JSON is an open standard file format and data interchange format that uses human-readable text to store and transmit data objects consisting of attribute-value pairs and arrays.
About System.Text.Json
System.Text.Json library provides high-performance and low-allocating types that serialize objects to JavaScript Object Notation (JSON) text and deserialize JSON text to objects, with UTF-8 support built-in. Also provides types to read and write JSON text encoded as UTF-8, and to create an in-memory document object model (DOM), that is read-only, for random access of the JSON elements within a structured view of the data.
Now, let's install and use JSON library in the ASP.NET website.
Install System.Text.Json library from NuGet
To install library, go to "Microsoft Visual Studio 2022" => "Tools" menu => "NuGet Package Manager" => "Manage NuGet Packages for solution", search for "System.Text.Json" and click "Install" as in below picture.
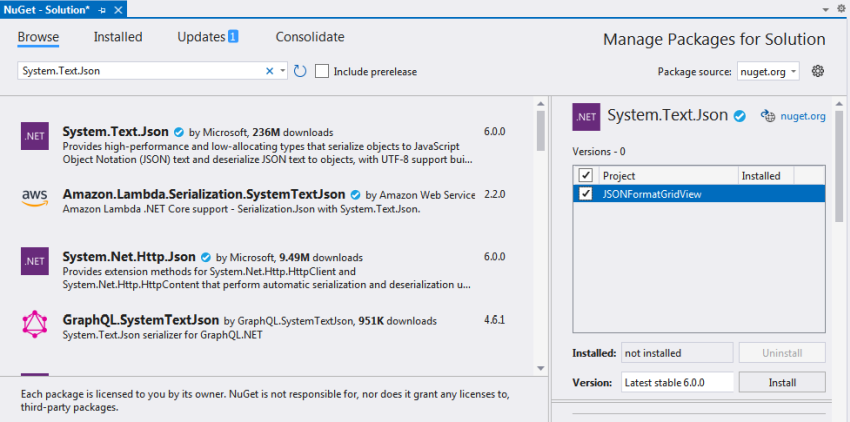
What is JSON Deserialization?
Deserialization is the process of parsing a string into an object of a specific type. The JsonSerializer.Deserialize() method converts a JSON string into an object of the type specified by a generic type parameter.
Below is the JSON format that will be used as an example:
string JsonString = @"{
""Version"": 1,
""Key"": ""314929"",
""Type"": ""City"",
""Rank"": 30,
""LocalizedName"": ""Stockholm"",
""EnglishName"": ""Stockholm"",
""PrimaryPostalCode"": """",
""Region"": {
""ID"": ""EUR"",
""LocalizedName"": ""Europe"",
""EnglishName"": ""Europe""
},
""Country"": {
""ID"": ""SE"",
""LocalizedName"": ""Sweden"",
""EnglishName"": ""Sweden""
},
""TimeZone"": {
""Code"": ""CET"",
""Name"": ""Europe/Stockholm"",
""GmtOffset"": 1.0,
""IsDaylightSaving"": false,
""NextOffsetChange"": ""2022-03-27T01:00:00Z""
},
""DataSets"": [
""AirQualityCurrentConditions"",
""AirQualityForecasts"",
""Alerts"",
""DailyPollenForecast"",
""ForecastConfidence"",
""FutureRadar"",
""MinuteCast"",
""Radar""
]
}";
And here are Class Properties for converting JSON string,
public class Cities {
public int Version {
get;
set;
}
public string Key {
get;
set;
}
public string Type {
get;
set;
}
public string EnglishName {
get;
set;
}
public Region Region {
get;
set;
}
public Country Country {
get;
set;
}
public TimeZone TimeZone {
get;
set;
}
public string[] DataSets {
get;
set;
}
}
public class Region {
public string ID {
get;
set;
}
public string LocalizedName {
get;
set;
}
public string EnglishName {
get;
set;
}
}
public class Country {
public string ID {
get;
set;
}
public string LocalizedName {
get;
set;
}
public string EnglishName {
get;
set;
}
}
public class TimeZone {
public string Code {
get;
set;
}
public string Name {
get;
set;
}
public float GmtOffset {
get;
set;
}
}
Now, the following is JsonSerializer.Deserialize() method to convert JSON string, which will be added to a datatable to use it in the GridView.
Cities cities = JsonSerializer.Deserialize<Cities>(JsonString);
int Version = cities.Version;
string Key = cities.Key;
string Type = cities.Type;
string City = cities.EnglishName;
string Region = cities.Region.EnglishName;
string Country = cities.Country.EnglishName;
string TimeZone = cities.TimeZone.Name;
string Alerts = cities.DataSets[2];
DataTable dt = new DataTable();
dt.Columns.Add("Version", typeof(string));
dt.Columns.Add("Key", typeof(string));
dt.Columns.Add("Type", typeof(string));
dt.Columns.Add("City", typeof(string));
dt.Columns.Add("Region", typeof(string));
dt.Columns.Add("Country", typeof(string));
dt.Columns.Add("TimeZone", typeof(string));
dt.Columns.Add("Alerts", typeof(string));
DataRow row;
row = dt.NewRow();
row["Version"] = Version;
row["Key"] = Key;
row["Type"] = Type;
row["City"] = City;
row["Region"] = Region;
row["Country"] = Country;
row["TimeZone"] = TimeZone;
row["Alerts"] = Alerts;
dt.Rows.Add(row);
GridView1.DataSource = dt;
GridView1.DataBind();
After running ASP.NET website, the following image is the result of JSON deserialization process.

In this article, System.Text.Json library was introduced, we saw how to install it and use it in an ASP.NET website. Also we discussed the steps to deserialize JSON string in an ASP.NET GridView. In conclusion, this was a demonstration of the concept of JSON deserialization and how System.Text.Json library helps achieve this process.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
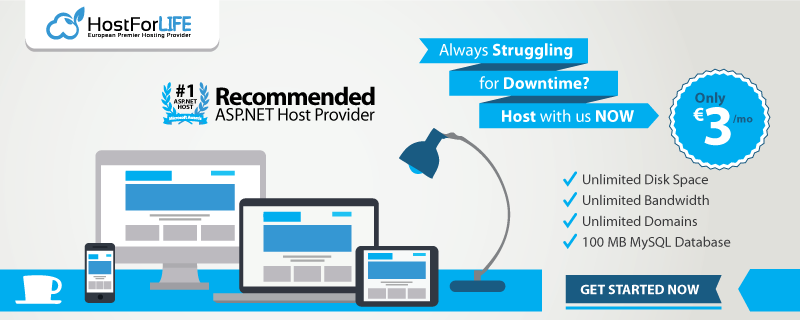