.Net core development is based on the open-source NuGet packages. NuGet will reduce work and manage the application very easily. We need to select the NuGet based on the purpose otherwise it will impact the application performance as well as application size also.
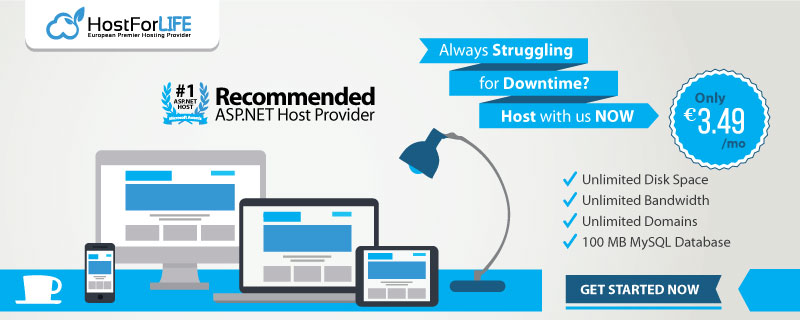
As part of the development lifecycle, we need to select different libraries at each stage.
While creating a new service, we need to inform what kind of APIs will be available and what fields will belong to that each APIs. It will provide high-level information about the service. So we need clear documentation at the first phase of development itself. We can create documentation either manually or code-wise. Manual documentation will double the work. In code wise we can generate the document by code and based on the needs we can update it later. We can create high-level API documentation using Swashbuckle at the initial stage of development.
Swashbuckle
We need to share the API specs while developing a restful API. Swashbuckle provides documentation for the API in JSON object format. The document contains the resource URL, request and response object, corresponding content format, and different status code types with the response object. It provides a built-in UI and loads the results into that. We can load the swagger YAML file into any open API application. It will be easy to understand and validate the API specs.
Once we have done the high level of documentation we need to start the API development. Each API will have a set of inputs. We need to validate the input before the processing logic. So we need to add a different set of validation rules based on the needs. We can add the validation to our API inputs using the Fluent validation library.
Fluent Validation
It contains .NET libraries and the validation is performed using the Lambda expression. We can add different types of custom validation to the input. We can build our custom validation rules based on the needs.
Once we have validated our inputs we need to store the data into a database based on our business. We need to convert the input model based on the data layer. So data conversion layer is required to map the user input to a data model. We can use AutoMapper to convert the user input objects to data objects and vice versa.
Automapper
It's a popular object-to-object mapping library that can be used to map objects belonging to dissimilar types. AutoMapper saves you the tedious effort of having to manually map one or more properties of such incompatible types.
Once data has been converted to data layer format we need to store the data into the database. So we need to establish the connection and form the query to run on the database. We need some mediators to establish a connection between the data context layer to the database. ORM serve this purpose using a different set of libraries
ORM
Data access is an important part of almost any software application. ASP.NET Core supports a variety of data access options and can work with any .NET data access framework. The choice of which data access framework to use depends on the application's needs. Dapper and Entity are used in most of the .net core apps. I have used both ORM into a single service based on the need to achieve better results in terms of performance and simplicity.
Once we have developed our APIs we need to check the application flow. We can identify the flows using logs. Logs will have information about the request, what will be the response for that request. Logs are mainly used to trace the application flows while debugging the errors. We can use ILogger to enable the logging mechanism to our services.
ILogger
It provides a set of features to store the application level logs to track the application flow. We can configure any logging providers like Serilog or Nlog and we can log any level of logs like information, warning, and errors. We can customize it based on our needs.
Based on the logs we can identify the root cause of errors. Sometimes due to network or data unavailability, the request will get failed. So we need to retry the request or retry after a while. It will increase the application's reliability while serving the request. We can enable the retry mechanism using the Polly library.
Polly
Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout in a fluent and thread-safe manner.
Service will serve the request as per the user's needs. After a while service might be get stopped due to some errors. We need to get the information once the service is stopped due to some errors. We need some kind of monitor to ensure our service is working as expected. .Net Core provides Diagnostics health checks libraries. Using this we can monitors service heartbeats at frequent intervals.
HealthChecks
Microservices-based applications often use heartbeats or health checks to enable their performance monitors, schedulers, and orchestrators to keep track of the multitude of services. Microsoft.AspNetCore.Diagnostics.HealthChecks package is referenced implicitly for ASP.NET Core apps
Once we have developed the API we need to test the new unit is working as expected. We shouldn't break the existing functions because of the new addition. So we need to test the unit of code before release to testing. We can do by adding unit test as part of development.
Unit Test
It's a type of software testing where individual units or components of the software are tested. The purpose is to validate that each unit of the software code performs as expected. Unit Testing is done during the development (coding phase) of an application by the developers. We can do the Unit testing by XUNIT or NUnit. I have used XUNIT for unit testing and open cover Nuget to generate the unit test reports.
Once we have developed our service we need to deploy it. Our service might be a web service or hosted service. While deploying multiple services it will be better to forward the request via a single endpoint using a gateway pattern. It will hide the request and forward it to internal services. We can implement API gateway using ocelot in .net core
Ocelot
It's a .NET API gateway library. It provides a single entry point to the system that is running multiple microservices and service-oriented services. It acts as a reverse proxy. Its supports HTTPS, HTTP, and WebSockets protocol. It provides gateway features like authentication, authorization, routing, query-string, and header transformation and aggregation. It's lightweight and provides better results while managing multiple services.
Some of the alternative libraries are available at each stage but we need to select them based on our needs. Choosing correct libraries will ease the development, increase the application performance and reduce the development time.