
March 8, 2024 07:34 by
Peter
Rate limitation is an important feature of web application security and performance management since it helps to prevent misuse and guarantee that resources are used fairly. In ASP.NET Core, rate restriction can be implemented using middleware, which provides a centralized way for controlling the rate of incoming requests. This blog discusses rate-limiting middleware, its implementation in ASP.NET Core, and its importance in online application development.
What is Rate Limiting?
Rate limitation is a mechanism that limits the amount of requests a client can make to a web server during a given time period. It aids in the prevention of misuse, protects against denial-of-service (DoS) assaults, and ensures equal resource access.
Rate-Limiting Middleware for ASP.NET Core
The rate-limiting middleware in ASP.NET Core intercepts incoming requests and enforces rate limitations based on predefined constraints. It lies between the client and the application, monitoring request rates and returning appropriate HTTP status codes when limitations are reached.
1. Install Required Packages
Install the AspNetCoreRateLimit package from NuGet:
dotnet add package AspNetCoreRateLimit
2. Configure Rate-Limiting Middleware
In the Startup.cs file, add the rate-limiting middleware to the request processing pipeline:
using AspNetCoreRateLimit;
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
// Other middleware configurations
app.UseIpRateLimiting();
app.UseClientRateLimiting();
}
3. Configure Rate-Limiting Options
Configure rate-limiting options in the appsettings.json file:
{
"IpRateLimiting": {
"EnableEndpointRateLimiting": true,
"StackBlockedRequests": true,
"RealIpHeader": "X-Real-IP",
"HttpStatusCode": 429,
"QuotaExceededResponse": {
"Content-Type": "application/json",
"Content": "{\"error\": \"Rate limit exceeded\"}"
},
"GeneralRules": [
{
"Endpoint": "*",
"Period": "1s",
"Limit": 5
}
]
},
"ClientRateLimiting": {
"EnableEndpointRateLimiting": true,
"StackBlockedRequests": true,
"HttpStatusCode": 429,
"QuotaExceededResponse": {
"Content-Type": "application/json",
"Content": "{\"error\": \"Rate limit exceeded\"}"
},
"GeneralRules": [
{
"Endpoint": "*",
"Period": "1s",
"Limit": 100
}
]
}
}
4. Test Rate Limiting
Test the rate-limiting middleware by sending requests to your ASP.NET Core application and observing the behavior when rate limits are exceeded.
Rate-limiting middleware in ASP.NET Core is a useful tool for controlling request rates and protecting online applications from misuse and overload. Rate limitation allows developers to improve the security, stability, and performance of their ASP.NET Core apps while also assuring fair and equitable access to resources for all users. Accept rate restriction as an essential component of web application development to protect your apps from malicious actions and resource depletion threats.
Conclusion
Rate-limiting middleware in ASP.NET Core is a useful tool for controlling request rates and protecting online applications from misuse and overload. Rate limitation allows developers to improve the security, stability, and performance of their ASP.NET Core apps while also assuring fair and equitable access to resources for all users. Accept rate restriction as an essential component of web application development to protect your apps from malicious actions and resource depletion threats.
Happy coding!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
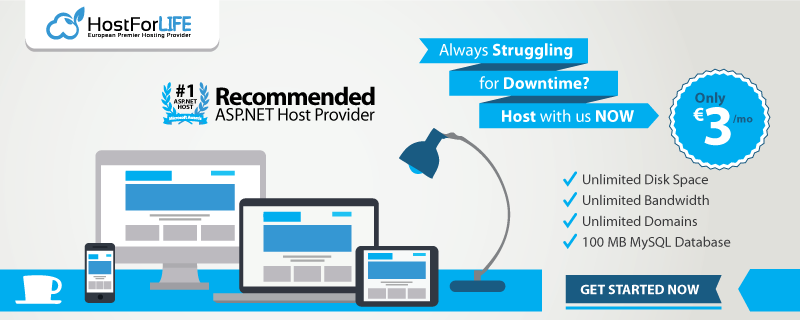