In order to comprehend and improve the performance of their applications, developers can benefit greatly from the use of monitoring and profiling in software development and maintenance. Let's investigate profiling and monitoring inside the framework of web apps, with an emphasis on Angular applications specifically.
Observing
Monitoring of Angular Performance
Angular Performance Explorer is an integrated tool that comes with Angular.
Some popular tools include.
1. Explorer of Angular Performance
Angular Performance Explorer is an integrated tool that comes with Angular. This tool gives you information on how well Angular components, directives, and services are performing. It can be accessed using the developer tools in your browser. It's also useful to you.
Examine how long the lifecycle hooks for each component take.
Examine the cycles of change detection and locate any possible bottlenecks.
Track the duration of each component's rendering.
To access the Angular Performance Explorer
Open Chrome DevTools (F12 or right-click and select "Inspect").
Navigate to the "Performance" tab.
Click on the "Angular" sub-tab.
2. Google Chrome DevTools
Google Chrome DevTools offers a powerful set of tools for monitoring and debugging Angular applications. The "Performance" tab within DevTools allows you to.
Record and analyze runtime performance.
Identify CPU and memory usage.
Understand the timeline of events during a user interaction or page load.
To use Chrome DevTools for Angular performance monitoring.
Open Chrome DevTools (F12 or right-click and select "Inspect").
Navigate to the "Performance" tab.
Record a performance profile by clicking the red circle.
Perform the desired actions in your Angular application.
Stop the recording by clicking the red square.
3. Real User Monitoring (RUM) Tools
Consider using Real User Monitoring (RUM) tools such as New Relic, Datadog, or others. These tools provide insights into how real users are experiencing your Angular application, including page load times, AJAX requests, and user interactions. Integrating RUM tools typically involves adding a monitoring script to your application, which then sends performance data to the chosen monitoring service.
Logging and Error Tracking
Logging and error tracking are essential components of any application's monitoring and maintenance strategy. They help developers identify issues, troubleshoot problems, and improve overall application stability. In the context of Angular applications, here are some strategies for logging and error tracking:
Logging in Angular
1. Console Logging
Angular applications can utilize standard console logging for debugging purposes. You can use console.log(), console. warn(), and console.error() statements to output information to the browser's console.
// Example component with console logging
@Component({
selector: 'app-example',
template: '<p>Example Component</p>',
})
export class ExampleComponent implements OnInit {
ngOnInit() {
console.log('Component initialized');
}
}
3. Angular Logging Services
For more sophisticated logging, consider using third-party logging libraries or services. Popular ones include.
Angular Logger Service: A custom logging service that can be injected into Angular components and services.
Ngx Logger: A configurable logging service for Angular applications.
Error Tracking
1. Global Error Handling
Implement a global error handler to catch unhandled errors throughout your Angular application. You can use the ErrorHandler service provided by Angular to customize error handling.
// Example global error handler
@Injectable()
export class GlobalErrorHandler implements ErrorHandler {
handleError(error: any): void {
// Handle the error (e.g., log it, send to an error tracking service)
console.error('Global error handler:', error);
}
}
In your AppModule, provide this error handler.
@NgModule({
providers: [
{ provide: ErrorHandler, useClass: GlobalErrorHandler },
],
})
export class AppModule { }
2. Third-Party Error Tracking Services
Integrate third-party error tracking services to monitor and receive alerts about errors in your application. Popular services include.
Sentry: A platform for real-time error tracking and monitoring.
Rollbar: A cloud-based error tracking and monitoring service.
Bugsnag: A tool for detecting and diagnosing application errors.
To integrate Sentry, for example, you would typically install the Sentry package and configure it in your Angular application.
npm install @sentry/angular @sentry/tracing
// Import and configure Sentry in your AppModule
import { SentryModule } from '@sentry/angular';
import { Integrations } from '@sentry/tracing';
Sentry.init({
dsn: 'YOUR_DSN',
integrations: [
new Integrations.BrowserTracing(),
],
});
@NgModule({
imports: [SentryModule],
})
export class AppModule { }
Logging and Error Tracking Best Practices
Environment-Specific Configuration: Configure logging and error tracking differently based on the environment (development, staging, production). For example, you might want more verbose logging in development but less in production.
Include Context Information: When logging or tracking errors, include relevant context information such as user details, browser version, and the application's state.
Security Considerations: Ensure that sensitive information is not exposed in logs or error reports. Be mindful of data privacy and security concerns.
Regular Monitoring: Regularly review logs and error reports to identify patterns, trends, and potential areas for optimization.
Continuous Improvement: Iteratively improve logging and error tracking based on the insights gained from monitoring and analysis.
