
August 2, 2021 07:49 by
Peter
In this article, you will learn how to integrate Mailjet Markup Language (MJML) in your ASP .Net Core Web API through the NuGet package. MJML sums up everything Mailjet has learned about HTML email creation over the past few years and includes a whole layer of complexity related to responsive email creation.
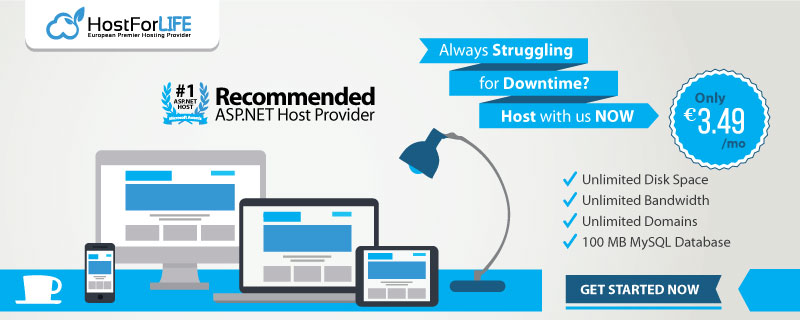
Make your speed and productivity enhanced with MJML semantic syntax. Say get rid of the endless HTML table or email client email. Creating a responsive email is much easier with tags like <mj-section>, <mj-image>, <mj-text> and <mj-column>. MJML is designed with responsiveness in mind. The abstraction it offers ensures you stay up-to-date with industry practice and responsiveness.
The only thing we do in our API is to add the NuGet package of MJML. Then we will write the MJML syntax for our email template and then we will call the “Render” method of the MJML Service which returns the HTML. We will simply pass it to our email body and it will be rendered in our email.
Let’s see how to do it in simple steps.
Steps
First of all open visual studio and click on create a new project.
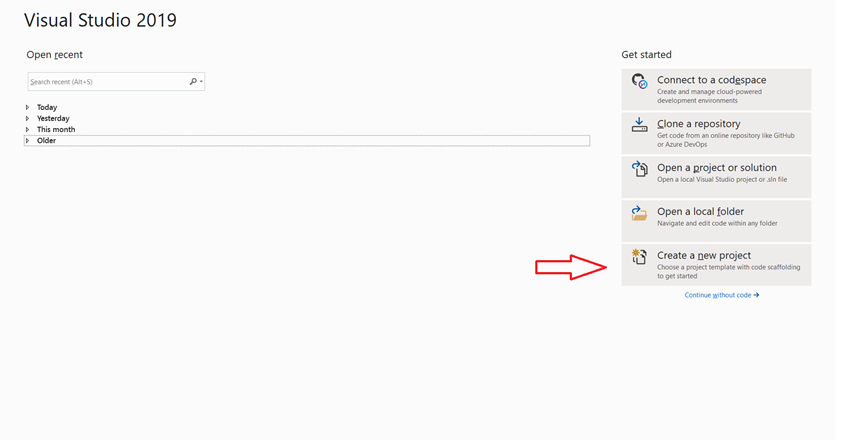
Select ASP .NET Core Web API and click Next.
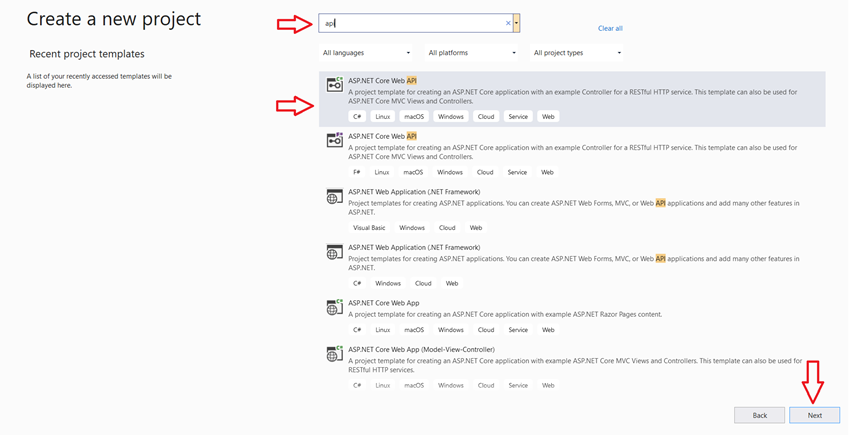
Enter the project name and click Next.
