
July 30, 2024 07:11 by
Peter
According to the RFC 7515 standard, JWS (JSON Web Signature) is a small, URL-safe technique for securely conveying claims between two parties. It gives you the ability to digitally sign documents and guarantees that they weren't altered in transit. A particular kind of message authentication code (MAC) called HMAC (Hash-based Message Authentication Code) uses a secret cryptographic key along with a cryptographic hash function. When JWS is used, computing the JWS Signature with a secret key and a hash function is known as employing HMAC.
Why Use JWS HMAC?
- Integrity and Authenticity: JWS with HMAC provides both data integrity and authentication. The signature ensures that the data has not been altered, and since the HMAC key is secret, it can verify that the sender (or signer) of the JWT is who they claim to be.
- Security: HMAC is considered a strong method of ensuring data integrity because it involves a secret key, which makes it difficult to forge compared to non-keyed hashes.
- Compactness: JWS provides a compact way to securely transmit information via URLs, HTTP headers, and within other contexts where space is limited.
How to Use JWS HMAC in an ASP.NET Web Application?
You'll usually be working with JWT (JSON Web Tokens), where JWS forms the signed and encoded string, in order to employ JWS HMAC in an ASP.NET application. Here's how to put this into practice:
Step 1. Install Necessary NuGet Package
A JWT-capable library is required. System is a well-liked option.IdentityModel.Coins.JWT. NuGet can be used to install it.
Install-Package System.IdentityModel.Tokens.Jwt
Step 2. Create and Sign a JWT with HMAC
Here's how you can create a JWT and sign it using HMAC in your ASP.NET application.
using System;
using Microsoft.IdentityModel.Tokens;
using System.IdentityModel.Tokens.Jwt;
using System.Text;
using System.Security.Claims;
public class TokenService
{
public string GenerateToken()
{
var secretKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your-256-bit-secret"));
var signinCredentials = new SigningCredentials(secretKey, SecurityAlgorithms.HmacSha256);
var tokenOptions = new JwtSecurityToken(
issuer: "https://yourdomain.com",
audience: "https://yourdomain.com",
claims: new List<Claim>(),
expires: DateTime.Now.AddMinutes(30),
signingCredentials: signinCredentials
);
var tokenString = new JwtSecurityTokenHandler().WriteToken(tokenOptions);
return tokenString;
}
}
Explanation
- Secret Key: This is a key used by HMAC for hashing. It should be kept secret and secure.
- Signing Credentials: Uses the secret key and specifies the HMAC SHA256 algorithm for signing.
- JwtSecurityToken: Represents the JWT data structure and allows setting properties like issuer, audience, claims, expiry time, etc.
- JwtSecurityTokenHandler: Handles the creation of the token string.
Step 3. Validate the JWT in ASP.NET
When you receive a JWT, you need to validate it to ensure it's still valid and verify its signature.
public ClaimsPrincipal ValidateToken(string token)
{
var tokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = "https://www.hostforlifeasp.net",
ValidAudience = "https://www.hostforlifeasp.net",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your-256-bit-secret"))
};
var tokenHandler = new JwtSecurityTokenHandler();
SecurityToken validatedToken;
var principal = tokenHandler.ValidateToken(token, tokenValidationParameters, out validatedToken);
return principal;
}
Note. Please change www.hostforlifeasp.net to www.yourdomain.com
This function uses JwtSecurityTokenHandler to validate the token and sets up the parameters (issuer, audience, lifetime, and signature key) that require validation. It throws an exception if the token is invalid and returns a ClaimsPrincipal with the token's claims.
Conclusion
A secure method for managing tokens for information exchange and authentication in ASP.NET is to use JWS HMAC. It gives you piece of mind and security for your online applications by making sure the tokens are authentic and unaltered.
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
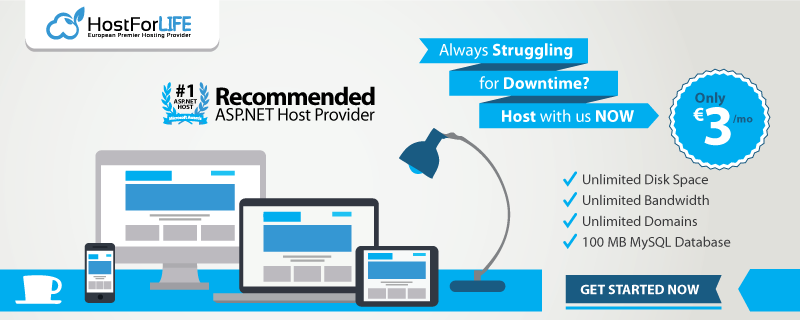