
January 22, 2024 06:21 by
Peter
To start a new ASP.NET Core Web API project, use the command line or Visual Studio.
dotnet new webapi -n WorkingwithSQLLiteinAsp.NETCoreWebAPI
cd WorkingwithSQLLiteinAsp.NETCoreWebAPI
Install SQLite NuGet Package
Install the SQLite NuGet package to enable SQLite support in your project.
dotnet add package Microsoft.EntityFrameworkCore.Sqlite
Now Create SQLite Database File in your Project
SQLLiteDatabase.db
Create Model
namespace WorkingwithSQLLiteinAsp.NETCoreWebAPI.Models
{
// Models/TodoItem.cs
public class TodoItem
{
public long Id { get; set; }
public string Name { get; set; }
public bool IsComplete { get; set; }
}
}
Now Crete Application Database Context
using Microsoft.EntityFrameworkCore;
using WorkingwithSQLLiteinAsp.NETCoreWebAPI.Models;
namespace WorkingwithSQLLiteinAsp.NETCoreWebAPI.ApplicationDbContext
{
public class AppDbContext : DbContext
{
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options) { }
public DbSet<TodoItem> TodoItems { get; set; }
}
}
Add Connection string in AppSetting.JSON File
// appsettings.json
{
"ConnectionStrings": {
"DefaultConnection": "Data Source=SQLLiteDatabase.db"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
Register the Dependancy in Program.cs File
using Microsoft.EntityFrameworkCore;
using Microsoft.OpenApi.Models;
using WorkingwithSQLLiteinAsp.NETCoreWebAPI.ApplicationDbContext;
var builder = WebApplication.CreateBuilder(args);
var configuration = builder.Configuration;
// Add services to the container.
builder.Services.AddDbContext<AppDbContext>(options =>
options.UseSqlite(configuration.GetConnectionString("DefaultConnection")));
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "Working with SQLLite In Asp.net Core Web API", Version = "v1" });
});
var app = builder.Build();
// Configure the HTTP request pipeline.
if(app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.Run();
Create and Apply Migrations
Run the following commands to create and apply migrations to create the SQLite database.
dotnet ef migrations add InitialCreate
dotnet ef database update
CRUD Operations
Implement CRUD operations in your controller.
// Controllers/TodoController.cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.EntityFrameworkCore;
using WorkingwithSQLLiteinAsp.NETCoreWebAPI.ApplicationDbContext;
using WorkingwithSQLLiteinAsp.NETCoreWebAPI.Models;
[ApiController]
[Route("api/[controller]/[Action]")]
public class TodoController : ControllerBase
{
private readonly AppDbContext _context;
public TodoController(AppDbContext context)
{
_context = context;
}
// GET: api/Todo
[HttpGet]
public async Task<ActionResult<IEnumerable<TodoItem>>> GetTodoItems()
{
return await _context.TodoItems.ToListAsync();
}
// GET: api/Todo/5
[HttpGet("{id}")]
public async Task<ActionResult<TodoItem>> GetTodoItem(long id)
{
var todoItem = await _context.TodoItems.FindAsync(id);
if(todoItem == null)
{
return NotFound();
}
return todoItem;
}
// POST: api/Todo
[HttpPost]
