
May 25, 2021 07:10 by
Peter
In this article, you will learn how to send email using ASP.NET C# through the various SMTP servers. In this article, I also explained the way of sending emails from the HostForLIFE server.
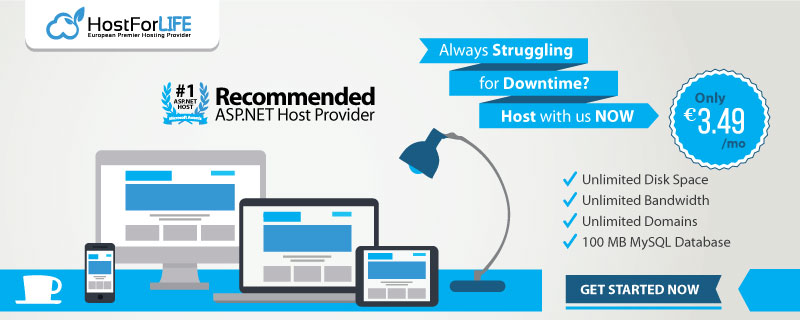
Below is the step to send an email using ASP.NET C#,
Step 1
Create a new website.
Step 2
Create a webform aspx page.
Step 3
Add CSS file as attached in source code.
Step 4
Add reference of AjaxToolKit as attached in source code.
Step 5
Design the webpage like below,
<!DOCTYPE html>
<html
xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Email Send Sample</title>
<link href="bootstrap.min.css" rel="stylesheet" />
</head>
<body>
<form id="form1" runat="server">
<div class="container">
<div class="row">
<div class="col-md-4 col-md-offset-4">
<div class="panel panel-primary">
<div class="panel-heading">
<h3 class="panel-title">Send Email Sample</h3>
</div>
<div class="panel-body">
<label>Name</label>
<asp:TextBox ID="txtname" runat="server" CssClass="form-control" placeholder="Name"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="Please Provide Name" ControlToValidate="txtname" ForeColor="Red"></asp:RequiredFieldValidator>
<br />
<label>Subject</label>
<asp:TextBox ID="txtbody" runat="server" CssClass="form-control" placeholder="Subject"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ErrorMessage="Please Provide Subject" ControlToValidate="txtbody" ForeColor="Red"></asp:RequiredFieldValidator>
<br />
<label>Email([email protected]</label>
<asp:TextBox ID="txtemail" runat="server" CssClass="form-control" placeholder="Email"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator4" runat="server" ErrorMessage="Please Provide Email-ID" ControlToValidate="txtemail" ForeColor="Red"></asp:RequiredFieldValidator>
<br />
<asp:RegularExpressionValidator ID="RegularExpressionValidator1" runat="server" ErrorMessage="Please Proide Valid Email-ID" ControlToValidate="txtemail" ForeColor="Red" ValidationExpression="\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"></asp:RegularExpressionValidator>
<br />
<asp:Button ID="Button1" runat="server" Text="Send Email" CssClass="btn btn-block btn-primary" OnClick="Button1_Click" />
<asp:Button ID="Button2" runat="server" Text="Send Email from HostForLIFE" CssClass="btn btn-block btn-primary" OnClick="Button2_Click" />
<asp:Label ID="lblmsg" runat="server" Text=""></asp:Label>
</div>
</div>
</div>
</div>
</div>
</form>
</body>
</html>
Step 6
Add Server-side validation for every field as name validation, email validation as written in the above webpage source code.
Step 7
Add Server-side RegularExpressionValidator to valid the correct email id.
Step 8
Added below namespace in .aspx.cs page,
using System.Net;
using System.Net.Mail;
Step 9
Add the below source code on button click event of the webpage.
Send email from local server,
string to = txtemail.Text; //To address
string from = "[email protected]"; //From address
MailMessage message = new MailMessage(from, to);
string mailbody = message.Subject = "This article will help you on how to send email using asp.net c# code";
message.Body = mailbody;
message.IsBodyHtml = false;
SmtpClient client = new SmtpClient();
client.Host = "smtp.gmail.com";
client.Port = 587;
System.Net.NetworkCredential basicCredential1 = new
System.Net.NetworkCredential("[email protected]", "password");
client.EnableSsl = false;
client.UseDefaultCredentials = false;
// client.Credentials = basicCredential1;
try {
client.Send(message);
lblmsg.Text = "Email Send Successfully";
} catch (Exception ex) {}
Send email from HostForLIFE Server,
string to = txtemail.Text; //To address
string from = "[email protected]"; //From address
MailMessage message = new MailMessage(from, to);
string mailbody = "This article will help you on how to send email using asp.net c# code";
message.Subject = txtbody.Text;
message.Body = mailbody;
message.IsBodyHtml = false;
SmtpClient client = new SmtpClient();
client.Host = "relay-hosting.secureserver.net"; //This is code SMTP Host server name
client.Port = 25; //This is default port
client.EnableSsl = false;
client.UseDefaultCredentials = false;
try {
client.Send(message);
lblmsg.Text = "Email Send Successfully";
} catch (Exception ex) {}
Step 10
Run the application.
If you get the below error then follow step 11.
WebForms UnobtrusiveValidationMode requires a ScriptResourceMapping for 'jquery'. Please add a ScriptResourceMapping named jquery(case-sensitive).
Step 11
Add the below code in web.config,
<appSettings>
<add key="ValidationSettings:UnobtrusiveValidationMode" value="None" />
</appSettings>
Step 12
Run the website and you will get the below screen. Fill in all the details and click on the button Send Email for Local server email like Gmail and Click on Send Email from HostForLIFE to send email from HostForLIFE server that will not work from local work only on the HostForLIFE server.
Step 13
Once you click on the button after filling in the data you get the message "Email Send Successfully".
Some of SMTP Class Properties,
Host
SMTP Server( Here you need to give the SMTP server name from where the email going to send).
Enable SSL
Check your host accepted SSL Connections Value is either True or False.
Port
SMTP Server Port (Here you need to give SMTP server port from where the email going to send).
Credentials
SMTP servers login credentials such as Email and Password.
UseDefaultCredentials
When we set to True in UseDefaultCredentials then that specifies to allow authentication based on the credentials of the account used to send emails.
In this article, we learned how to send email using ASP.NET C# through the SMTP server. I hope this article is useful for those who want to send email using ASP.NET C#.