In this article, we will be sending push notifications to Android devices via C# Dot Net/.Net. Using Firebase Cloud Messaging API HTTP V1.
This article will guide using of FCM HTTP V1 over Legacy FCM API.
Legacy FCM API :
https://fcm.googleapis.com/fcm/send
HTTP
Latest FCM Http V1:
https://fcm.googleapis.com/v1/projects/myproject-b5ae1/messages:send
HTTP
The latest FCM Http V1 provides better security, Support for the new Client Platform version.
Section 1. Create/ Login in Firebase Console Account.
URL: https://console.firebase.google.com/
After creating of account/Login, Click on Add Project.
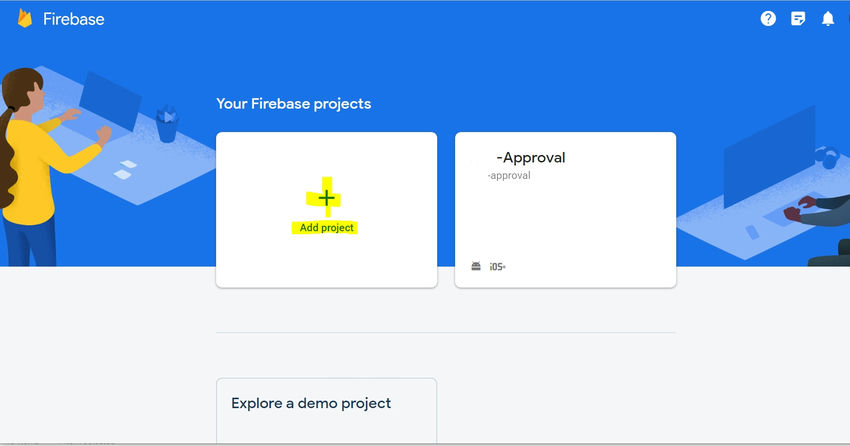
Section 2. Add Firebase to your Android project
Section 3. Go to Project Settings
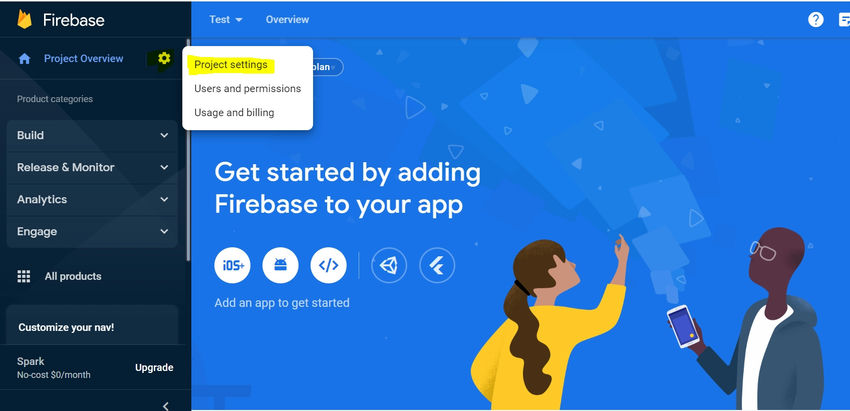
Section 4. Enable FCM HTTP V1
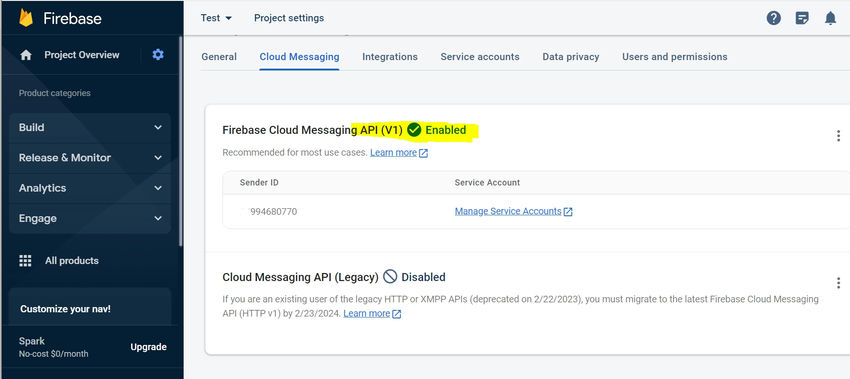
Section 5. Create a .Net Web API project; write this code to generate a Bearer token for calling FCM HTTP V1 API.

