This post will show you how to use Visual Studio Community 2022 to construct a Document Viewer in an Asp.Net Core 7.0 application.
What exactly is GroupDocs.Viewer?
GroupDocs.Viewer is a document viewing and rendering package created by GroupDocs, a business that specializes in document manipulation and conversion APIs for.NET and Java. GroupDocs.Viewer is intended to assist developers in incorporating document viewing capabilities into their applications, allowing users to read a variety of documents right within the application's interface without the need to download or open them with other software.
Let's get started with the document viewer.
In _Layout.html, import the bootstrap 5 cdn.
Install the Nuget Package GroupDocs.Viewer
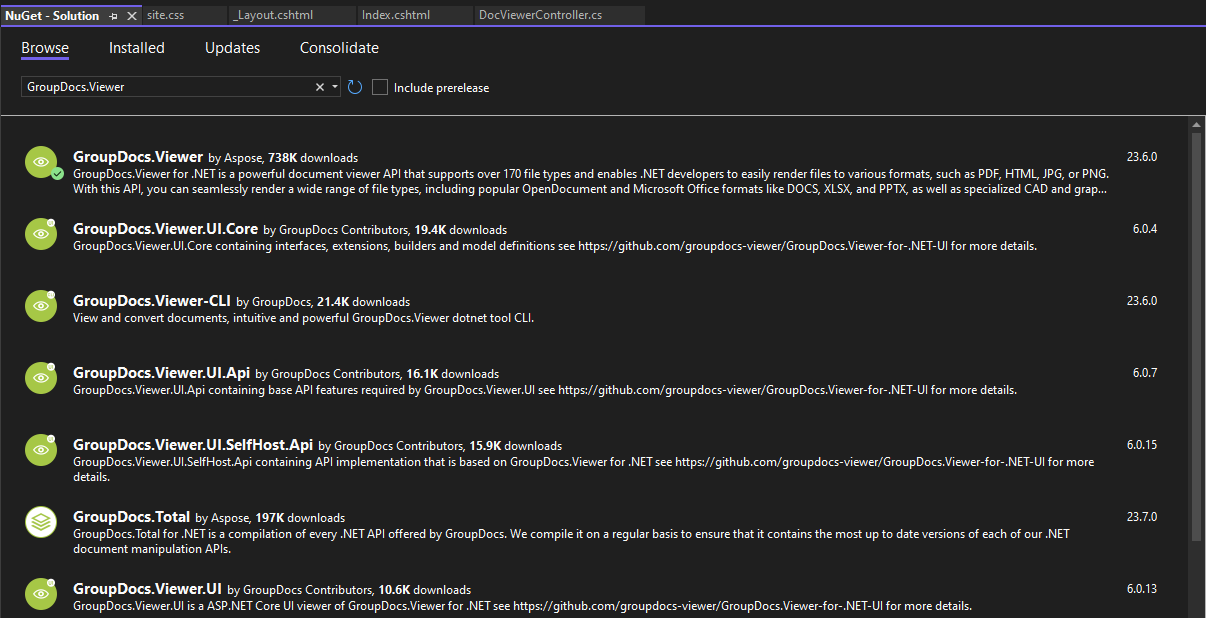
Make a fresh ASP.NET CORE MVC project.
DocumentViewer.Application is the name of the project.
In the DocumentViewer.Application project, add a new controller.
public class DocViewerController : Controller
{
private readonly IHostingEnvironment _hostingEnvironment;
private string projectRootPath;
private string outputPath;
private string storagePath;
List<string> lstFiles;
public DocViewerController(IHostingEnvironment hostingEnvironment)
{
_hostingEnvironment = hostingEnvironment;
projectRootPath = _hostingEnvironment.ContentRootPath;
outputPath = Path.Combine(projectRootPath, "wwwroot/Content");
storagePath = Path.Combine(projectRootPath, "storage");
lstFiles = new List<string>();
}
public IActionResult Index()
{
var files = Directory.GetFiles(storagePath);
foreach (var file in files)
{
lstFiles.Add(Path.GetFileName(file));
}
ViewBag.lstFiles = lstFiles;
return View();
}
[HttpPost]
public IActionResult OnPost(string FileName)
{
int pageCount = 0;
string imageFilesFolder = Path.Combine(outputPath, Path.GetFileName(FileName).Replace(".", "_"));
if (!Directory.Exists(imageFilesFolder))
{
Directory.CreateDirectory(imageFilesFolder);
}
string imageFilesPath = Path.Combine(imageFilesFolder, "page-{0}.png");
using (Viewer viewer = new Viewer(Path.Combine(storagePath, FileName)))
{
//Get document info
ViewInfo info = viewer.GetViewInfo(ViewInfoOptions.ForPngView(false));
pageCount = info.Pages.Count;
//Set options and render document
PngViewOptions options = new PngViewOptions(imageFilesPath);
viewer.View(options);
}
return new JsonResult(pageCount);
}
}
The method marked with the [HttpPost] tag, which indicates that it responds to HTTP POST requests, lies at the heart of this code. The method accepts a FileName parameter, which is the name of the uploaded file. The method returns an IActionResult, which allows for the return of many forms of replies, such as JSON, views, or redirects.
