An essential component of online application security is session management. We fix a widespread issue in this post that lets potential attackers reuse existing session IDs to obtain unauthorized access to ASP.NET sessions even after they have been logged out. We'll go over how to apply best practices like SSL/TLS and secure cookie settings, as well as how to correctly invalidate sessions upon logout and regenerate session IDs upon login. You can safeguard your ASP.NET application against replay and session fixation attacks and provide a safer environment for your users by adhering to these rules.
How to Handle the Problem
1. Upon logout, invalidate the session
Make sure that when the user logs out, the session is appropriately invalidated. You can accomplish this by using Session.Abandon() in your logout procedure.
An illustration of the logout action
Example in Logout Action
public ActionResult Logout()
{
// Clear the session
Session.Abandon();
Session.Clear();
// Clear authentication cookies
FormsAuthentication.SignOut();
// Redirect to the login page or home page
return RedirectToAction("Login", "Account");
}
Explanation
- Session.Abandon() marks the session as abandoned, which means that the session will no longer be used and a new session will be created for the next request.
- Session.Clear() removes all items from the session.
- FormsAuthentication.SignOut() logs the user out and clears the authentication ticket.
2. Regenerate the Session ID Upon Login
It's important to regenerate the session ID after a successful login to prevent session fixation attacks. This ensures that any previous session ID is no longer valid.
Example
Explanation
The SessionIDManager is used to create a new session ID, which is then saved to the current session. This ensures that after logging in, the user’s session ID is different from the one used before authentication.
3. Enforce Session ID expiration
Set a shorter session timeout to minimize the risk of an old session ID being used.
Web. config Setting
Explanation
The timeout attribute specifies the number of minutes a session can be idle before it is abandoned. A shorter timeout can reduce the risk of session reuse.
4. Use SSL/TLS
Ensure that your application uses SSL/TLS to protect the session ID in transit. This prevents attackers from capturing the session ID via network sniffing.
5. Secure Cookies
Mark the session cookies as HttpOnly and Secure to prevent client-side access to the session ID and ensure they are only transmitted over secure connections.
Web. config Setting
Explanation
- requireSSL="true" ensures that cookies are only sent over HTTPS.
- cookieSameSite="Strict" helps prevent CSRF attacks by limiting the conditions under which cookies are sent.
Summary
By ensuring that the session is properly invalidated on logout, regenerating the session ID upon login, setting session expiration policies, and securing your application with SSL/TLS and secure cookies, you can effectively mitigate the risk of session fixation and replay attacks. This will enhance the overall security of your ASP.NET application.
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
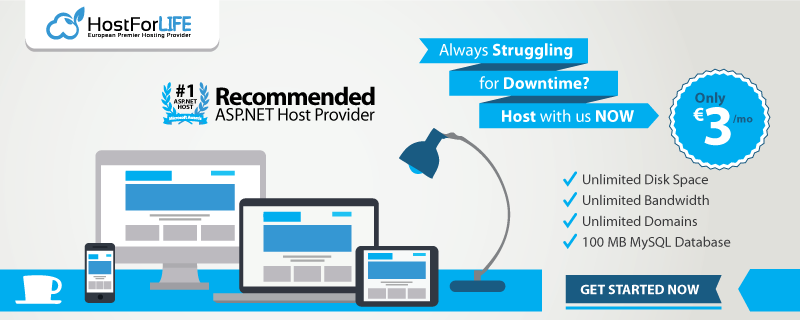