As the world of microservices evolved, so did the strategy and technologies that surrounded it. Communication across multiple services is one of the most critical components of microservices. Remote procedure calls are one method of communication that is employed, and gRPC provides a complex framework for it.
As the acronym suggests, gRPC stands for Google remote procedure call. It was the framework that Google utilized before opening it out to the open-source community. It employs the HTTP2 protocol.
It has a few advantages, such as.
- Messages that are brief
- It is quick
- It supports full-duplex communication
gRPC is generally used for internal service communication, however it does support web gRPC, which may be accessed via browsers.
Unlike RESTful services, the service signature and message property must be known by both the client and the server.
It offers versatility. Both the client and the server can be constructed in various technology stacks and still communicate effectively.
Now, begin building gRPC in.Net Core, but note that the.Net API project can have both Rest API and gRPC services, and in this tutorial, we will add gRPC to the already existing
RestFull API Project
For Rest API, I use Mediator and CQRS architecture, but you can use any technique.
Step 1: Make a Prototype file.

Now, define the RPC Service structure.
service EventCatalogService {
rpc GetAllCategories(GetCategoriesRequest) returns (CategoriesResponse);
}
As we can see, the Name is GetAllCategories, the request it is taking is GetCategoriesRequest, and the Response is sending CategoriesResponse.
Now, Create the request and response messages for services.
message GetCategoriesRequest
{
}
message CategoriesResponse {
repeated CategoryItem Categories = 1;
}
message CategoryItem {
string CategoryId = 1;
string Name = 2;
}
The repeated is defining the collection here.
You can see the number against each attribute defining the position of the attribute in the message. We can also reserve some position for future use.
syntax = "proto3";
option csharp_namespace = "EventCatalog.gRPC";
import "google/protobuf/timestamp.proto";
service EventCatalogService {
rpc GetAllCategories(GetCategoriesRequest) returns (CategoriesResponse);
}
message GetCategoriesRequest {}
message EventByCategoryIdRequest {
string CategoryId = 1;
}
message EventByEventIdRequest {
string EventId = 1;
}
message EventByCategoryIdResponse {
string EventId = 1;
string Name = 2;
int32 Price = 3;
string Artist = 4;
google.protobuf.Timestamp Date = 5;
string Description = 6;
string ImageUrl = 7;
string CategoryId = 8;
string CategoryName = 9;
}
message EventByEventIdResponse {
string EventId = 1;
string Name = 2;
int32 Price = 3;
string Artist = 4;
google.protobuf.Timestamp Date = 5;
string Description = 6;
string ImageUrl = 7;
string CategoryId = 8;
string CategoryName = 9;
}
message CategoriesResponse {
repeated CategoryItem Categories = 1;
}
message CategoryItem {
string CategoryId = 1;
string Name = 2;
}
The proto file is ready.
Step 2. Add gRPC to your .Net project
Add the proto file as a connected service.
Click on project connected service.
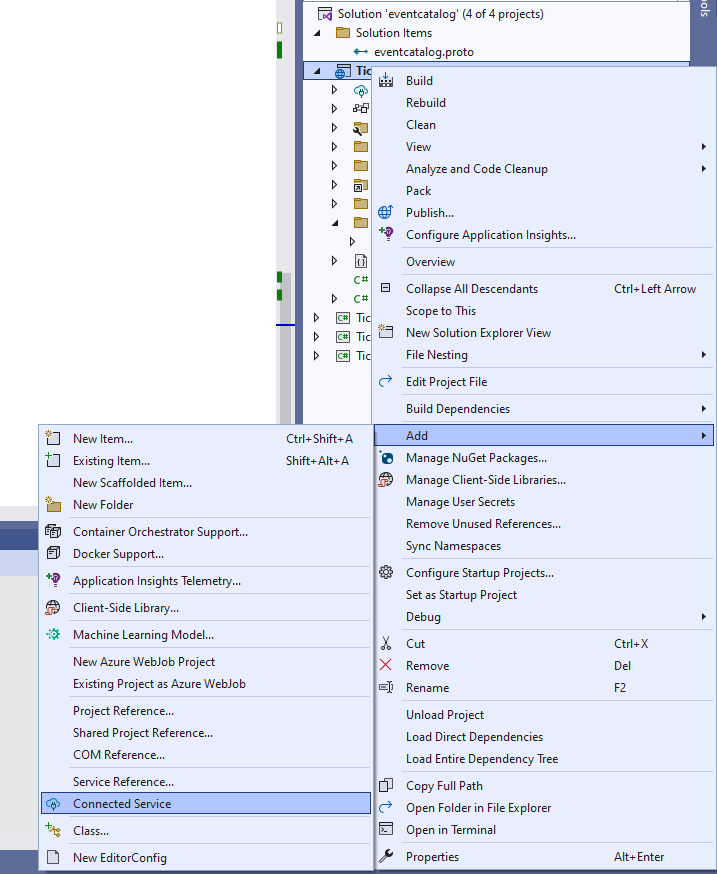
Now, create the grpc server by giving the proto file path.