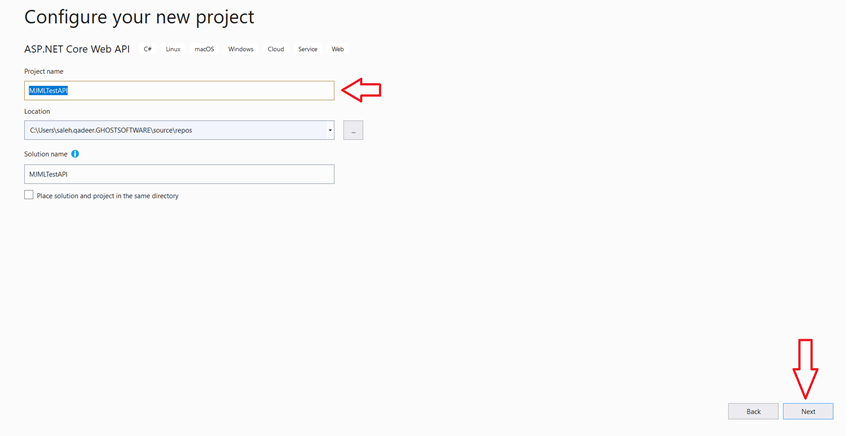
Select target framework as 3.1 and click on Create.
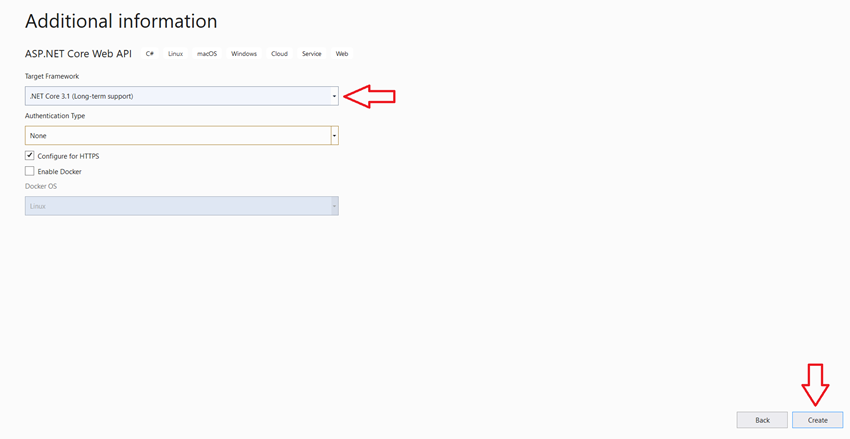
It will take few seconds and your project will be created. There will be a few folders and files in the project already. Right-click on the Controller folder and add a new empty MVC Controller.
