
November 24, 2020 08:30 by
Peter
Distributed computing is an integral part of almost every software development. Before .Net Remoting, DCOM was the most used method of developing distributed application on Microsoft platform. Because of object oriented architecture, .NET Remoting replaces DCOM as .Net framework replaces COM.
Benefits of Distributed Application Development
Fault Tolerance: Fault tolerance means that a system should be resilient when failures within the system occur.
Scalability: Scalability is the ability of a system to handle increased load with only an incremental change in performance.
Administration: Managing the system from one place.
In brief, .NET remoting is an architecture which enables communication between different application domains or processes using different transportation protocols, serialization formats, object lifetime schemes, and modes of object creation. Remote means any object which executes outside the application domain. The two processes can exist on the same computer or on two computers connected by a LAN or the Internet. This is called marshalling (This is the process of passing parameters from one context to another.), and there are two basic ways to marshal an object:
Marshal by value: the server creates a copy of the object passes the copy to the client.
Marshal by reference: the client creates a proxy for the object and then uses the proxy to access the object.
Comparison between .NET Remoting and Web services
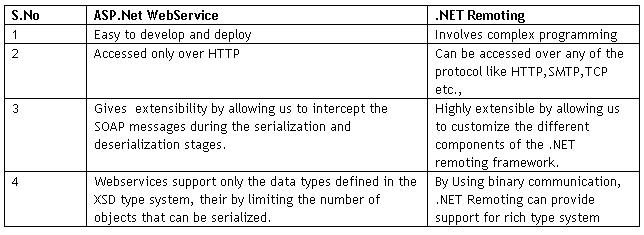
Architecture
Remote objects are accessed thro channels. Channels are Transport protocols for passing the messages between Remote objects. A channel is an object that makes communication between a client and a remote object, across app domain boundaries. The .NET Framework implements two default channel classes, as follows:
HttpChannel: Implements a channel that uses the HTTP protocol. TcpChannel: Implements a channel that uses the TCP protocol (Transmission Control Protocol). Channel take stream of data and creates package for a transport protocol and sends to other machine. A simple architecture of .NET remoting is as in Fig 1.
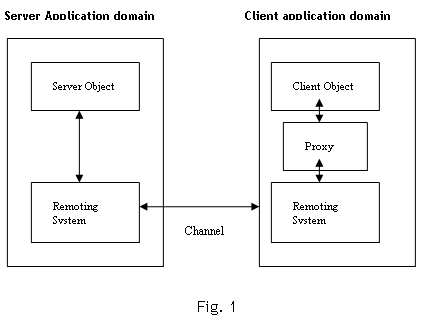
As Fig.1 shows, Remoting system creates a proxy for the server object and a reference to the proxy will be returned to the client. When client calls a method, Remoting system sends request thro the channel to the server. Then client receives the response sent by the server process thro the proxy.
Example
Let us see a simple example which demonstrates .Net Remoting. In This example the Remoting object will send us the maximum of the two integer numbers sent.
Creating Remote Server and the Service classes on Machine 1: Please note for Remoting support your service (Remote object) should be derived from MarshalByRefObject.
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinks
using System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channel
using System.IO;
namespace ServerApp {
public class RemotingServer {
public RemotingServer() {
//
// TODO: Add constructor logic here
//
}
}
//Service class
public class Service: MarshalByRefObject {
public void WriteMessage(int num1, int num2) {
Console.WriteLine(Math.Max(num1, num2));
}
}
//Server Class
public class Server {
public static void Main() {
HttpChannel channel = new HttpChannel(8001); //Create a new channel
ChannelServices.RegisterChannel(channel); //Register channel
RemotingConfiguration.RegisterWellKnownServiceType(typeof Service), "Service", WellKnownObjectMode.Singleton);
Console.WriteLine("Server ON at port number:8001");
Console.WriteLine("Please press enter to stop the server.");
Console.ReadLine();
}
}
}
Save the above file as ServerApp.cs. Create an executable by using Visual Studio.Net command prompt by, csc /r:system.runtime.remoting.dll /r:system.dll ServerApp.cs
A ServerApp.Exe will be generated in the Class folder.
Run the ServerApp.Exe will give below message on the console
Server ON at port number:8001
Please press enter to stop the server.
In order to check whether the HTTP channel is binded to the port, type http://localhost:8001/Service?WSDL in the browser. You should see a XML file describing the Service class.
Please note before running above URL on the browser your server (ServerApp.Exe should be running) should be ON.
Creating Proxy and the Client application on Machine 2
SoapSuds.exe is a utility which can be used for creating a proxy dll.
Type below command on Visual studio.Net command prompt.
soapsuds -url:http://< Machine Name where service is running>:8001/Service?WSDL -oa:Server.dll
This will generates a proxy dll by name Server.dll. This will be used to access remote object.
Client Code
using System;
using System.Runtime.Remoting.Channels; //To support and handle Channel and channel sinks
using System.Runtime.Remoting;
using System.Runtime.Remoting.Channels.Http; //For HTTP channel
using System.IO;
using ServerApp;
namespace RemotingApp {
public class ClientApp {
public ClientApp() {}
public static void Main(string[] args) {
HttpChannel channel = new HttpChannel(8002); //Create a new channel
ChannelServices.RegisterChannel(channel); //Register the channel
//Create Service class object
Service svc = (Service) Activator.GetObject(typeof(Service), "http://<Machine name where Service running>:8001/Service"); //Localhost can be replaced by
//Pass Message
svc.WriteMessage(10, 20);
}
}
}
Save the above file as ClientApp.cs. Create an executable by using Visual Studio.Net command prompt by, csc /r:system.runtime.remoting.dll /r:system.dll ClientrApp.cs
A ClientApp.Exe will be generated in the Class folder. Run ClientApp.Exe , we can see the result on Running ServerApp.EXE command prompt.
In the same way we can implement it for TCP channel also.
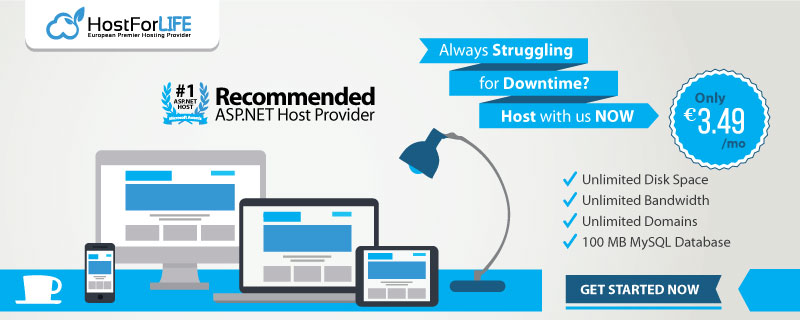