Pattern matching is a functional programming feature that is already present in several prominent languages, including Scala, Rust, Python, Haskell, Prolog, and others. It is used to test expressions for certain circumstances while also testing the kinds.
It enables you to check if a value matches a specific pattern and then execute code based on that match in an efficient and simple manner. This capability is very handy when working with complex data structures like collections and when simplifying conditional expressions.
Pattern Matching was introduced in C# 7.
Benefits- Patterns Matching
- Type-Testing
- Nullable-type checking
- Typecasting and assignment
- High readability
- Concise syntax Less convoluted code.
Usages
Pattern matching can be used in 2 places.
'is' expressions
'switch' expressions
Patterns Matching Types through C# Versions
C# 7
Type Pattern
Declaration Pattern
Constant Pattern
Null Pattern
Var Pattern
C# 8
Property Pattern
Discard Pattern
Positional Pattern
Discard Pattern
C# 9
Type Pattern
Relative Pattern
Logical Pattern (Combinators)
Negated Null Constant Pattern
Parenthesized Pattern
C# 10
Extended Property Pattern
C# 11
List Pattern
Type Pattern
A type pattern in C# allows you to check whether an object is of a specific type and, if it is, cast it to that type while declaring a new variable.
using System;
public class Program
{
public static void Main()
{
object someObject = "Hello, World!";
if (someObject is string stringValue)
{
// stringValue is now a strongly-typed variable of type string
Console.WriteLine($"Length of the string: {stringValue.Length}");
}
else
{
Console.WriteLine("someObject is not a string.");
}
}
}
Pattern of Declaration
In C#, a declaration pattern is one that not only examines whether an expression matches a given pattern but also declares a new variable with the matched value. This pattern is frequently used in conjunction with is expressions to accomplish pattern matching as well as variable declaration in a single action.
C# 7.0 added declaration patterns, which provided a handy approach to simplify code.
using System;
public class Program
{
public static void Main()
{
object someObject = 42;
if (someObject is int intValue)
{
// intValue is now a strongly-typed variable of type int
Console.WriteLine($"The value is an integer: {intValue}");
}
else
{
Console.WriteLine("The value is not an integer.");
}
}
}
Recurring pattern
Testing against a constant value, which can be an int, float, char, string, bool, enum, const-declared field, or null.
It's frequently used in switch statements and pattern-matching contexts to conduct different actions based on an expression's value.
using System;
public class Program
{
public static void Main()
{
object someObject = 42;
if (someObject is int intValue)
{
// intValue is now a strongly-typed variable of type int
Console.WriteLine($"The value is an integer: {intValue}");
}
else
{
Console.WriteLine("The value is not an integer.");
}
}
}
Null Pattern
Check if a reference or nullable type is null.
It is particularly useful for safely handling null values and reducing null reference exceptions.
Null pattern matching was introduced in C# 9.0 as part of the language's pattern-matching enhancements.
Var Pattern
Similar to the type pattern, the var pattern matches an expression and checks for null, as well as assigns a value to the variable.
The var type is declared based on the matched expression’s compile-time type.
using System;
public class Program
{
public static void Main()
{
object someObject = (42, "Hello, Peter!");
if (someObject is var (number, message) && number is int && message is string)
{
Console.WriteLine($"Number: {number}, Message: {message}");
}
else
{
Console.WriteLine("Pattern match failed.");
}
}
}
Property Pattern
Property pattern matching in C# is a feature that allows you to match objects based on the values of their properties or fields.
It simplifies code by enabling you to specify patterns that involve property values directly in pattern-matching expressions.
Property pattern matching was introduced in C# 8.0 and is a powerful way to work with complex data structures.
using System;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Program
{
public static void Main()
{
Person person = new Person { Name = "Alice", Age = 30 };
if (person is { Name: "Alice", Age: 30 })
{
Console.WriteLine("It's Alice, age 30!");
}
else
{
Console.WriteLine("It's someone else.");
}
}
}
Discard Pattern
discard pattern in C# allows you to match a pattern but discard the matched value.
It's represented by the underscore (_) and is especially useful when you want to indicate that you're not interested in the value that matches a pattern, which can help improve code clarity and readability. Discard patterns were introduced in C# 7.0.
using System;
public class Program
{
public static void Main()
{
object someObject = "Hello, World!";
if (someObject is string _)
{
Console.WriteLine("The object is a string, but we're not interested in its value.");
}
else
{
Console.WriteLine("The object is not a string.");
}
}
}
Positional Pattern
Positional patterns in C# allow you to match objects based on the values of their elements in a specific order, making it easier to work with complex data structures like arrays, tuples, or user-defined types.
This feature was introduced in C# 8.0 and provides a concise way to match objects by their elements' positions.
using System;
public class Program
{
public static void Main()
{
Point point = new Point(3, 4);
string location = DescribePoint(point);
Console.WriteLine(location);
}
public static string DescribePoint(Point point)
{
return point switch
{
(0, 0) => "The point is at the origin (0, 0).",
(var x, var y) when x == y => $"The point is on the diagonal at ({x}, {y}).",
(var x, var y) => $"The point is at ({x}, {y}).",
_ => "Unknown location."
};
}
}
public record Point(int X, int Y);
Tuple Pattern
Tuple patterns in C# allow you to match objects against specific tuple structures. This feature simplifies the process of deconstructing and matching tuples in pattern-matching expressions.
Tuple patterns were introduced in C# 8.0 and make it easier to work with complex data structures involving tuples.
using System;
public class Program
{
public static void Main()
{
var point = (3, 4);
string location = DescribePoint(point);
Console.WriteLine(location);
}
public static string DescribePoint((int X, int Y) point)
{
return point switch
{
(0, 0) => "The point is at the origin (0, 0).",
(_, 0) => "The point is on the x-axis.",
(0, _) => "The point is on the y-axis.",
var (x, y) when x == y => $"The point is on the diagonal at ({x}, {y}).",
var (x, y) => $"The point is at ({x}, {y}).",
};
}
}
var person = ("Alice", 30);
var description = person switch
{
("Alice", 30) => "This is Alice, age 30.",
("Bob", var age) => $"This is Bob, age {age}.",
_ => "Unknown person."
};
C#
‘Enhanced’ Type Pattern
You can do type checking in switch expressions without using the discards with each type
public string CheckValueType(object value)=> value switch
{
int => "integer number",
decimal => "decimal number",
double => "double number",
_ => throw new InvalidNumberException(value)
};
Pattern matching is a powerful feature in C# that simplifies code by allowing you to match objects and structures based on patterns. Whether you're checking types, values, or even properties, pattern matching makes your code more concise and readable.
Pattern matching in C# streamlines your code, reduces errors, and enhances readability, making it a valuable tool for developers.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
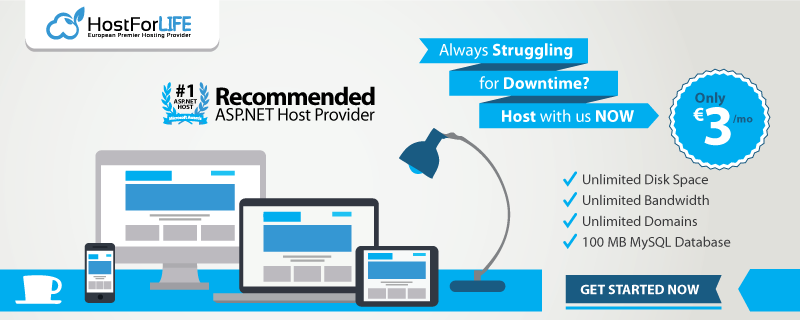