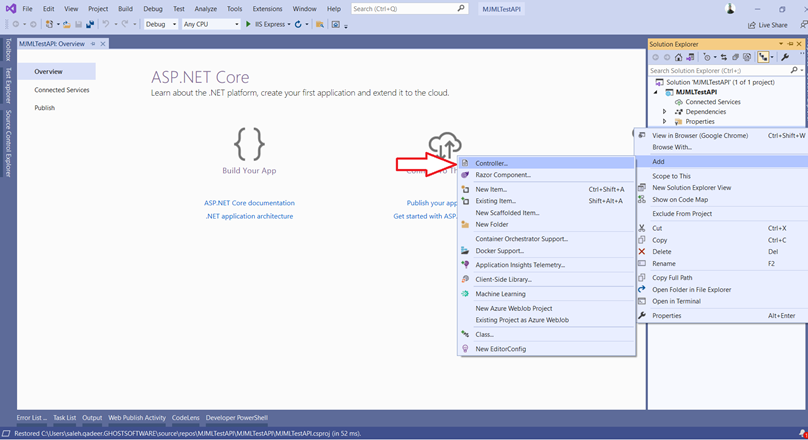
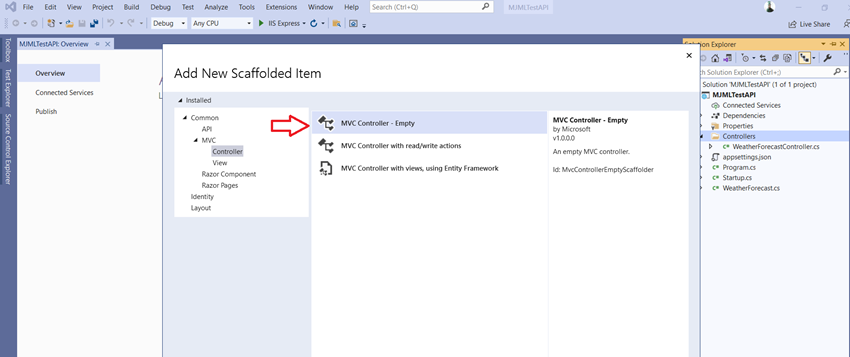
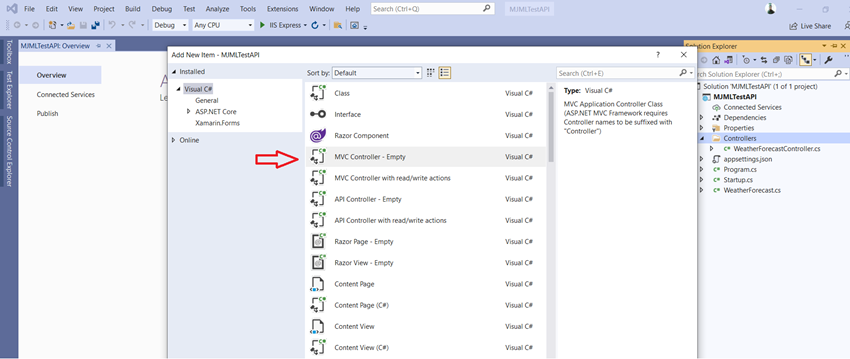
Name the controller as “EmailController”. After creating the EmailController right-click on your project and click on manage NuGet packages. Write MJML in the browse tab and install the latest stable version of Mjml.AspNetCore package.
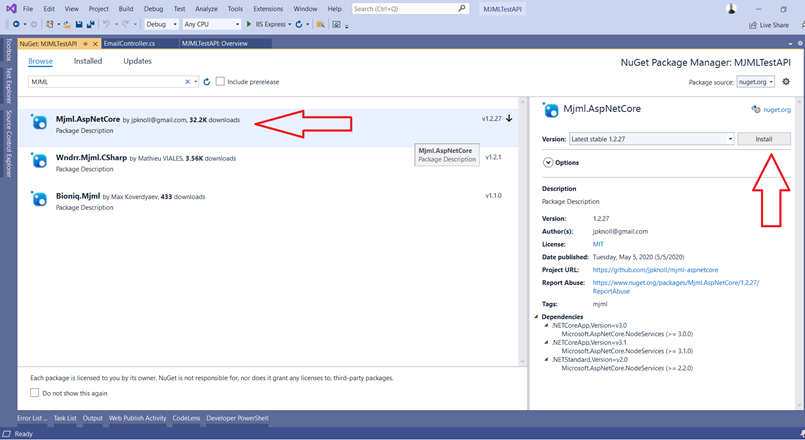
After installing the package add the namespace of “using Mjml.AspNetCore” in EmailController. You can use dependency injection which will make it much easier to use Mjml Services. For this purpose, write the below code in your “ConfigureServices” method in the startup.cs class and also add namespace “using Mjml.AspNetCore”.
