
April 14, 2025 07:19 by
Peter
A free database is provided by a Firebase database connection. In essence, we are able to perform CRUD tasks, which include Insert, Update, Get, and Delete. I've broken out how to install and create a project on the Firebase console panel here. Opening Firebase and logging into your account is the first step. The second step is to select the terminal application that opens Dashboard, click Create Project, and then enter the name of your project.
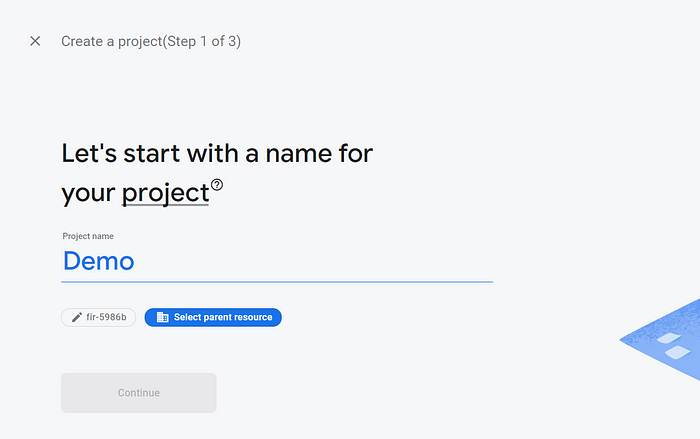
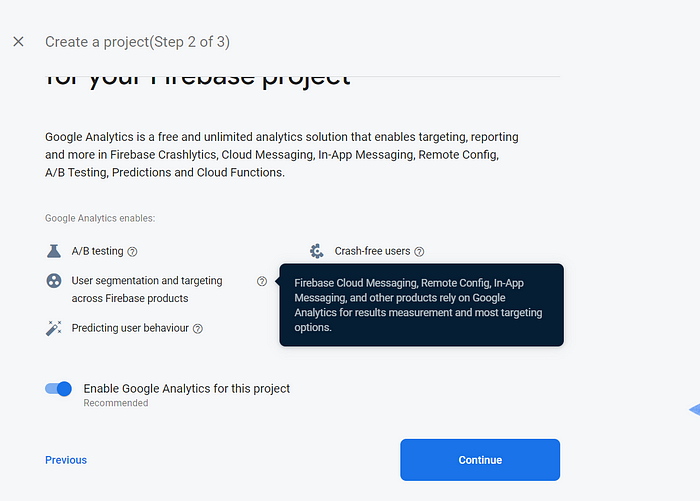
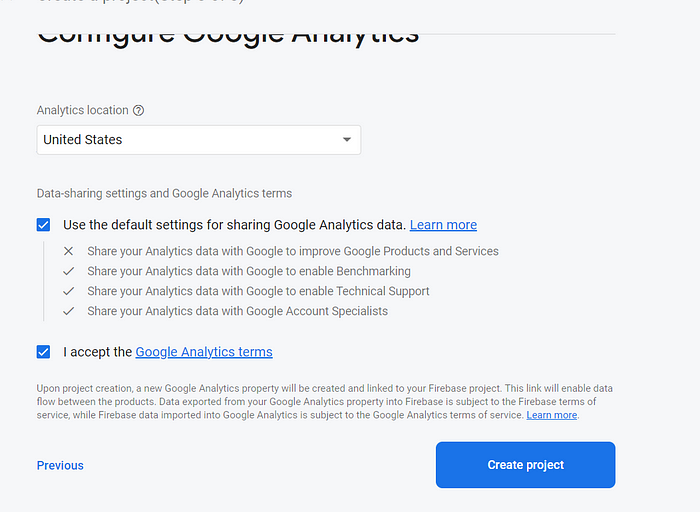
Making a real-time database is the third phase. Then select the test mode and press the "enable" button. You can now use this configuration for crude operations as your database has been built. The first step is to register for a Firebase account. I've included a link that will assist you in using the Firebase console app to create a project.
These are some steps listed below that take you to the exact place of Firebase integration with .NET. Firstly, you need to create a .net project and then install the NuGet package; and name of the package is FireSharp, and the version of the package is 2.0.4.
Now, you will need credentials to perform the CRUD operation.
To connect with your real-time database, copy the base path from the console app.
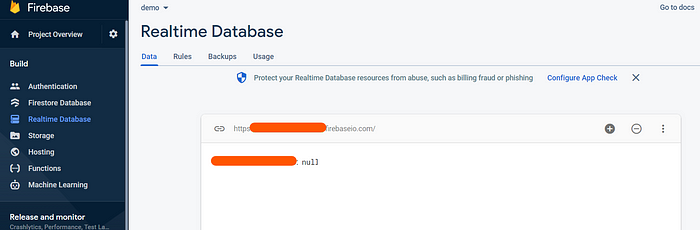
Then we need the authsecret key, that key you can fetch from the project setting > service account > database secret key.
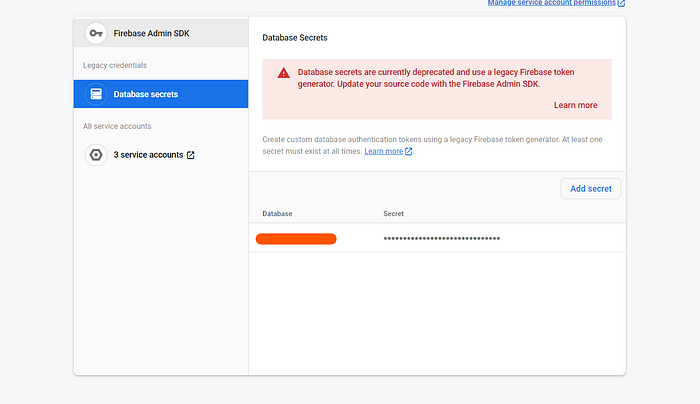
Lastly, let’s write code in .NET.
// Firebase configuration
IFirebaseConfig ifc = new FirebaseConfig()
{
AuthSecret = "**********x8Ed6HVU0YXlXW-L75ho4ps",
BasePath = "https://we****.firebaseio.com/"
};
IFirebaseClient client;
// Create a user object
User user = new User()
{
Id = 1,
FirstName = "Test 1",
LastName = "Test 2"
};
// Initialize Firebase client
client = new FireSharp.FirebaseClient(ifc);
// Insert data
var set = client.Set("User/" + user.Id, user);
// Delete data
set = client.Delete("User/" + user.Id);
// Update data
set = client.Update("User/" + user.Id, user);
// Retrieve data
set = client.Get("User/" + user.Id);
To explore more classes, please visit the official doc of Firebase Admin .NET SDK: firebase.google.com/docs/reference/admin/dotnet