
January 31, 2024 07:55 by
Peter
In modern web development, making HTTP requests is a fundamental aspect of building robust and dynamic applications. In the .NET Core ecosystem, the HttpClient class has long been the go-to choice for sending HTTP requests. However, as applications grow in complexity, managing instances of HttpClient can become a challenge. This is where the HttpClientFactory in .NET Core comes to the rescue, providing a more efficient and scalable solution for handling HTTP requests.
HttpClient: What is it?
Developers can send and receive HTTP requests and responses by using the HttpClient class in the System.Net.Http namespace. Because of its portability and ability to establish and manage HTTP connections, it can be used in situations where several requests are sent to the same endpoint.
This is a simple example of sending a GET request using HttpClient.
using System;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
using (HttpClient client = new HttpClient())
{
string url = "https://api.example.com/data";
HttpResponseMessage response = await client.GetAsync(url);
if (response.IsSuccessStatusCode)
{
string result = await response.Content.ReadAsStringAsync();
Console.WriteLine(result);
}
}
}
}
Even if this method functions, it's not the most effective for handling several requests at once. It is typically not advised to create a new instance of HttpClient for each request as this can cause problems such as socket exhaustion.
HttpClientFactory: What is it?
The HttpClientFactory is part of the Microsoft.Extensions.In order to overcome the problems of manually managing HttpClient instances, the http package was established. It offers a more organized and scalable method for setting up, configuring, and administering HttpClient instances.
Principal Advantages of Using HttpClientFactory
- Lifetime Management: It manages the HttpClient instances' lifecycle, reusing existing connections to avoid problems like socket exhaustion.
- Configuration: HttpClientFactory makes it easier to manage many API endpoints with distinct settings by enabling you to configure HttpClient objects through named clients.
- Dependency Injection Integration: It makes injecting HttpClient objects into your services simple by integrating smoothly with the ASP.NET Core dependency injection framework.
HttpClientFactory use
Let's begin by going over how to use HttpClientFactory in a.NET Core application step-by-step.
1. Set up the required NuGet package.
dotnet add package Microsoft.Extensions.Http
2. Configure HttpClient in Startup.cs
services.AddHttpClient("MyApiClient", client =>
{
client.BaseAddress = new Uri("https://api.example.com/");
// Additional configuration options can be set here
});
3. Inject HttpClient into your service
public class MyService
{
private readonly HttpClient _httpClient;
public MyService(HttpClient httpClient)
{
_httpClient = httpClient;
}
// Use _httpClient to make requests
}
4. Use IHttpClientFactory for manual creation (optional)
If you need more control, you can inject IHttpClientFactory and create HttpClient instances manually.
public class MyService
{
private readonly IHttpClientFactory _httpClientFactory;
public MyService(IHttpClientFactory httpClientFactory)
{
_httpClientFactory = httpClientFactory;
}
public async Task MakeRequest()
{
HttpClient client = _httpClientFactory.CreateClient("MyApiClient");
HttpResponseMessage response = await client.GetAsync("/data");
// Handle the response
}
}
In order to effectively use HttpClient and fully utilize HttpClientFactory, one must grasp HTTP requests in.NET Core. By implementing best practices, developers may create scalable and performant apps that communicate with external APIs with ease. Examples of these practices include controlling the lifetime of HttpClient objects and utilizing dependency injection. Developers may design dependable, high-performance solutions for managing HTTP requests in their.NET Core applications with the help of HttpClient and HttpClientFactory.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
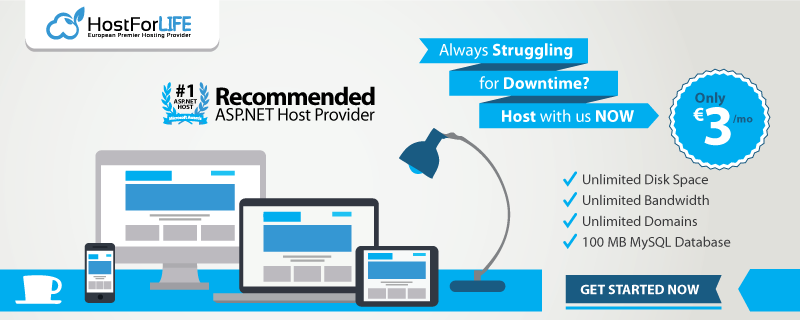