This article shows how to use MySQL with ASP.NET Core 2.1 using Entity Framework Core.
The Entity Framework MySQL package can be downloaded using the NuGet package Pomelo.EntityFrameworkCore.MySql. At present no official provider from MySQL exists for Entity Framework Core which can be used in an ASP.NET Core application.
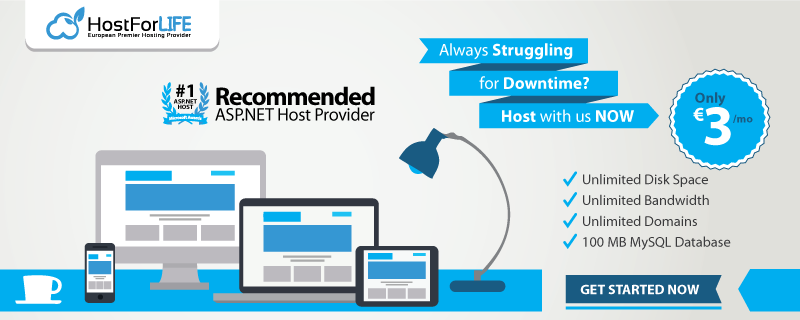
The Pomelo.EntityFrameworkCore.MySql package can be added to the csproj file.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netcoreapp2.2</TargetFramework>
<AssemblyName>DataAccessMySqlProvider</AssemblyName>
<PackageId>DataAccessMySqlProvider</PackageId>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include="..\DomainModel\DomainModel.csproj" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.2.0" PrivateAssets="All" />
<PackageReference Include="Pomelo.EntityFrameworkCore.MySql" Version="2.0.1" />
</ItemGroup>
<ItemGroup>
<Folder Include="Properties\" />
</ItemGroup>
</Project>
The web project which loads the project with EF Core needs to support migrations if you wish to create a database this way.
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>netcoreapp2.1</TargetFramework>
<AssemblyName>AspNetCoreMultipleProject</AssemblyName>
<PackageId>AspNet5MultipleProject</PackageId>
</PropertyGroup>
<ItemGroup>
<Content Update="wwwroot\**\*;Views;Areas\**\Views;appsettings.json;config.json;web.config">
<CopyToPublishDirectory>PreserveNewest</CopyToPublishDirectory>
</Content>
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\DataAccessMsSqlServerProvider\DataAccessMsSqlServerProvider.csproj" />
<ProjectReference Include="..\DataAccessMySqlProvider\DataAccessMySqlProvider.csproj" />
<ProjectReference Include="..\DataAccessPostgreSqlProvider\DataAccessPostgreSqlProvider.csproj"
/>
<ProjectReference Include="..\DataAccessSqliteProvider\DataAccessSqliteProvider.csproj" />
<ProjectReference Include="..\DomainModel\DomainModel.csproj" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.2.0" PrivateAssets="All" />
</ItemGroup>
<ItemGroup>
<DotNetCliToolReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Tools" Version="2.0.0" />
</ItemGroup>
<ItemGroup>
<Folder Include="Migrations\" />
</ItemGroup>
</Project>
An EfCore DbContext can be added like any other context supported by Entity Framework Core.
using System;
using System.Linq;
using DomainModel.Model;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
namespace DataAccessMySqlProvider
{
// >dotnet ef migration add testMigration
public class DomainModelMySqlContext : DbContext
{
public DomainModelMySqlContext(DbContextOptions<DomainModelMySqlContext> options) :base(options)
{ }
public DbSet<DataEventRecord> DataEventRecords { get; set; }
public DbSet<SourceInfo> SourceInfos { get; set; }
protected override void OnModelCreating(ModelBuilder builder)
{
builder.Entity<DataEventRecord>().HasKey(m => m.DataEventRecordId);
builder.Entity<SourceInfo>().HasKey(m => m.SourceInfoId);
// shadow properties
builder.Entity<DataEventRecord>().Property<DateTime>("UpdatedTimestamp");
builder.Entity<SourceInfo>().Property<DateTime>("UpdatedTimestamp");
base.OnModelCreating(builder);
}
public override int SaveChanges()
{
ChangeTracker.DetectChanges();
updateUpdatedProperty<SourceInfo>();
updateUpdatedProperty<DataEventRecord>();
return base.SaveChanges();
}
private void updateUpdatedProperty<T>() where T : class
{
var modifiedSourceInfo =
ChangeTracker.Entries<T>()
.Where(e => e.State == EntityState.Added || e.State == EntityState.Modified);
foreach (var entry in modifiedSourceInfo)
{
entry.Property("UpdatedTimestamp").CurrentValue = DateTime.UtcNow;
}
}
}
}
In an ASP.NET Core web application, the DbContext is added to the application in the startup class. In this example, the DbContext is defined in a different class library. The MigrationsAssembly needs to be defined, so that the migrations will work. If the context and the migrations are defined in the same assembly, this is not required.