The code starts by creating a folder in which to save the created PNG images. The name of the folder is determined by the FileName option, with any dots in the filename replaced by underscores. If the folder does not already exist, the code uses the Directory to create it.The method CreateDirectory.
By combining the storage location and the FileName parameter, the using block is utilized to generate a Viewer object. This Viewer object is responsible for interacting with the document file.
For saving images, a PngViewOptions object is created and specified using the imageFilesPath pattern. The created choices are then passed to the View method of the viewer. This phase transforms the pages of the document as PNG images and saves them to the chosen folder.
The pageCount variable, which reflects the total number of pages in the document, is returned in the JSON response. The client or caller can utilize this JSON response to obtain this information.
Create an Index.cshtml file now.
In index.cshtml, we use an Ajax call.
@{
}
<script src="http://code.jquery.com/jquery-1.8.2.js"></script>
<script>
function ViewDocument(file) {
$("#loader").fadeIn();
var data = { FileName: file };
$.ajax({
type: "POST",
url: '/DocViewer/OnPost',
data: data,
dataType: "text"
}).done(function (data) {
var folderName = file.replace(".", "_");
$("#content").empty();
for (var i = 1; i <= data; i++) {
$("#content").append("<img class='img-fluid' src='Content/" + folderName + "/page-" + i + ".png'/>");
}
$("#loader").fadeOut();
})
}
</script>
<script type="text/javascript">
$(window).load(function () {
$("#loader").fadeOut(1000);
});
</script>
<div class="container">
<div class="row">
<div class="col-md-3">
<div class="sidenav bg-light p-3">
<div id="loader"></div>
<h2 class="ps-3">Files</h2>
@if (ViewBag.lstFiles != null)
{
@foreach (string file in ViewBag.lstFiles)
{
<a href="#" onclick="ViewDocument('@file')" class="d-block">@file</a>
}
}
</div>
</div>
<div class="col-md-9">
<h2>Preview</h2>
<div id="content" class="border p-3"></div>
</div>
</div>
</div>
Output:
Image preview
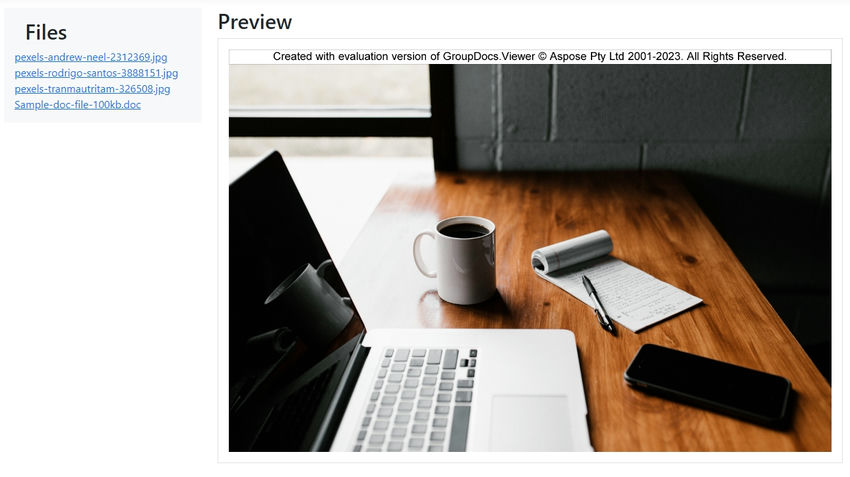
Document file preview
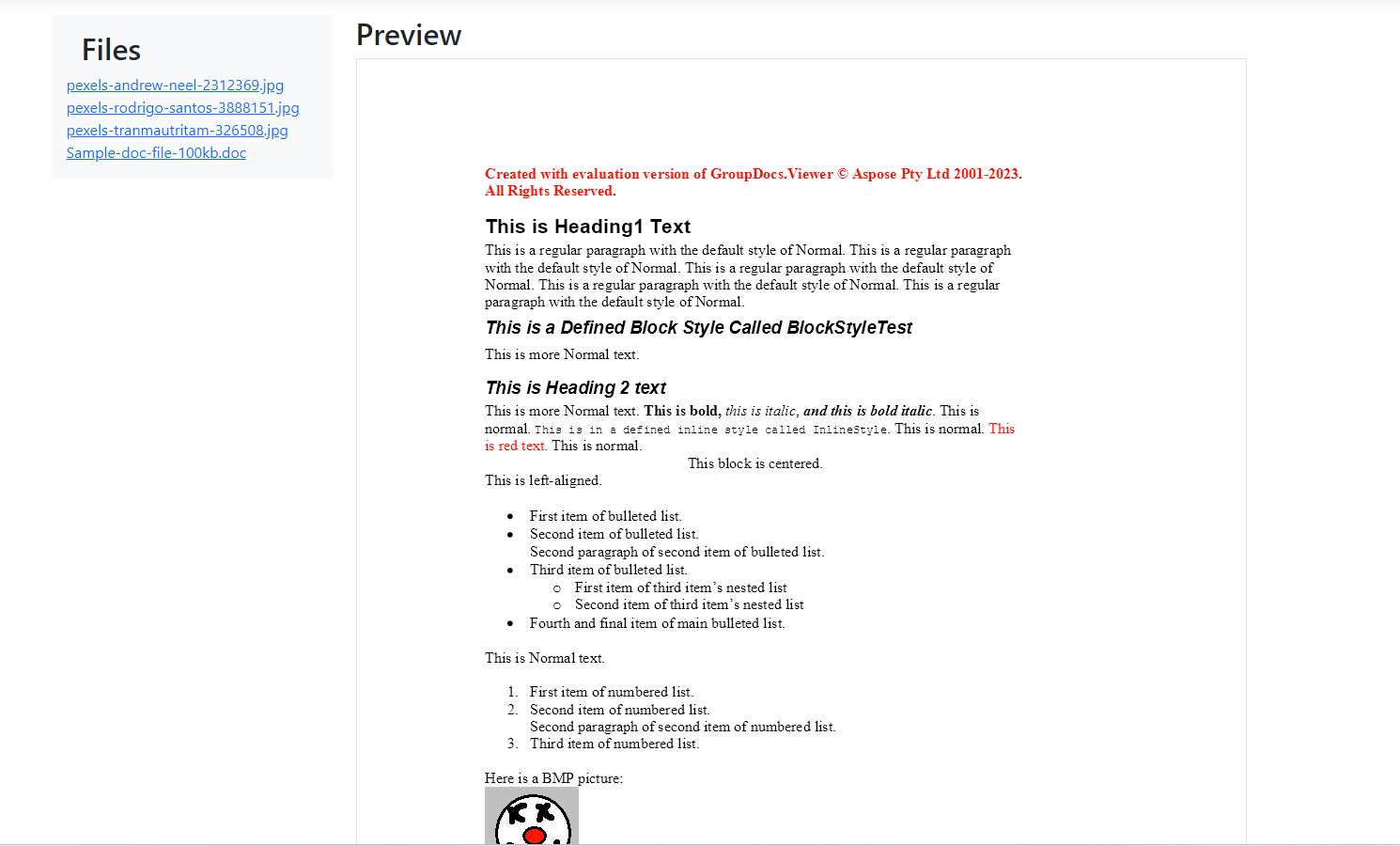
GroupDocs.Viewer is a software development tool that offers APIs and libraries for viewing and visualizing different sorts of documents and files within applications. It enables developers to incorporate document viewing features into their apps without requiring users to install the native applications that generated those documents. The application supports a variety of document formats, including PDF, Microsoft Office (Word, Excel, PowerPoint), pictures, AutoCAD files, and others.