Profiling
Profiling is the process of analyzing and measuring various aspects of a program's performance to identify bottlenecks, optimize resource usage, and improve overall efficiency. In the context of Angular applications, profiling can help developers understand how the application behaves and where performance improvements can be made. Here are some profiling techniques and tools for Angular.
Angular Profiler
Angular itself provides a built-in profiler that can be enabled using the Angular CLI. This profiler offers insights into change detection, rendering, and other Angular-specific metrics.
To enable the Angular profiler, you can use the following command when starting your Angular application.
ng serve --profile
This enables the Angular profiler, which provides additional information about change detection, rendering, and other performance-related metrics.
Chrome DevTools
1. Performance Tab
Chrome DevTools is a powerful set of tools for debugging and profiling web applications. The "Performance" tab in Chrome DevTools allows you to record and analyze runtime performance, CPU usage, memory allocation, and other metrics.
To use the Performance tab.
- Open Chrome DevTools (F12 or right-click and select "Inspect").
- Navigate to the "Performance" tab.
- Record a performance profile by clicking the red circle.
- Perform the desired actions in your Angular application.
- Stop the recording by clicking the red square.
2. Memory Tab
The "Memory" tab in Chrome DevTools helps you analyze memory usage in your application. It can be useful for identifying memory leaks and optimizing memory consumption.
Augury
Angury is a Chrome and Firefox DevTools extension specifically designed for debugging and profiling Angular applications. It provides additional insights into the structure, state, and performance of your Angular application.
To use Augury
Install the Augury extension from the Chrome Web Store or Firefox Add-ons.
Open Chrome DevTools or Firefox Developer Tools.
Navigate to the "Augury" tab.
Web Performance Testing
1. Lighthouse
Lighthouse is an open-source, automated tool for improving the quality of web pages. It has audits for performance, accessibility, progressive web apps, SEO, and more.
You can run Lighthouse from the Chrome DevTools or use the Lighthouse CLI to perform audits on your Angular application.
# Install Lighthouse globally
npm install -g lighthouse
# Run Lighthouse on your Angular app
lighthouse http://localhost:4200
Webpack Bundle Analyzer
If you want to analyze the size of your application bundles, you can use tools like Webpack Bundle Analyzer. This tool visualizes the size of your bundles and dependencies, helping you identify areas for optimization.
# Install Webpack Bundle Analyzer
npm install --save-dev webpack-bundle-analyzer
Then, add a script in your package.json to run the analyzer
"scripts": {
"analyze": "webpack-bundle-analyzer dist/your-app-name/stats.json"
}
Run the script after building your Angular application.
npm run build -- --prod
npm run analyze
Profiling tools and techniques are essential for identifying performance bottlenecks and optimizing Angular applications. Regularly profile your application, especially during development and before deploying to production, to ensure optimal performance.
Best Practices
1. Code Splitting: Implement code splitting to reduce the initial load time of your Angular application. This involves breaking your application into smaller modules that are loaded on demand.
2. Lazy Loading: Take advantage of Angular's lazy loading feature to load modules only when they are needed, improving the initial page load time.
3. Optimize Change Detection: Angular's change detection can be resource-intensive. Optimize it by using the OnPush change detection strategy and avoiding unnecessary triggers.
4. Bundle Optimization: Configure your build tools (such as Angular CLI or Webpack) to optimize and minify your application bundles for production. This reduces the overall size of your application, improving load times.
5. Caching Strategies: Implement proper caching strategies for assets and API calls to reduce the number of network requests and improve the overall performance of your application.
6. Performance Budgets: Set performance budgets to establish acceptable thresholds for metrics like page load time, bundle size, and other critical performance indicators.
7. Continuous Monitoring: Implement continuous monitoring practices to catch performance issues early in development and production. This can include automated tests, performance regression testing, and monitoring tools integrated into your CI/CD pipeline.
By combining monitoring and profiling techniques with best practices, you can ensure that your Angular application is not only performant but also maintainable and scalable. Regularly revisit and adjust your strategies as your application evolves to keep it optimized over time.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
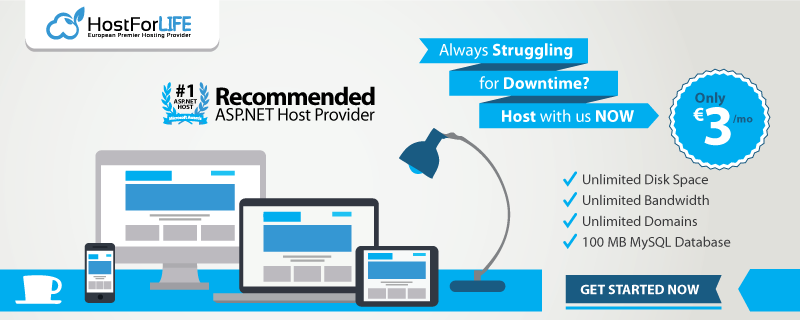