Validation of data is an important part of web application development. It guarantees that the information submitted by users is correct, safe, and follows the anticipated format. FluentValidation in ASP.NET Core provides a powerful and easy way to handle validation logic, allowing developers to validate user input while maintaining clean, maintainable code.
What is ASP.NET Core FluentValidation?
FluentValidation is a well-known.NET library for creating strongly typed validation rules. FluentValidation, as opposed to traditional attribute-based validation, provides a more expressive syntax and allows developers to construct validation logic in a distinct, clean, and reusable manner. It integrates effortlessly into ASP.NET Core applications, providing a strong solution for validating user input.To begin using FluentValidation, create an ASP.NET Core Web API project.
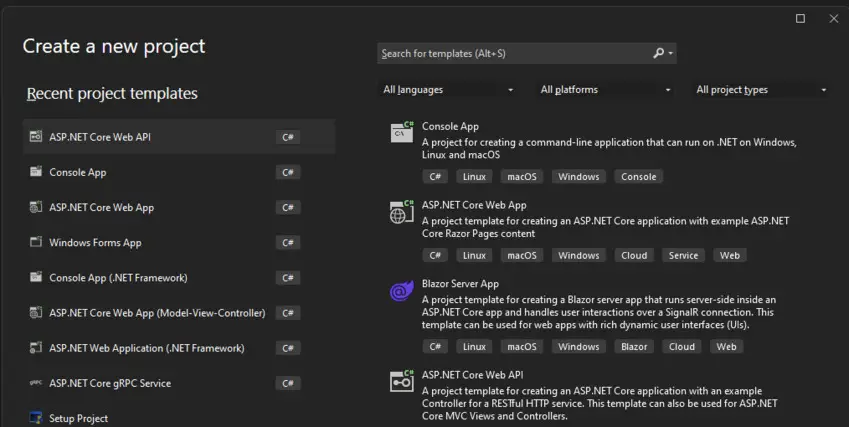
After you've created the project, you'll need to add two NuGet packages to it.
NuGet\Install-Package FluentValidation -Version 11.7.1
NuGet\Install-Package FluentValidation.DependencyInjectionExtensions -Version 11.7.1
After the installation is complete, you will be able to define validation criteria for the models you generate.
Developing Validation Rules
Consider a straightforward registration form with fields such as username, email, and password. Create a validator class and an associated model to validate this form:
public class UserRegistrationModel
{
public string Username { get; set; }
public string Email { get; set; }
public string Password { get; set; }
}
public class UserRegistrationValidator : AbstractValidator<UserRegistrationModel>
{
public UserRegistrationValidator()
{
RuleFor(x => x.Username).NotEmpty().MinimumLength(3);
RuleFor(x => x.Email).NotEmpty().EmailAddress();
RuleFor(x => x.Password).NotEmpty().MinimumLength(6);
}
}
After the installation is complete, you will be able to define validation criteria for the models you generate.
Developing Validation Rules
Consider a straightforward registration form with fields such as username, email, and password. Create a validator class and an associated model to validate this form:
builder.Services.AddScoped<IValidator<UserRegistrationModel>, UserRegistrationValidator>(); // register validators
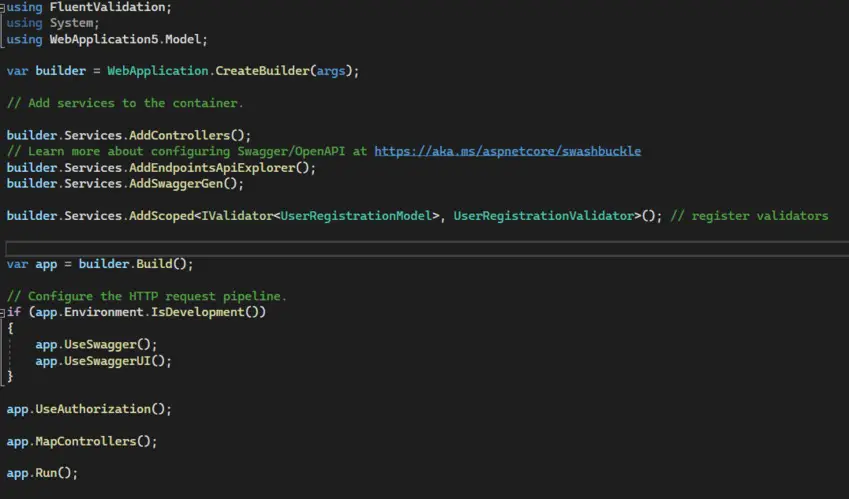
Including Validation in Controllers
You can now utilize the validator in your controller to validate the model.