public async Task<ActionResult<TodoItem>> PostTodoItem(TodoItem todoItem)
{
_context.TodoItems.Add(todoItem);
await _context.SaveChangesAsync();
return CreatedAtAction(nameof(GetTodoItem), new { id = todoItem.Id }, todoItem);
}
// PUT: api/Todo/5
[HttpPut("{id}")]
public async Task<IActionResult> PutTodoItem(long id, TodoItem todoItem)
{
if(id != todoItem.Id)
{
return BadRequest();
}
_context.Entry(todoItem).State = EntityState.Modified;
try
{
await _context.SaveChangesAsync();
}
catch(DbUpdateConcurrencyException)
{
if(!TodoItemExists(id))
{
return NotFound();
}
else
{
throw;
}
}
return NoContent();
}
// DELETE: api/Todo/5
[HttpDelete("{id}")]
public async Task<IActionResult> DeleteTodoItem(long id)
{
var todoItem = await _context.TodoItems.FindAsync(id);
if(todoItem == null)
{
return NotFound();
}
_context.TodoItems.Remove(todoItem);
await _context.SaveChangesAsync();
return NoContent();
}
private bool TodoItemExists(long id)
{
return _context.TodoItems.Any(e => e.Id == id);
}
}
Output
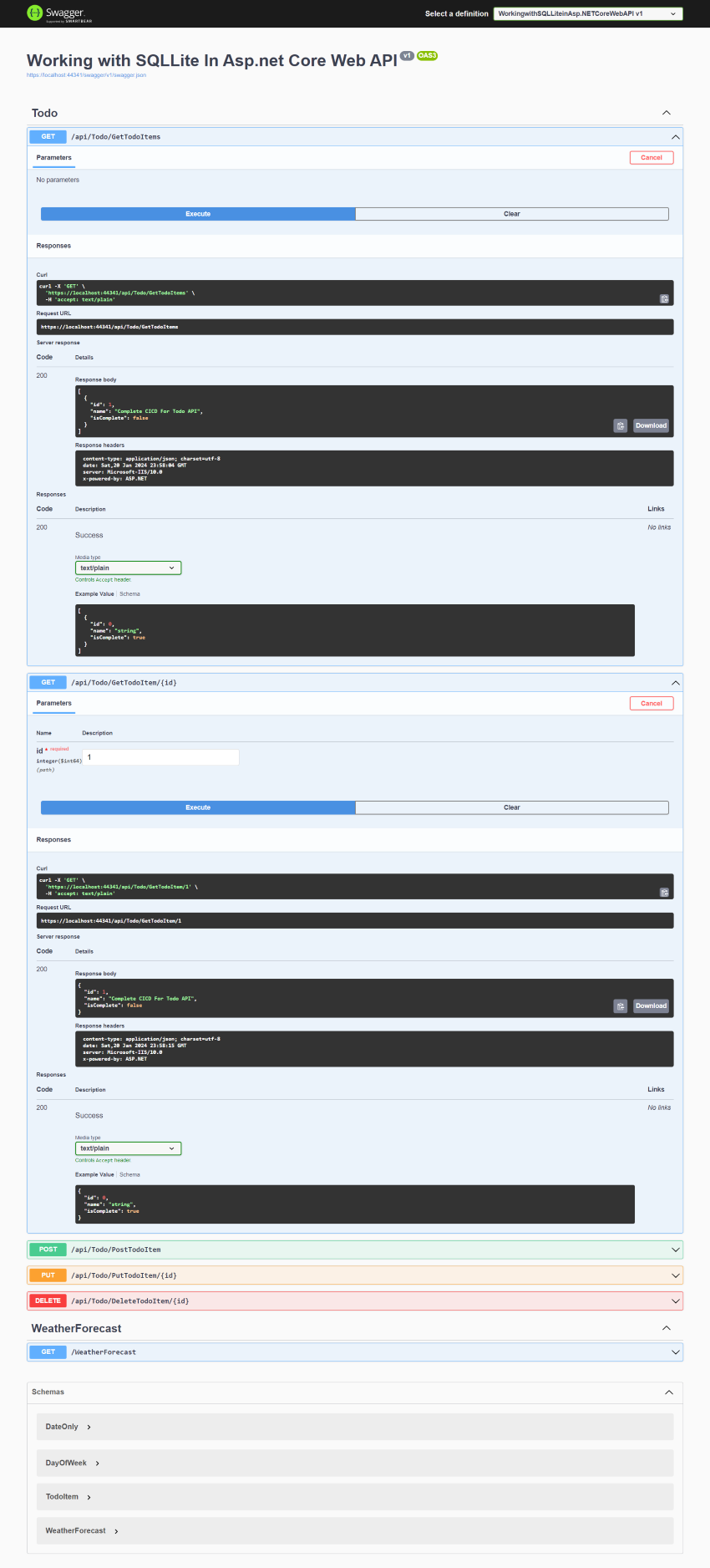
Conclusion
SQLite is a relational database management system (RDBMS) that is self-contained, serverless, and requires no configuration. It is an embedded database engine that is lightweight, open-source, and runs directly on the client device, eliminating the need for a separate server process. Some of SQLite's key features are.
- Self-contained: It is simple to install and maintain because the whole database system is housed in a single library.
- Serverless: SQLite does not function as a separate server process, in contrast to conventional database management systems. Rather, it is incorporated straight into the program.
- Zero-configuration: Complex setup or administration are not necessary for SQLite. The database is a single, straightforward file that often has a.db extension; no server setup or upkeep is required.
- Cross-platform: SQLite is compatible with a number of operating systems and mobile platforms, including iOS and Android, as well as Windows, macOS, and Linux.
- Single-user: Because SQLite is optimized for single-user applications, embedded systems, mobile apps, and lightweight desktop programs can all benefit from its use.
- ACID-compliant: SQLite preserves the ACID (Atomicity, Consistency, Isolation, Durability) qualities, guaranteeing data reliability and integrity, even with its lightweight design.
- Dynamic typing: SQLite stores data using dynamic typing, which enables users to store several kinds of data in the same column.
- Broad language support: SQLite is adaptable to a variety of development contexts since it supports a wide range of programming languages, including C, C++, Java, Python, and.NET.
SQLite is often utilized in situations where a lightweight and integrated database solution is needed, such as mobile applications, desktop software, and embedded systems, because of its simplicity, portability, and ease of integration.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
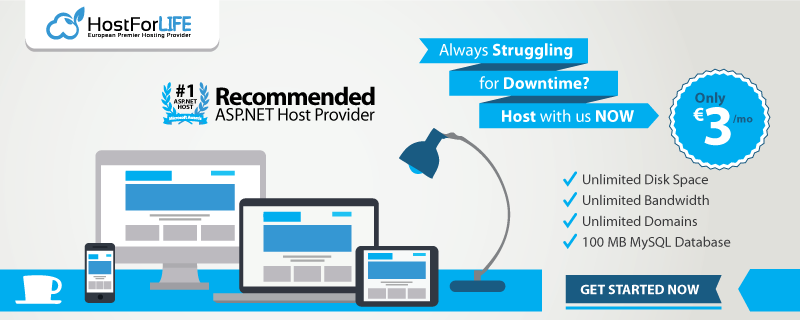