Code
using System.IO;
using Google.Apis.Auth.OAuth2;
using System.Net.Http.Headers;
using System.Text;
using System.Web;
using System.Web.Configuration;
using System.Net.Http;
using System.Web.Http;
//----------Generating Bearer token for FCM---------------
string fileName = System.Web.Hosting.HostingEnvironment.MapPath("~/***-approval-firebase-adminsdk-gui00-761039f087.json"); //Download from Firebase Console ServiceAccount
string scopes = "https://www.googleapis.com/auth/firebase.messaging";
var bearertoken = ""; // Bearer Token in this variable
using(var stream = new FileStream(fileName, FileMode.Open, FileAccess.Read)) {
bearertoken = GoogleCredential
.FromStream(stream) // Loads key file
.CreateScoped(scopes) // Gathers scopes requested
.UnderlyingCredential // Gets the credentials
.GetAccessTokenForRequestAsync().Result; // Gets the Access Token
}
Section 6. Follow this Step to Download the Json file from the Firebase Console Account (Project Settings -> Service Account -> Generate New Private Key) & Place the Json File in your Project directory.
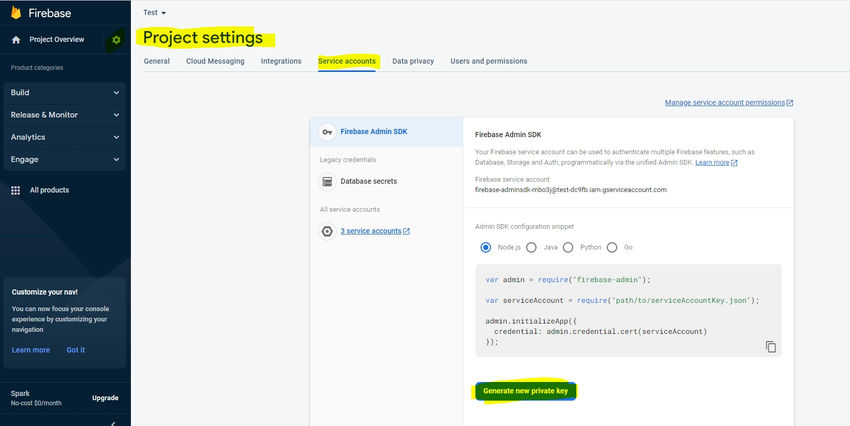
Section 7. Place the downloaded JSON File in your .net Project directory.
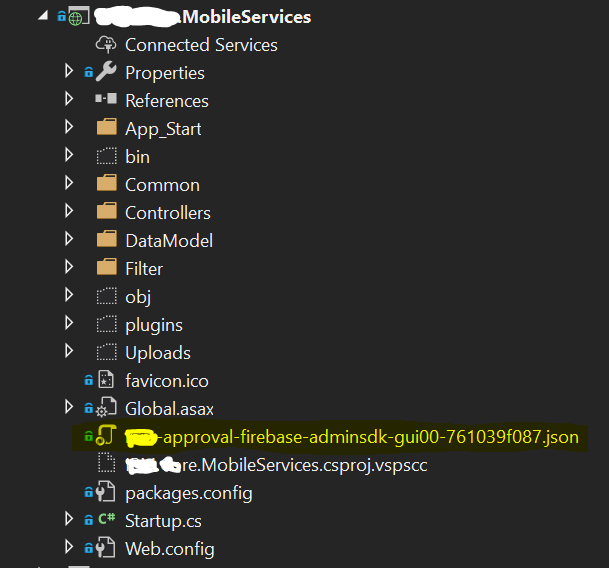
Section 8. Install Google.Api.Auth from Nuget Package Manager
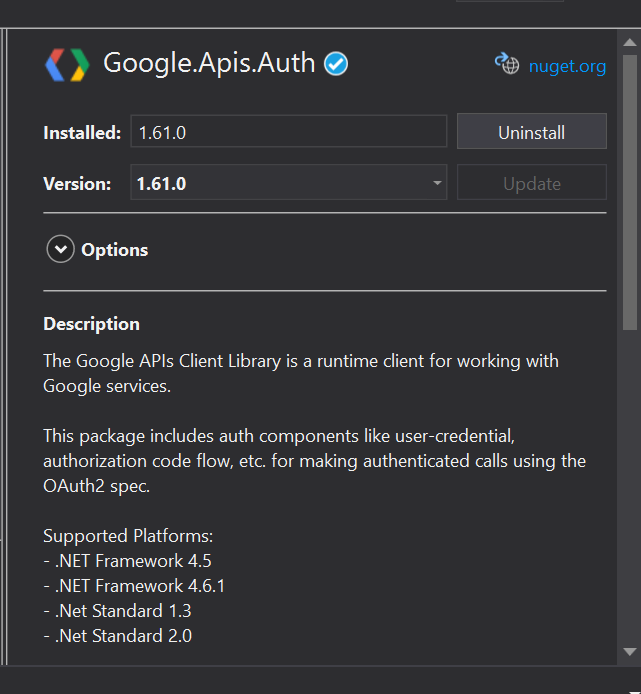
Section 9. Use the Authorization token generated above via c# code to call FCM HTTP V1 API; get the FCM Token of Android from an Android device. Test using Postman; a push notification will be sent to the Android device.