[HttpPost]
public IActionResult Register(UserRegistrationModel model)
{
var validator = new UserRegistrationValidator();
var validationResult = validator.Validate(model);
if (!validationResult.IsValid)
{
return BadRequest(validationResult.Errors);
}
// Process registration logic if the model is valid
return Ok("Registration successful!");
}
In this case, the Register action method validated the receiving UserRegistrationModel with UserRegistrationValidator. If the model is invalid, a BadRequest response containing the validation errors is returned.
Please test it with Postman to check that it is working properly. Please let me know if you require any assistance in setting up the test.
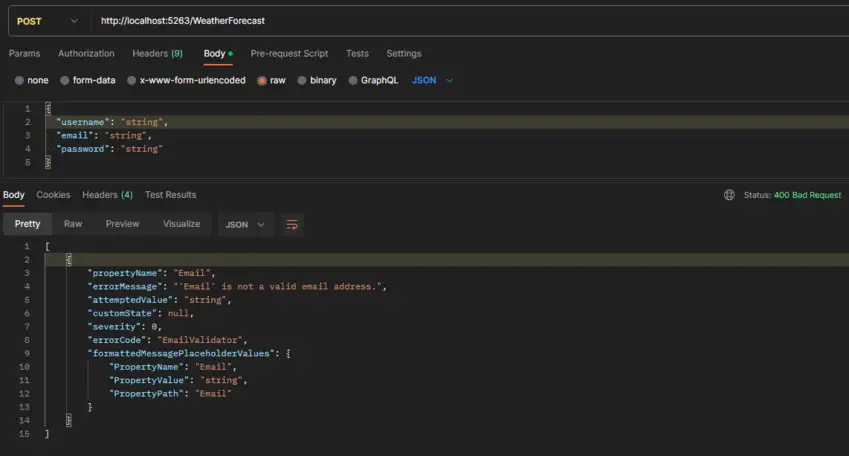
The validation appears to be working well now.
FluentValidation streamlines the data validation process in ASP.NET Core apps. Developers can design complicated validation rules in a legible and maintainable manner by providing a fluent and expressive API. You can ensure that your apps process user input accurately and securely by introducing FluentValidation into your ASP.NET Core projects, resulting in a more robust and dependable user experience.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
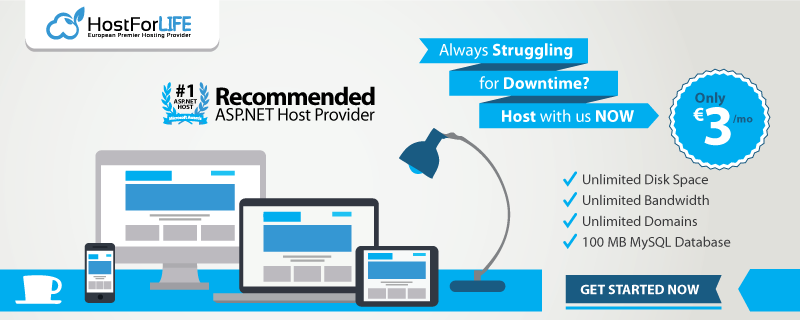