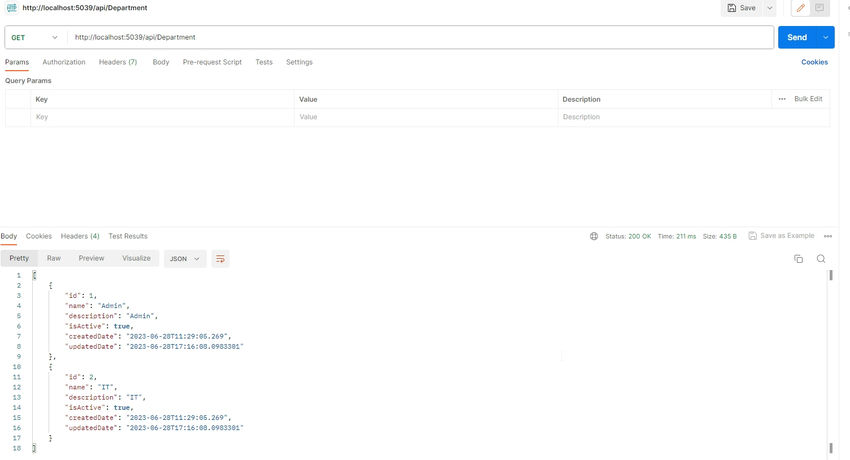
Now we will create a new employee using the Post endpoint of the employee controller.
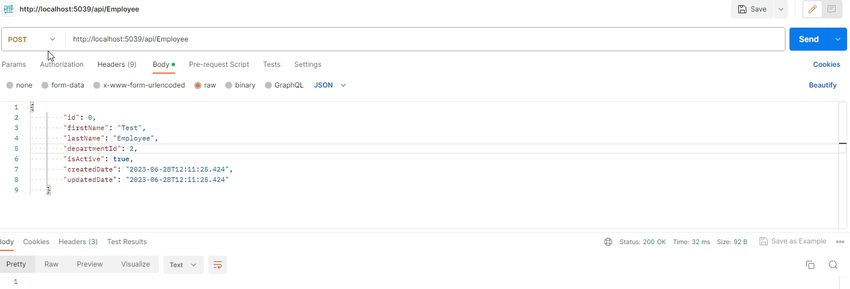
Now we will hit the Get endpoint of the employee controller.
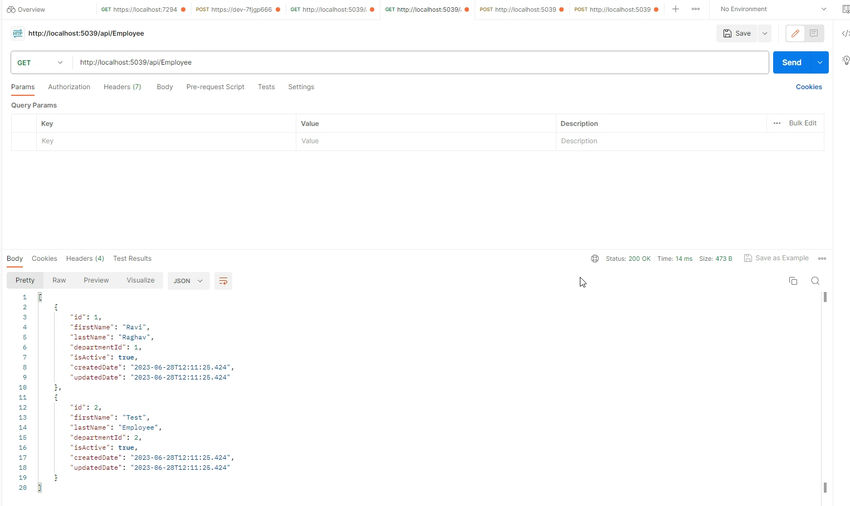
Now we can verify the same values from the database as well.
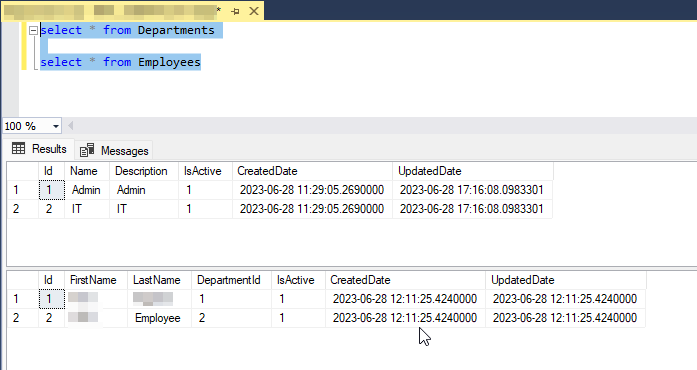
As we can verify, our values are stored in the database as well.
We have seen how to create a .NET 7 API and use EF Core to interact with the relational database to store and get the data.
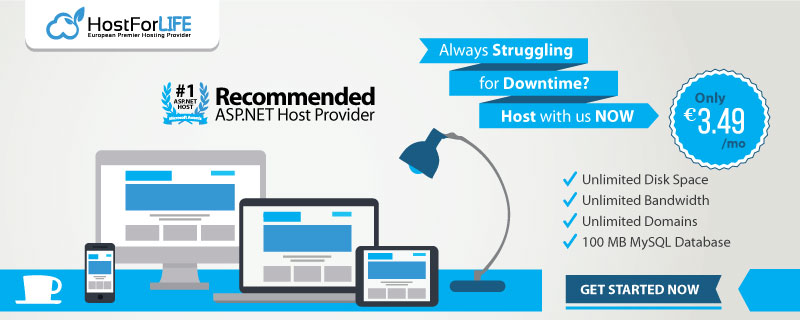