One of the common issues with events and event handlers is memory leaks. In applications, it is possible that handlers attached to event sources will not be destroyed if we forget to unregister the handlers from the event. This situation can lead to memory leaks. .Net 4.5 introduces a weak event pattern that can be used to address this issue.
Problem: Without using weak event pattern.
Let's understand the problem with an example. If we understand the problem then the solution is very easy.
public class EventSource
{
public event EventHandler<EventArgs> Event = delegate { };
public void Raise()
{
Event(this, EventArgs.Empty);
}
}
public class EventListener
{
public int Counter { get; set; }
EventSource eventSourceObject;
public EventListener(EventSource source)
{
eventSourceObject= source;
eventSourceObject.Event += MyHandler;
}
private void MyHandler(object source, EventArgs args)
{
Counter++;
Console.WriteLine("MyHandler received event "+ Counter);
}
public void UnRegisterEvent()
{
eventSourceObject.Event -= MyHandler;
}
}
static void Main(string[] args)
{
EventSource source = new EventSource();
EventListener listener = new EventListener(source);
Console.WriteLine("Raise event first time");
Console.Read();
source.Raise();
//Set listener to null and call GC to release the
//listener object from memory.
Console.WriteLine("\nSet listener to null and call GC");
Console.Read();
//developer forget to unregister the event
//listener.UnRegisterEvent();
listener = null;
GC.Collect();
Console.WriteLine("\nRaise event 2nd time");
Console.Read();
source.Raise();
Console.ReadKey();
}
Output
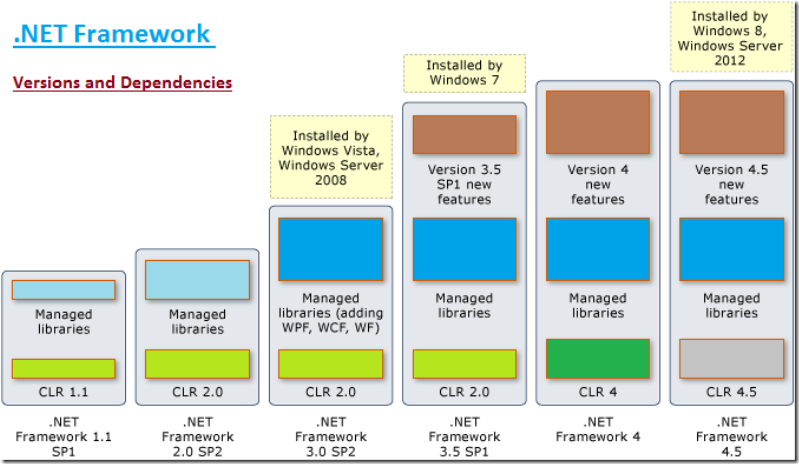
The developer is expecting that set listener = null is sufficient to release the object but this is not the case. The event listener object is still in memory.
the EventListener's handler should be called a second time. But it's calling.
In this example the developer forget to unregister the event. With this mistake, the listener object is not released from memory and it's still active. If we call the unregister method then the EventListener would be released from memory. But the developer does not remember when to unregister the event.
This is the problem!
Solution: Weak event pattern.
The weak event pattern is designed to solve this memory leak problem. The weak event pattern can be used whenever a listener needs to register for an event, but the listener does not explicitly know when to unregister.
In the old way of attaching the event with a source it is tightly coupled.
eventSourceObject.Event += MyHandler;
Using Weak event pattern
Replace the preceding registration with the following code and run the program.
WeakEventManager<EventSource, EventArgs>.AddHandler(source, "Event", MyHandler);
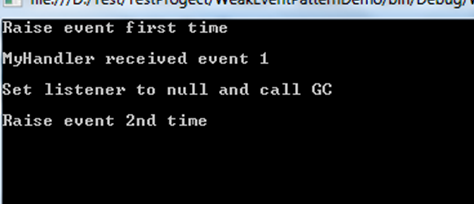
After raising the event a second time, the listener is doing nothing. It means the listener is no longer in memory.
Now the developer need not be concerned about calling the unregister event.
For any specific scenario if we want to unregister the event then we can use the RemoveHandler method as in the following:
WeakEventManager<EventSource, EventArgs>. RemoveHandler(source, "Event", MyHandler);