
June 7, 2021 08:26 by
Peter
If there was a poll to find that one little feature every C# developer would love to have in .Net, then it is most likely the ability to store Date and Time individually. For years now, we had to use DateTime to represent Date when the Time part of the object had to be ignored. The same thing happened when we wanted to save time and had to ignore the Date of the DateTime component.
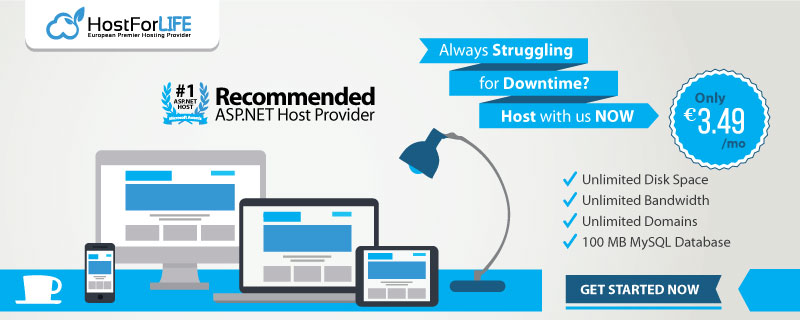
And with .Net 6, that long agonizing wait has come to an end. .Net 6 has now introduced DateOnly and TimeOnly Structures which could store Date and Time components.
DateOnly
The DateOnly structure allows you to save a Date property with a more explicit structure rather than using the DateTime and ignoring the Time part.
var dateOnlyFirst = new DateOnly(2020, 2, 16);
var dateOnlySecond = DateOnly.FromDateTime(DateTime.Now);
Console.WriteLine(dateOnlyFirst);
Console.WriteLine(dateOnlySecond);
// Output
16-02-2020
04-06-2021
We could create the instance either using the Constructors or static helper methods like DateOnly.FromDateTime. It is also interesting to know what happens behinds the scenes. Internally, the DateOnly structure stores the value in an Int32 field that represents the number of days since 1/1/0001. The field is designed to store values from 1 Jan,0001 to 31st Dec 9999. If you take a glimpse of code in the .Net Github source code for DateOnly, you could find some of the following,
private readonly int _dayNumber;
// Maps to Jan 1st year 1
private const int MinDayNumber = 0;
// Maps to December 31 year 9999. The value calculated from "new DateTime(9999, 12, 31).Ticks / TimeSpan.TicksPerDay"
private const int MaxDayNumber = 3_652_058;
public DateOnly(int year, int month, int day) => _dayNumber = DayNumberFromDateTime(new DateTime(year, month, day));
public static DateOnly FromDateTime(DateTime dateTime) => new DateOnly(DayNumberFromDateTime(dateTime));
private static int DayNumberFromDateTime(DateTime dt) => (int)(dt.Ticks / TimeSpan.TicksPerDay);
The DateOnly provides most of the (applicable) functionality that DateTime provides including AddDays(),AddMonths(),AddYears, ParseExact and many more.
TimeOnly
TimeOnly Quite similar to DateOnly, the TimeOnly structure provides a way to represent the Time component effectively in .Net.
var timeOnlyFirst = new TimeOnly(10,10,10);
var timeOnlySecond = TimeOnly.FromDateTime(DateTime.Now);
Console.WriteLine(timeOnlyFirst);
Console.WriteLine(timeOnlySecond);
//Output
10:10
05:35
Internally, TimeOnly uses a long to represent the ticks elapsed since midnight. Let us know to take a glimpse of the internal code of TimeOnly.
// represent the number of ticks map to the time of the day. 1 ticks = 100-nanosecond in time measurements.
private readonly long _ticks;
// MinTimeTicks is the ticks for the midnight time 00:00:00.000 AM
private const long MinTimeTicks = 0;
// MaxTimeTicks is the max tick value for the time in the day. It is calculated using DateTime.Today.AddTicks(-1).TimeOfDay.Ticks.
private const long MaxTimeTicks = 863_999_999_999;
public TimeOnly(int hour, int minute, int second) : this(DateTime.TimeToTicks(hour, minute, second, 0)) {}
public TimeOnly(long ticks)
{
if ((ulong)ticks > MaxTimeTicks)
{
throw new ArgumentOutOfRangeException(nameof(ticks), SR.ArgumentOutOfRange_TimeOnlyBadTicks);
}
_ticks = ticks;
}
As with the DateOnly structure, the TimeOnly too comes in with a lot of helper methods that would aid the developer.
It is quite safe to assume that a lot of developers would be quite happy with the introduction of these structures. These would definitely improve the code base a lot and make the representations more explicit, rather than using DateTime and ignoring a part of it.