services.AddMjmlServices(o => {
o.DefaultKeepComments = true;
o.DefaultBeautify = true;
});
We have successfully created the Mjml service now it’s time to inject it into our controller. Inject “MjmlServices” through the constructor and create a HttpGet method by the name “Send” which returns Ok in case of successful email send. After that write the Mjml syntax for your email template and call the “Render” method of the Mjml service. It will return HTML in response. Simply pass that HTML as your email body. Below is the complete code of the EmailController.
using Microsoft.AspNetCore.Mvc;
using Mjml.AspNetCore;
using System;
using System.Net;
using System.Net.Mail;
using System.Threading.Tasks;
namespace MJMLTestAPI.Controllers {
[ApiController]
[Route("[controller]")]
public class EmailController: ControllerBase {
public readonly IMjmlServices _mjmlServices;
public EmailController(IMjmlServices _mjml) => (_mjmlServices) = (_mjml);
[HttpGet]
public IActionResult Send() {
SendEmail();
return Ok();
}
public async Task SendEmail() {
var prehtml = "<mjml><mj-body><mj-container><mj-section><mj-column><mj-text>Hello World!</mj-text></mj-column></mj-section></mj-container></mj-body></mjml>";
var result = await _mjmlServices.Render(prehtml);
SendEmail(result.Html);
}
public void SendEmail(string emailBody) {
try {
SmtpClient objsmtp = new SmtpClient(EmailServer, Port);
objsmtp.UseDefaultCredentials = false;
objsmtp.Credentials = new NetworkCredential(UserName, Password);
objsmtp.DeliveryMethod = SmtpDeliveryMethod.Network;
MailMessage msg = new MailMessage(EmailFrom, EmailTo, "MJML Test", emailBody);
msg.IsBodyHtml = true;
objsmtp.Timeout = 50000;
objsmtp.Send(msg);
} catch (Exception ex) {}
}
}
}
Note that the variables (EmailServer, Port, UserName, Password, EmailFrom, EmailTo) in the above SendEmail method will be the settings of your SMTP email server. “prehtml” will be the MJML syntax by which you designed your email template. I used a sample syntax here. You can see sample email templates and their MJML code by visiting this link.
Now, just run your project and replace the weatherforecast in the URL with Email, and press enter button. The email will be sent to your desired email address with the generated email template in the email body.
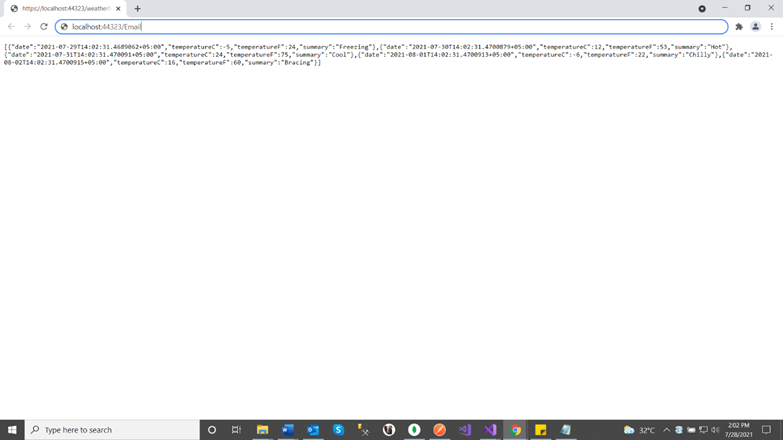
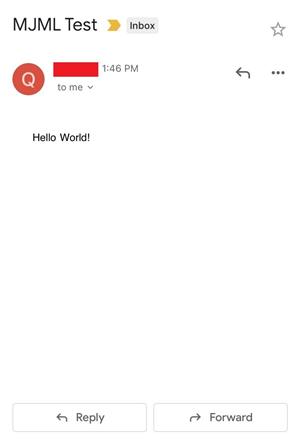