The next major release is .NET 6. It has support for MAUI, Improved SPA templates, Blazor Enhancements, Lightweight API development, and much more. In this tutorial, we are going to cover how .NET 6 will allow us to develop lightweight APIs.
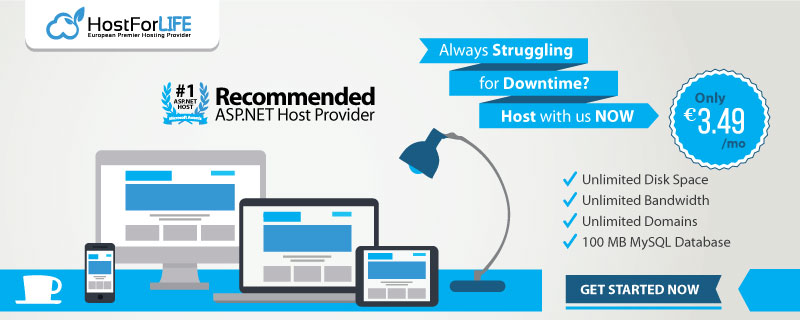
The good thing about this lightweight API is that no additional template and no extra effort are required. You can start from an empty web project and be up and running without any extra configuration. So, let’s get started with development.
Software and Packages Required
- Visual Studio Code
- .NET 6 SDK Preview 4
What we are building,
URL |
VERB |
MAP OVERLOAD |
/ |
GET |
MapGet |
/ |
POST |
MapPost |
/id |
DELETE |
MapDelete |
Solution Setup
Check .NET version
D:\LightweightDotnetAPI>dotnet --version
6.0.100-preview.4.21255.9
dotnet new sln #create new solution
dotnet new web -o Api #create API Project
dotnet sln add Api/Api.csproj #Add Project in Solution
dotnet add package Microsoft.AspNetCore.Mvc.NewtonsoftJson --version 5.0.7
The result project structure will look like this,
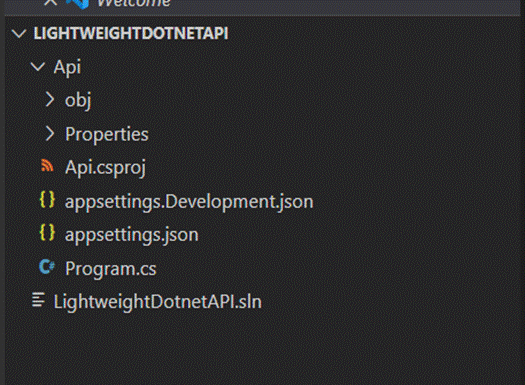
Code Walkthrough
Now, our Program.cs will be like the below file.
Program.cs
using System;
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.Hosting;
using Microsoft.AspNetCore.Http;
using Newtonsoft.Json;
using System.IO;
using System.Text;
var builder = WebApplication.CreateBuilder(args);
await using
var app = builder.Build();
if (app.Environment.IsDevelopment()) {
app.UseDeveloperExceptionPage();
}
app.MapGet("/", (Func < string > )(() => "Hello World!"));
app.MapPost("/", (Func < PostBody, string > )((body) => {
string text = JsonConvert.SerializeObject(body);
return text;
}));
app.MapDelete("/{id}", (Func < int, string > )((id) => "Delete Request for " + id));
await app.RunAsync();
public class PostBody {
public string Data {
get;
set;
}
}
That’s it, we have built an API that can accept GET, POST, and DELETE with very few lines of code. You can see in the above code that all the things have been done in one file and with minimal effort. With less overhead, we get more performance. Microservices can be built using this simple structure. It is very simple and easy to understand.
We have built a lightweight API in .NET 6 (currently in Preview). We have covered the new Routing APIs that use Map Overloads in .NET 6 starting from Preview 4. We built API using GET, POST, and DELETE verbs in the Program.cs file. The lines of code were minimal. With minimum lines of code, we have better performance. So far we have covered,
- Setting up the project in .NET CLI
- Creating lightweight API in Program.cs
- Advantages