If we have made a Restful API utilizing the ASP.NET Core 2.2.1 Web API and if your API is in one space and the UI is in an alternate area then you may get problem because of cross-domain issues. And here is the way:
- OPTIONS http://localhost:5000/api/ 404 (Not Found).XMLHttpRequest cannot load http://localhost:5000/api/.
- No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:54317' is therefore not allowed access. The response had HTTP status code 404.
As it were you can't make a call to the WebAPI by means of your front end that is facilitated on an alternate domain.
So you must utilization JSONP to get information utilizing using AJAX, not by JSON. To attain to this objective you have to introduce JsonpMediaTypeFormatter. For introducing the JsonpMediaTypeFormatter in Visual Studio, seek JsonpMediaTypeFormatter in "Manage NuGet Packages" and install it.
Next, you can see in your project references that a new DLL was added. WebApiContrib.Formatting.Jsonp.dll
To use this DLL you need to change in the Application_Start() method in the Global.asax.cs file.
using WebApiContrib.Formatting.Jsonp;
using System.Net.Http.Formatting;
GlobalConfiguration.Configuration.Formatters.Clear(); GlobalConfiguration.Configuration.Formatters.Add(new JsonpMediaTypeFormatter(new JsonMediaTypeFormatter()));
You also can use JsonMediaTypeFormatter code, you should write this code in the App_Start folder & register the following code in Application_Start().
JsonMediaTypeFormatter code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Net.Http.Formatting;
using System.Net.Http.Headers;
using System.Net.Http;
using Newtonsoft.Json.Converters;
using System.IO;
using System.Net;
using System.Threading.Tasks;
namespace WebAPI
{
/// <summary>
/// Handles JsonP requests when requests are fired with text/javascript
/// </summary>
public class JsonpFormatter : JsonMediaTypeFormatter
{
public JsonpFormatter()
{
SupportedMediaTypes.Add(new MediaTypeHeaderValue("application/json"));
SupportedMediaTypes.Add(new MediaTypeHeaderValue("text/javascript"));
JsonpParameterName = "callback";
}
/// <summary>
/// Name of the query string parameter to look for
/// the jsonp function name
/// </summary>
public string JsonpParameterName { get; set; }
/// <summary>
/// Captured name of the Jsonp function that the JSON call
/// is wrapped in. Set in GetPerRequestFormatter Instance
/// </summary>
private string JsonpCallbackFunction;
public override bool CanWriteType(Type type)
{
return true;
}
/// <summary>
/// Override this method to capture the Request object
/// </summary>
/// <param name="type"></param>
/// <param name="request"></param>
/// <param name="mediaType"></param>
/// <returns></returns>
public override MediaTypeFormatter GetPerRequestFormatterInstance(Type type, HttpRequestMessage request, MediaTypeHeaderValue mediaType)
{
var formatter = new JsonpFormatter()
{
JsonpCallbackFunction = GetJsonCallbackFunction(request)
};
// this doesn't work unfortunately
//formatter.SerializerSettings = GlobalConfiguration.Configuration.Formatters.JsonFormatter.SerializerSettings;
// You have to reapply any JSON.NET default serializer Customizations here
formatter.SerializerSettings.Converters.Add(new StringEnumConverter());
formatter.SerializerSettings.Formatting = Newtonsoft.Json.Formatting.Indented;
return formatter;
}
public override Task WriteToStreamAsync(Type type, object value,
Stream stream,
HttpContent content,
TransportContext transportContext)
{
if (string.IsNullOrEmpty(JsonpCallbackFunction))
return base.WriteToStreamAsync(type, value, stream, content, transportContext);
StreamWriter writer = null;
// write the pre-amble
try
{
writer = new StreamWriter(stream);
writer.Write(JsonpCallbackFunction + "(");
writer.Flush();
}
catch (Exception ex)
{
try
{
if (writer != null)
writer.Dispose();
}
catch { }
var tcs = new TaskCompletionSource<object>();
tcs.SetException(ex);
return tcs.Task;
}
return base.WriteToStreamAsync(type, value, stream, content, transportContext)
.ContinueWith(innerTask =>
{
if (innerTask.Status == TaskStatus.RanToCompletion)
{
writer.Write(")");
writer.Flush();
}
writer.Dispose();
return innerTask;
}, TaskContinuationOptions.ExecuteSynchronously)
.Unwrap();
}
/// <summary>
/// Retrieves the Jsonp Callback function
/// from the query string
/// </summary>
/// <returns></returns>
private string GetJsonCallbackFunction(HttpRequestMessage request)
{
if (request.Method != HttpMethod.Get)
return null;
var query = HttpUtility.ParseQueryString(request.RequestUri.Query);
var queryVal = query[this.JsonpParameterName];
if (string.IsNullOrEmpty(queryVal))
return null;
return queryVal;
}
}
}
Next step, use the following code to make change in the Application_Start() method in the Global.asax.cs file.
GlobalConfiguration.Configuration.Formatters.Insert(0, new WebAPI.JsonpFormatter());
jQuery code to use the Web API:
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script language="javascript" type="text/javascript">
function GetInformation() {
var apiServicePath = "http://localhost:5000/api/";
jQuery.ajax({
crossDomain: true,
dataType: "jsonp",
url: apiServicePath + "test/GetInformation",
async: false,
context: document.body
}).done(function (data) {
alert("Done");
});
};
</script>
HostForLIFE.eu ASP.NET Core 2.2.1 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
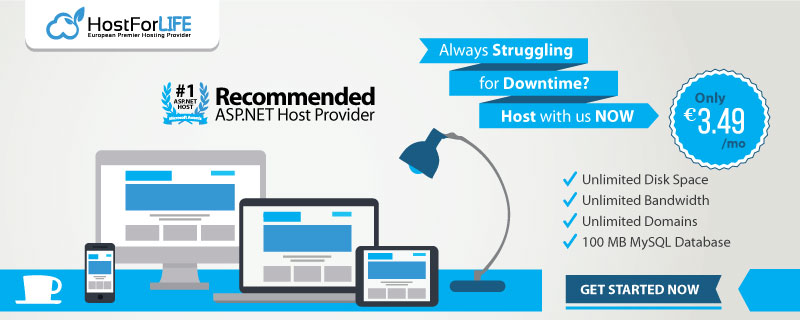