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true)
.AddJsonFile("config.json", optional: true, reloadOnChange: true);
Configuration = builder.Build();
}
public void ConfigureServices(IServiceCollection services)
{
var sqlConnectionString = Configuration.GetConnectionString("DataAccessMySqlProvider");
services.AddDbContext<DomainModelMySqlContext>(options =>
options.UseMySQL(
sqlConnectionString,
b => b.MigrationsAssembly("AspNetCoreMultipleProject")
)
);
}
The application uses the configuration from the config.json. This file is used to get the MySQL connection string, which is used in the Startup class.
{
"ConnectionStrings": {
"DataAccessMySqlProvider": "server=localhost;userid=store;password=3333;database=store;"
}
}
}
MySQL workbench can be used to add the schema ‘store to the MySQL database. The user ‘store is also required, which must match the defined user in the connection string. If you configure the MySQL database differently, then you need to change the connection string in the config.json file.
Now the database migrations can be created and the database can be updated.
>
> dotnet ef migrations add mySqlMigration --context DomainModelMySqlContext
>
> dotnet ef database update --context DomainModelMySqlContext
>
If successful, the tables are created.
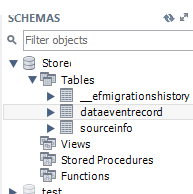
The MySQL provider can be used in a MVC controller using construction injection.
using System.Collections.Generic;
using DomainModel;
using DomainModel.Model;
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
namespace AspNet5MultipleProject.Controllers
{
[Route("api/[controller]")]
public class DataEventRecordsController : Controller
{
private readonly IDataAccessProvider _dataAccessProvider;
public DataEventRecordsController(IDataAccessProvider dataAccessProvider)
{
_dataAccessProvider = dataAccessProvider;
}
[HttpGet]
public IEnumerable<DataEventRecord> Get()
{
return _dataAccessProvider.GetDataEventRecords();
}
[HttpGet]
[Route("SourceInfos")]
public IEnumerable<SourceInfo> GetSourceInfos(bool withChildren)
{
return _dataAccessProvider.GetSourceInfos(withChildren);
}
[HttpGet("{id}")]
public DataEventRecord Get(long id)
{
return _dataAccessProvider.GetDataEventRecord(id);
}
[HttpPost]
public void Post([FromBody]DataEventRecord value)
{
_dataAccessProvider.AddDataEventRecord(value);
}
[HttpPut("{id}")]
public void Put(long id, [FromBody]DataEventRecord value)
{
_dataAccessProvider.UpdateDataEventRecord(id, value);
}
[HttpDelete("{id}")]
public void Delete(long id)
{
_dataAccessProvider.DeleteDataEventRecord(id);
}
}
}
The controller api can be called using Fiddler:
POST http://localhost:5000/api/dataeventrecords HTTP/1.1
User-Agent: Fiddler
Host: localhost:5000
Content-Length: 135
Content-Type: application/json;
{
"DataEventRecordId":3,
"Name":"Funny data",
"Description":"yes",
"Timestamp":"2015-12-27T08:31:35Z",
"SourceInfo":
{
"SourceInfoId":0,
"Name":"Beauty",
"Description":"second Source",
"Timestamp":"2015-12-23T08:31:35+01:00",
"DataEventRecords":[]
},
"SourceInfoId":0
}
The data is added to the database as required.
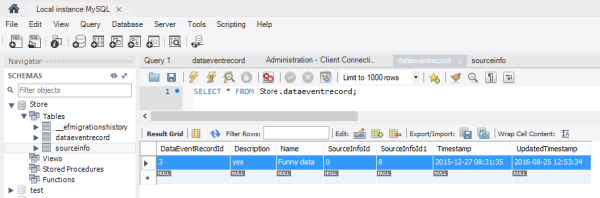