
January 16, 2024 06:25 by
Peter
FluentValidation: What Is It?
An open-source validation toolkit for.NET called FluentValidation offers a fluid interface for creating and implementing validation rules. It makes validation logic easy to comprehend, write, and maintain by enabling developers to describe it succinctly and clearly.
.NET 8's FluentValidation
Installing the FluentValidation NuGet package is required in order to use FluentValidation in.NET 8. The following command can be used to accomplish this in the.NET CLI or Package Manager Console:
dotnet add package FluentValidation
Verifying a Basic User Model
Let's construct a straightforward example in which we wish to validate a User class. To make sure the user's email address is legitimate and their name is not empty, we will use FluentValidation.
using FluentValidation;
public class User
{
public string Name { get; set; }
public string Email { get; set; }
}
public class UserValidator : AbstractValidator<User>
{
public UserValidator()
{
RuleFor(user => user.Name).NotEmpty().WithMessage("Name cannot be empty");
RuleFor(user => user.Email).NotEmpty().EmailAddress().WithMessage("Invalid email address");
}
}
In this instance:
- We provide properties for Name and Email in our User class definition.
- We construct a class called UserValidator, which is derived from AbstractValidator<User>.
- We define validation rules for the Name and Email properties inside the UserValidator constructor using the RuleFor method.
Applying FluentValidation to Your Software
Let's look at how we can utilize FluentValidation in our application now that we have our User class and matching validator:
class Program
{
static void Main()
{
var user = new User { Name = "Mahesh Chand", Email = "[email protected]" };
var validator = new UserValidator();
var validationResult = validator.Validate(user);
if (validationResult.IsValid)
{
Console.WriteLine("User is valid");
}
else
{
foreach (var error in validationResult.Errors)
{
Console.WriteLine($"Validation Error: {error.ErrorMessage}");
}
}
}
}
In this instance:
- We construct an instance of the User class and set its properties.
- We instantiate a UserValidator object.
- By handing in the User instance, we invoke the Validate method on the validator.
- We verify the validity of the validation result. We print a success message if it is. If not, we repeat the mistakes and print them.
.NET 8's FluentValidation offers a tidy and expressive approach to handling validation in your applications. You can confidently design and run validation rules thanks to its fluid interface and simple syntax. Using FluentValidation makes it possible to isolate your validation logic apart from your business logic, as seen in our example, which makes your codebase easier to read and manage.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
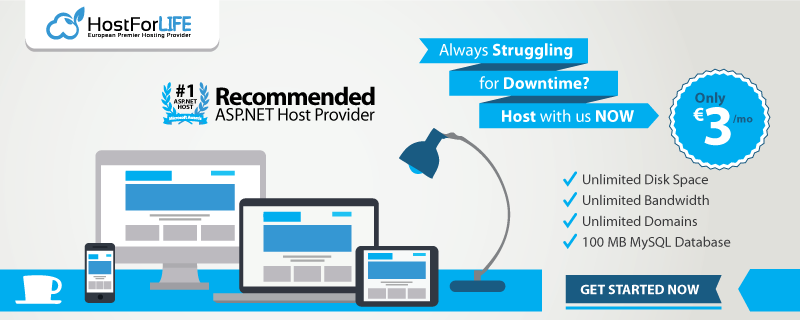