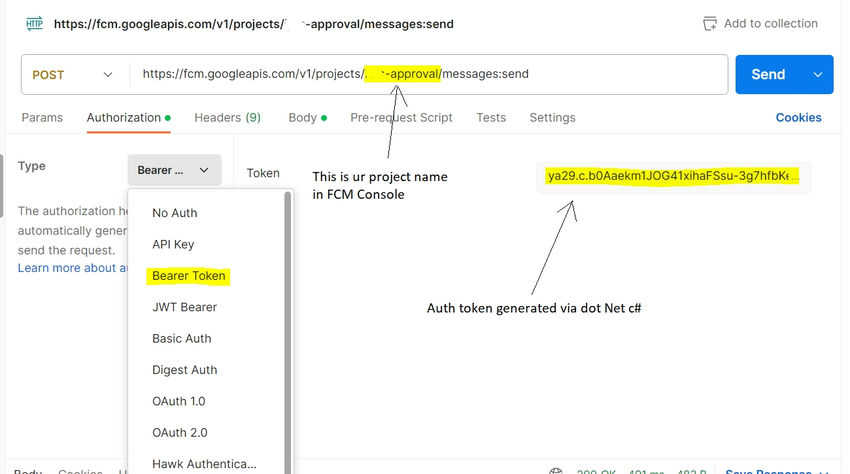
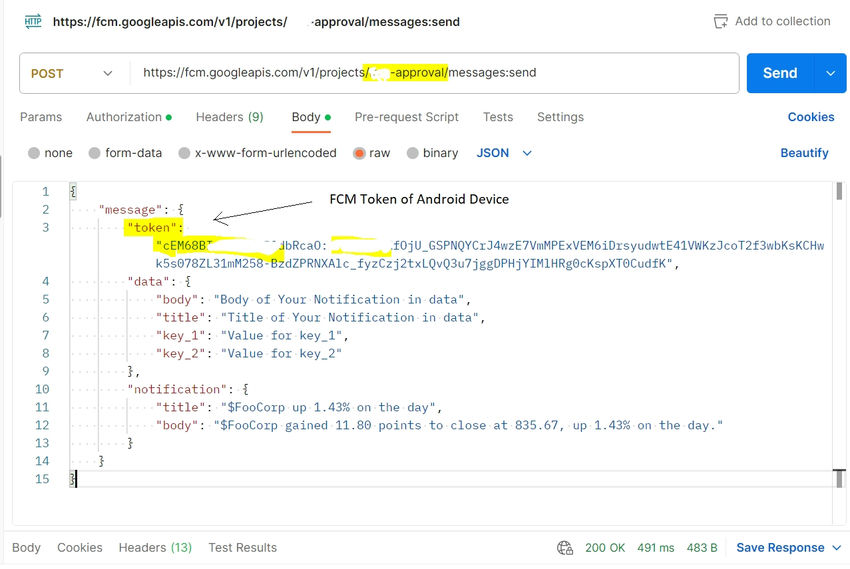
Section 10. On successful completion of the above steps, Implement Further, Create this Model Classes for storing Request & Response data.
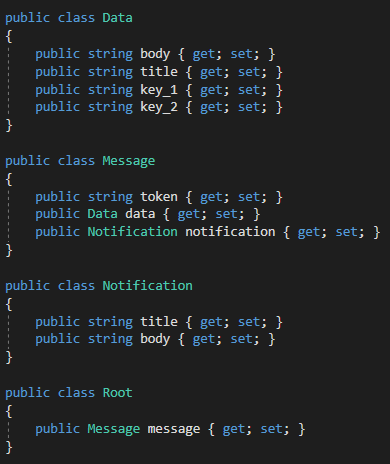
Section 11. Call the FCM HTTP V1 API using the Authorization token generated & get the FCM id / Token from the Android device.
