HttpClient class provides a base class for sending/receiving the HTTP requests/responses from a URL. It is a supported async feature of .NET framework. HttpClient is able to process multiple concurrent requests. It is a layer over HttpWebRequest and HttpWebResponse. All methods with HttpClient are asynchronous.
In this example, I have created a console application.
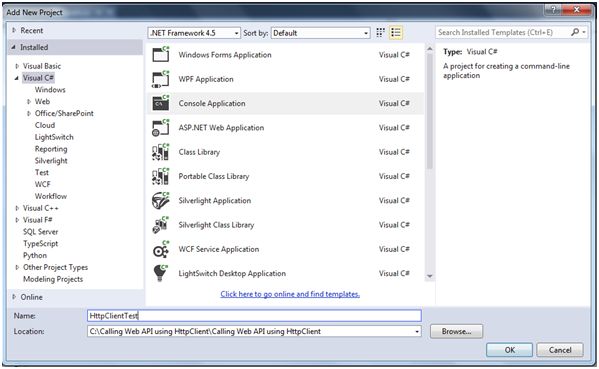
To call Web API methods from the console Application, the first step is to install the required packages, using NuGet Package Manager. The following package needs to be installed in the console Application.
Install-Package Microsoft.AspNet.WebApi.Client
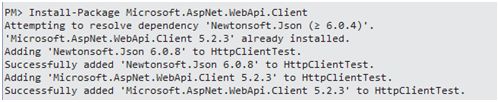
Next step is to create HttpClient object. In the following code snippet, the main function calls private static method "CallWebAPIAsync" and this blocks until CallWebAPIAsync completes the process, using wait function.
static void Main(string[] args)
{
CallWebAPIAsync()
.Wait();
}
static asyncTaskCallWebAPIAsync()
{
using(var client = newHttpClient())
{
//Send HTTP requests from here.
}
}
Afterwards, we have set the base URL for the HTTP request and set the Accept header. In this example, I have set Accept header to "application/json" which tells the Server to send the data into JSON format.
using(var client = newHttpClient())
{
client.BaseAddress = newUri("http://localhost:55587/");
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(newMediaTypeWithQualityHeaderValue("application/json"));
}
HTTP GET Request
Following code is used to send a GET request for department, as shown below:
using(var client = newHttpClient())
{
client.BaseAddress = newUri("http://localhost:55587/");
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(newMediaTypeWithQualityHeaderValue("application/json"));
//GET Method
HttpResponseMessage response = awaitclient.GetAsync("api/Department/1");
if (response.IsSuccessStatusCode)
{
Departmentdepartment = awaitresponse.Content.ReadAsAsync < Department > ();
Console.WriteLine("Id:{0}\tName:{1}", department.DepartmentId, department.DepartmentName);
Console.WriteLine("No of Employee in Department: {0}", department.Employees.Count);
}
else
{
Console.WriteLine("Internal server Error");
}
}
In the code given above, I have used GetAsync method to send HTTP GET request asynchronously. When the execution of this method is finished, it returns HttpResponseMessage, which contains HTTP response. If the response contains success code as response, it means the response body contains the data in the form of JSON. ReadAsAsync method is used to deserialize the JSON object.
HttpClient does not throw any error when HTTP response contains an error code, but it sets the IsSuccessStatusCode property to false. If we want to treat HTTP error codes as exceptions, we can use HttpResponseMessage.EnsureSuccessStatusCode method.
When we run the code, given above, it throws the exception "Internal Server error".
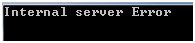
We have to configure the serializer to detect then handle self-referencing loops. The following code needs to be placed in Global.asax.cs in Web API:
GlobalConfiguration.Configuration.Formatters.JsonFormatter.SerializerSettings
.PreserveReferencesHandling =Newtonsoft.Json.PreserveReferencesHandling.All;
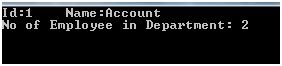
HTTP POST request
Following code is used to send a POST request for the department:
var department = newDepartment() { DepartmentName = "Test Department" };
HttpResponseMessage response = awaitclient.PostAsJsonAsync("api/Department", department);
if (response.IsSuccessStatusCode)
{
// Get the URI of the created resource.
UrireturnUrl = response.Headers.Location;
Console.WriteLine(returnUrl);
}
In this code, PostAsJsonAsync method serializes the object into JSON format and sends this JSON object in POST request. HttpClient has a built-in method "PostAsXmlAsync" to send XML in POST request. Also, we can use "PostAsync" for any other formatter.
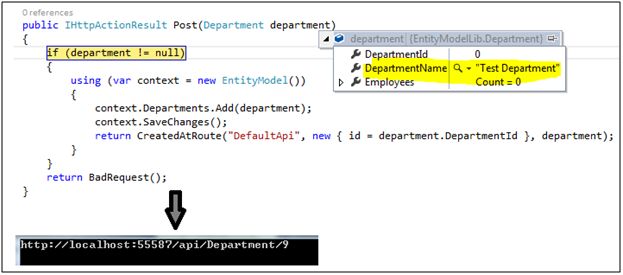
HTTP PUT Request
Following code is used to send a PUT request for the department:
//PUT Method
var department = newDepartment() { DepartmentId = 9, DepartmentName = "Updated Department" };
HttpResponseMessage response = awaitclient.PutAsJsonAsync("api/Department", department);
if (response.IsSuccessStatusCode)
{
Console.WriteLine("Success");
}
Same as POST, HttpClient also supports all three methods: PutAsJsonAsync, PutAsXmlAsync and PutAsync.
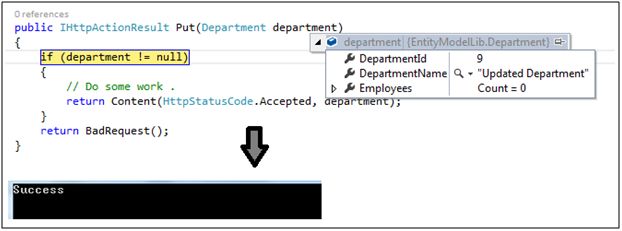
HTTP DELETE Request
Following code is used to send a DELETE request for the department:
intdepartmentId = 9;
HttpResponseMessage response = awaitclient.DeleteAsync("api/Department/" + departmentId);
if (response.IsSuccessStatusCode)
{
Console.WriteLine("Success");
}
HttpClient only supports DeleteAsync method because Delete method does not have a request body.
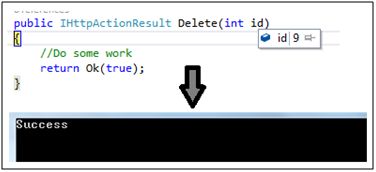
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
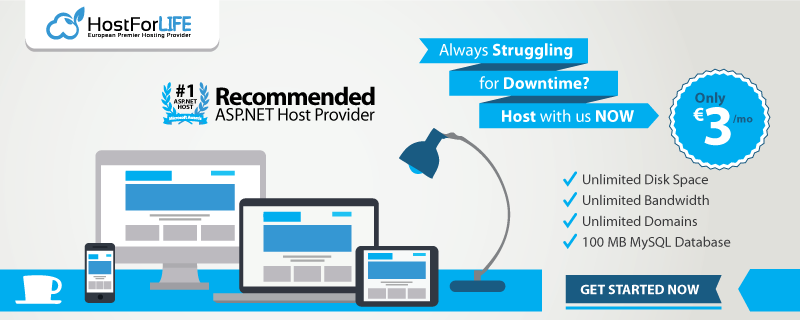