
March 5, 2024 06:32 by
Peter
Data structures are at the foundation of software development, determining how information is organized, stored, and manipulated within applications. Developers in the.NET environment have access to a wide range of data structures that allow for efficient data management, resulting in strong and scalable software solutions. In this post, we will look at some key.NET data structures with practical examples, providing light on their uses and benefits.
1. Lists (.NET Dynamic Arrays)
Lists are dynamic arrays in.NET that allow for size flexibility and easy manipulation. Consider the following scenario: we need to keep track of the names of all our students.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> students = new List<string>();
// Adding students to the list
students.Add("Alice");
students.Add("Bob");
students.Add("Charlie");
// Iterating over the list
foreach (var student in students)
{
Console.WriteLine(student);
}
}
}
2. Queues (FIFO Principle)
Queues follow the First-In-First-Out (FIFO) principle, commonly used in task scheduling or message processing scenarios. Let's simulate a simple printing queue.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Queue<string> printQueue = new Queue<string>();
// Enqueueing documents to be printed
printQueue.Enqueue("Document1");
printQueue.Enqueue("Document2");
printQueue.Enqueue("Document3");
// Printing documents in the order they were added
while (printQueue.Count > 0)
{
string document = printQueue.Dequeue();
Console.WriteLine("Printing: " + document);
}
}
}
3. Stacks (LIFO Principle)
Stacks adhere to the Last-In-First-Out (LIFO) principle and are commonly used in situations like expression evaluation or browser history. Let's implement a simple browser history using a stack.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Stack<string> browserHistory = new Stack<string>();
// Navigating through web pages
browserHistory.Push("Homepage");
browserHistory.Push("About");
browserHistory.Push("Contact");
// Navigating back through history
while (browserHistory.Count > 0)
{
string currentPage = browserHistory.Pop();
Console.WriteLine("Current Page: " + currentPage);
}
}
}
4. Dictionaries (Key-Value Pairs)
Dictionaries in .NET store key-value pairs, enabling efficient lookup and retrieval based on keys. Let's create a simple dictionary to store the ages of individuals.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
Dictionary<string, int> ages = new Dictionary<string, int>();
// Adding individuals and their ages
ages["Alice"] = 25;
ages["Bob"] = 30;
ages["Charlie"] = 35;
// Retrieving ages
Console.WriteLine("Bob's age: " + ages["Bob"]);
}
}
Conclusion
Understanding and utilizing.NET data structures is critical for effective program development. Developers can create more robust and scalable apps by learning about these data structures and their uses through real examples. Whether you're managing collections, creating algorithms, or improving performance, a good understanding of.NET data structures gives you the confidence to take on a variety of programming issues.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
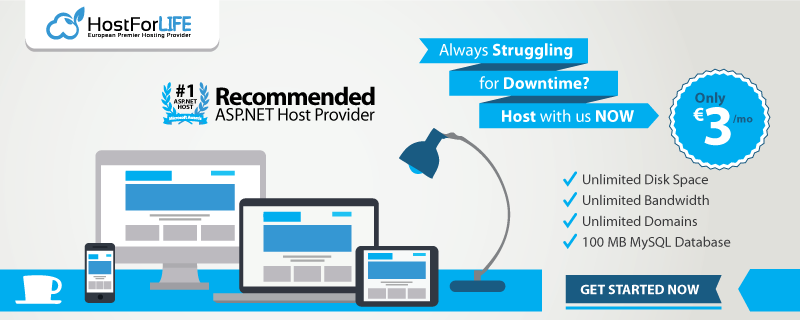