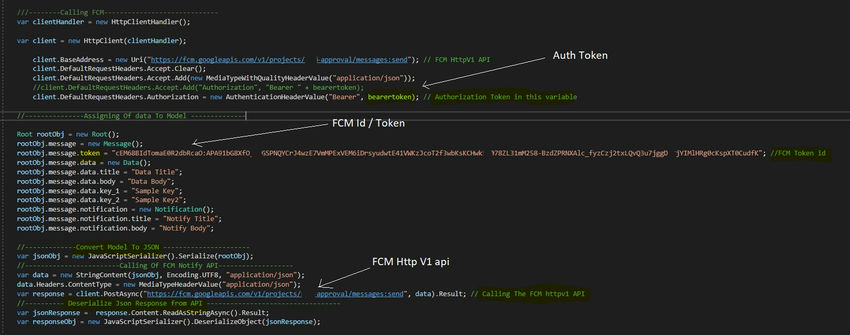
Section 12. Entire Code
#region FCM Auth & Send Notification To Mobile //notify FCM Code
public class Data {
public string body {
get;
set;
}
public string title {
get;
set;
}
public string key_1 {
get;
set;
}
public string key_2 {
get;
set;
}
}
public class Message {
public string token {
get;
set;
}
public Data data {
get;
set;
}
public Notification notification {
get;
set;
}
}
public class Notification {
public string title {
get;
set;
}
public string body {
get;
set;
}
}
public class Root {
public Message message {
get;
set;
}
}
public void GenerateFCM_Auth_SendNotifcn()
{
//----------Generating Bearer token for FCM---------------
string fileName = System.Web.Hosting.HostingEnvironment.MapPath("~/***-approval-firebase-adminsdk-gui00-761039f087.json"); //Download from Firebase Console ServiceAccount
string scopes = "https://www.googleapis.com/auth/firebase.messaging";
var bearertoken = ""; // Bearer Token in this variable
using(var stream = new FileStream(fileName, FileMode.Open, FileAccess.Read))
{
bearertoken = GoogleCredential
.FromStream(stream) // Loads key file
.CreateScoped(scopes) // Gathers scopes requested
.UnderlyingCredential // Gets the credentials
.GetAccessTokenForRequestAsync().Result; // Gets the Access Token
}
///--------Calling FCM-----------------------------
var clientHandler = new HttpClientHandler();
var client = new HttpClient(clientHandler);
client.BaseAddress = new Uri("https://fcm.googleapis.com/v1/projects/***-approval/messages:send"); // FCM HttpV1 API
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
//client.DefaultRequestHeaders.Accept.Add("Authorization", "Bearer " + bearertoken);
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", bearertoken); // Authorization Token in this variable
//---------------Assigning Of data To Model --------------
Root rootObj = new Root();
rootObj.message = new Message();
rootObj.message.token = "cEM68BIdTomaE0R2dbaO:APA91bG8XfOjU_GSPNQYCrJ4wzE7VmMPEsyudwtE41VWKzJcoT2f3wbKsKCHwk5s078ZL31mM258-BzdZPRNXAlc_fyzCzj2txLQvQ3u7jggDPHjYIMlHRgspXT0CudfK"; //FCM Token id
rootObj.message.data = new Data();
rootObj.message.data.title = "Data Title";
rootObj.message.data.body = "Data Body";
rootObj.message.data.key_1 = "Sample Key";
rootObj.message.data.key_2 = "Sample Key2";
rootObj.message.notification = new Notification();
rootObj.message.notification.title = "Notify Title";
rootObj.message.notification.body = "Notify Body";
//-------------Convert Model To JSON ----------------------
var jsonObj = new JavaScriptSerializer().Serialize(rootObj);
//------------------------Calling Of FCM Notify API-------------------
var data = new StringContent(jsonObj, Encoding.UTF8, "application/json");
data.Headers.ContentType = new MediaTypeHeaderValue("application/json");
var response = client.PostAsync("https://fcm.googleapis.com/v1/projects/**-approval/messages:send", data).Result; // Calling The FCM httpv1 API
//---------- Deserialize Json Response from API ----------------------------------
var jsonResponse = response.Content.ReadAsStringAsync().Result;
var responseObj = new JavaScriptSerializer().DeserializeObject(jsonResponse);
}
#endregion
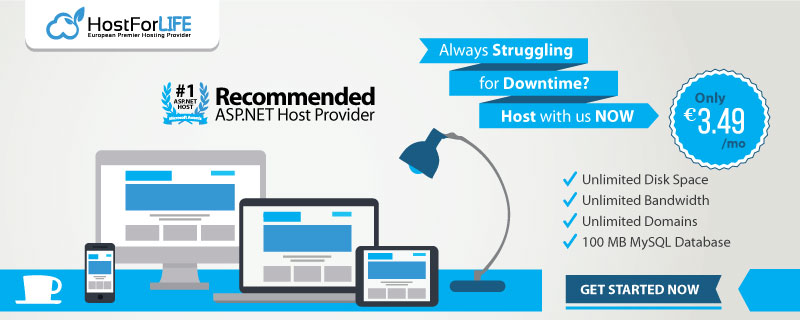