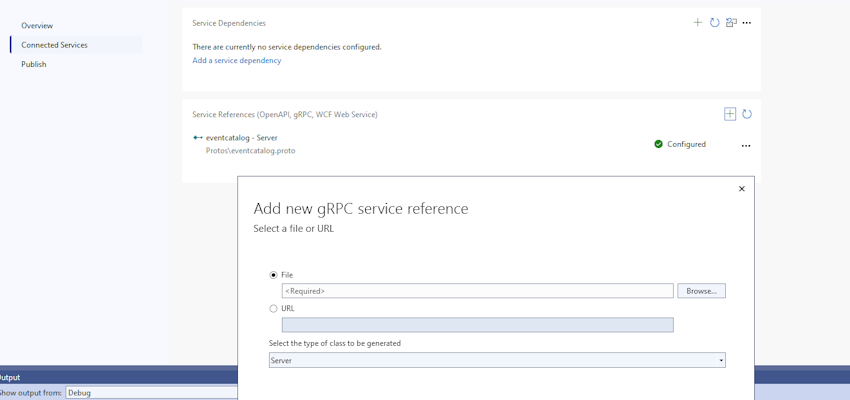
It will include the proto file as well as other necessary Nuget packages in the project.
Step 3: Add the gRPC to your service layer
First, establish the gRPC service that will inherit the basic class of gRPC service, and then integrate your business layer into it. Because I'm using mediatr, I'll get the message from the gRPC service, pass it to my application layer, and then provide a response.
using EventCatalog.gRPC;
using FOA.Application.Mapper;
using Grpc.Core;
using MediatR;
using TicketManagement.Services.EventCatalog.Application.Feature.Category.Queries;
using static EventCatalog.gRPC.EventCatalogService;
namespace TicketManagement.Services.EventCatalog.RPCServices;
public class EventCatalogRPCService : EventCatalogServiceBase
{
private readonly IMediator _mediator;
public EventCatalogRPCService(IMediator mediator)
{
_mediator = mediator;
}
public override async Task<CategoriesResponse> GetAllCategories(GetCategoriesRequest request,ServerCallContext context)
{
var serviceResult = await _mediator.Send(new GetAllCategoriesRequest());
var result = gRPCMappers.Mapper.Map<List<CategoryItem>>(serviceResult);
var response = new CategoriesResponse();
response.Categories.AddRange(result);
return response;
}
}
Step 5. Add gRPC to your program.cs
// adding grpc
builder.Services.AddGrpc(cfg => cfg.EnableDetailedErrors = true);
After build, add the following line.
app.MapGrpcService<EventCatalogRPCService>();
Now the server is ready, we need a client to test it.
Step 6. Create gRPC Client
Create a new .Net API project and the Client gRPC through the connected service, but this time, instead of the server, select client.
As the client is only going to send and receive messages from the server, there will be not much code other than basic communication.
var channel = GrpcChannel.ForAddress(_serviceURL);
var client = new EventCatalogServiceClient(channel);
var request = new GetCategoriesRequest();
var response = client.GetAllCategories(request);
It is creating a channel through the service URL, which is the URL of our gRPC Server, and then creating a client through that channel and calling the service through that client.
Step 7. Add authorization to your service
So, adding security to your gRPC service is very easy. As the gRPC is added as a middleware to your application, the already implemented authentication or Authorization is already available to you.
You just need to add an Authorization tag to your service.
[Authorize]
public override async Task<CategoriesResponse> GetAllCategories(GetCategoriesRequest request,ServerCallContext context)
{
var serviceResult = await _mediator.Send(new GetAllCategoriesRequest());
var result = gRPCMappers.Mapper.Map<List<CategoryItem>>(serviceResult);
var response = new CategoriesResponse();
response.Categories.AddRange(result);
return response;
}
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
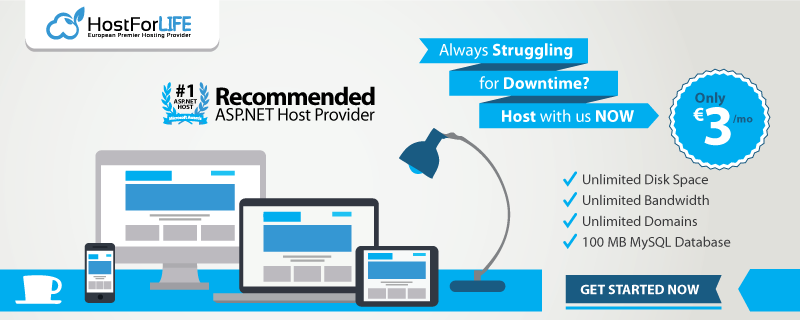