
January 26, 2024 05:37 by
Peter
Overview of the Entity Framework
A strong and adaptable Object-Relational Mapping (ORM) framework that makes database interactions in.NET applications easier is called Entity Framework (EF). We'll explore several Entity Framework techniques in this extensive tutorial, with examples to help even.NET novices understand the ideas.
Entity Framework removes the requirement for developers to write raw SQL queries by enabling them to communicate with databases using.NET objects. It supports various strategies, each having benefits and applications of its own.
1. A database-first strategy
The Database-First method entails building the database first, then using it to generate the model.
Actions
- Build Database: Using SQL Server Management Studio or another database tool, design and build the database.
- Create Model: To create the model from the current database, use the Entity Data Model Wizard.
- Employ Created Classes For database activities, make use of the automatically produced classes.
// Auto-generated class representing a table in the database
public partial class Product
{
public int ProductID { get; set; }
public string ProductName { get; set; }
// Other properties
}
// Usage in code
using (var context = new YourDbContext())
{
var products = context.Products.ToList();
// Perform operations on products
}
2. Code-First Approach
The Code-First method starts with the creation of the domain classes, from which the database is then generated.
Actions
- Establish domain classes that represent entities by using define classes.
- Establish Relationships: To establish relationships between entities, use Data Annotations or Fluent API.
- Create Database: Based on the model, create and update the database using migrations.
public class Product
{
public int ProductID { get; set; }
public string ProductName { get; set; }
// Other properties
}
public class YourDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
// Other DbSets
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
// Fluent API configuration for relationships
}
}
3. Model-First Method
Using the Entity Data Model Designer, the Entity Data Model (EDM) is created, and the database is subsequently generated from it via the Model-First approach.
Actions
- To develop an Entity Data Model, use the Entity Data Model Designer.
- Generate Database: Select the Generate Database from Model option to generate the database from the EDM.
As an illustration
With Entity Data Model Designer, the model-first approach frequently incorporates visual design, which makes it less code-oriented.
4. Context of Databases and LINQ Queries
Whichever strategy is used, after the model is created developers use a database context to communicate with the database via LINQ queries.
using (var context = new YourDbContext())
{
var products = from p in context.Products
where p.ProductName.Contains("Shoes")
select p;
// Perform operations on products
}
Comprehending the various methodologies in Entity Framework enables.NET developers to select the most appropriate technique for their project needs. Entity Framework offers flexibility and abstraction for effective database interactions in.NET applications, regardless of your preference for building databases first, writing classes, or using visual modeling.
Have fun with coding!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
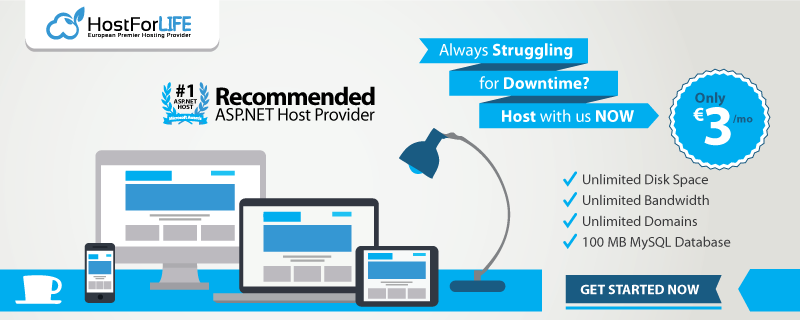