
September 19, 2023 08:34 by
Peter
Dependency injection is a technique for getting a dependant object from somewhere other than the class that was created with the new keyword.
Pros
- It improved modularity.
- It boosts testability.
- Place the dependent in a central location.
- Uncouple the system
The dependency injection (DI) software design pattern, which is a mechanism for accomplishing Inversion of Control (IoC) between classes and their dependencies, is supported by ASP.NET Core.
- Constructor Injection: The most common method of injecting dependencies into a class by giving them as constructor parameters.
- Method Injection is the process of injecting dependencies into a method as parameters.
- Property Injection is the process of populating a class's properties with the necessary dependencies.
We must provide the dependency's lifespan when we register it with DI Container (IServiceCollection).
Service Duration
The service lifetime determines how long an object lives once it is generated by the container. When registering, use the following method on the IServiceColletion to create the lifetime.
- Services are transient since they are produced each time they are requested.
- For example, with transitory tea makers, each customer gets a different tea. They get a one-of-a-kind tea experience because a new tea maker arrives for each order and serving.
- Services are created just once per request.
- In a teashop, for example, scored tea makers are equivalent to having one tea maker assigned to each table or group, giving the same type of tea to everyone within the group. When a new group arrives, they are assigned a tea maker.
- Singleton: A service is created only once for the duration of the program. For example, having a singleton tea maker in a teashop is equivalent to having a renowned tea master who provides the same tea to every customer. This tea master is constantly there, remembers all orders, and regularly serves everyone the same tea.
Let's now dive into the code to further explain this concept.
TeaService
public class TeaService : ITeaService
{
private readonly int _randomId ;
private readonly Dictionary<int, string> _teaDictionary = new()
{
{ 1, "Normal Tea ☕️" },
{ 2, "Lemon Tea ☕️" },
{ 3, "Green Tea ☕️" },
{ 4, "Masala Chai ☕️" },
{ 5, "Ginger Tea ☕️" }
};
public TeaService()
{
_randomId = new Random().Next(1, 5);
}
public string GetTea()
{
return _teaDictionary[_randomId];
}
}
public interface ITeaService
{
string GetTea();
}
RestaurantService: which injects TeaService.
public class RestaurantService : IRestaurantService
{
private readonly ITeaService _teaService;
public RestaurantService(ITeaService teaService)
{
_teaService = teaService;
}
public string GetTea()
{
return _teaService.GetTea();
}
}
public interface IRestaurantService
{
string GetTea();
}
Tea Controller: which is injecting TeaService and RestaurantService.
[Route("api/[controller]")]
[ApiController]
public class TeaController : ControllerBase
{
private readonly ITeaService _teaService;
private readonly IRestaurantService _restaurantService;
public TeaController(ITeaService teaService,
IRestaurantService restaurantService)
{
_teaService = teaService;
_restaurantService = restaurantService;
}
[HttpGet]
public IActionResult Get()
{
var tea = _teaService.GetTea();
var teaFromRestra = _restaurantService.GetTea();
return Ok($"From TeaService : {tea}
\nFrom RestaurantService : {teaFromRestra}");
}
}
Register services to DI Container.
a. Transient
// Add the below line to configure service in Statup.cs
services.AddTransient<ITeaService, TeaService>();
services.AddTransient<IRestaurantService, RestaurantService>();
Output
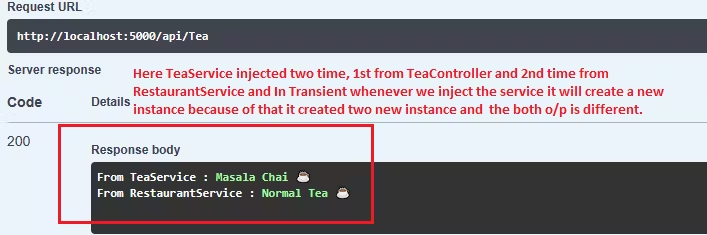
b. Scoped
// Add the below line to configure service in Statup.cs
services.AddScoped<ITeaService, TeaService>();
services.AddScoped<IRestaurantService, RestaurantService>();
Output
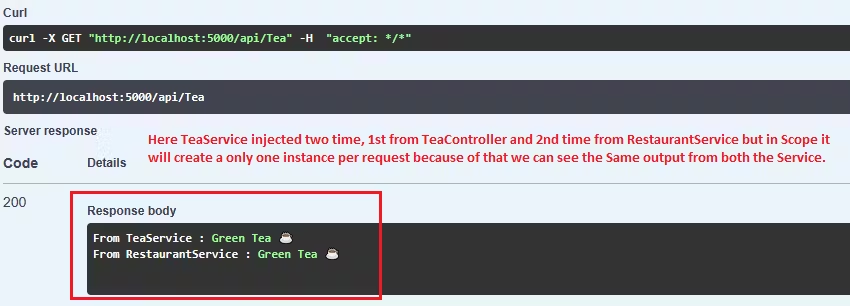
c: Singleton
// Add the below line to configure service in Statup.cs
services.AddSingleton<ITeaService, TeaService>();
services.AddSingleton<IRestaurantService, RestaurantService>();
Output
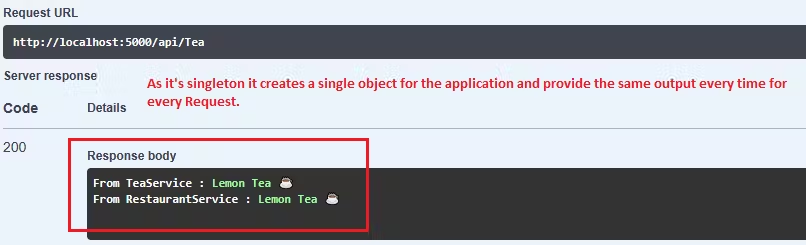
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
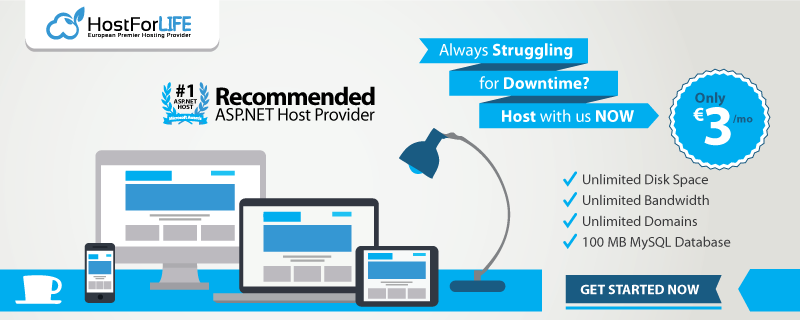