
February 16, 2015 06:48 by
Peter
In this article, I will tell you how to create form validation in AngularJs and ASP.NET 5. In most of Web Apps, we require user to register on the application. I need to do some action like Form validation , so that user can not submit a Invalid form. There are too many way to validate a form in Client side.
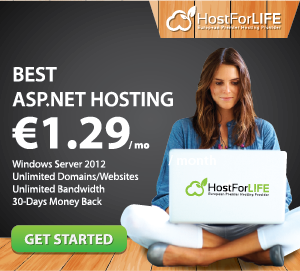
Here we are using AngularJs for the form validation. AngularJs Provide many form Validation Directives, We will use then to validate our form. Now, let’s create new project. First, Add a Employee class and write the code below:
namespace FormValidationInAj.Models
{
public class Employee
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Email { get; set; }
public int Age { get; set; }
}
}
Create an Empty Home Controller
using System.Web.Mvc;
namespace FormValidationInAj.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
}
}
Add Index view corresponding to Index action with this code:
@model FormValidationInAj.Models.Employee
<div ng-controller="myCntrl" class="divForm">
<form name="registerForm" novalidate ng-submit="Save(registerForm.$valid)">
@Html.AntiForgeryToken()
<fieldset>
<legend>Employee</legend>
<div class="editor-label">
@Html.LabelFor(model => model.FirstName)
</div>
<div class="editor-field form-group">
<input type="text" ng-model="firstName" name="firstName" ng-required="true" />
<p ng-show="registerForm.firstName.$error.required && !registerForm.firstName.$pristine" class=" error">First Name Required</p>
</div>
<div class="editor-label">
@Html.LabelFor(model => model.LastName)
</div>
<div class="editor-field form-group">
<input type="text" ng-model="lastName" name="lastName" />
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Email)
</div>
<div class="editor-field form-group">
<input type="email" ng-model="Email" name="Email" ng-required="true" />
<p ng-show="registerForm.Email.$error.required && !registerForm.Email.$pristine" class="error">Email Required</p>
<p ng-show="registerForm.Email.$error.email && !registerForm.Email.$pristine" class="error">Invalid Email</p>
</div>
<div class="editor-label">
@Html.LabelFor(model => model.Age)
</div>
<div class="editor-field form-group">
<input type="number" ng-model="Age" name="Age" ng-required="true" />
<p ng-show="registerForm.Age.$error.required && !registerForm.Age.$pristine" class="error">Age Required</p>
<p ng-show="registerForm.Age.$error.number && !registerForm.Age.$pristine" class="error">Invalid Age </p>
</div>
<p>
<input type="submit" value="Create" class="btn btn-primary" />
</p>
</fieldset>
</form>
</div>
Above I even have used AngularJs directives for form validation.
- Required :using ng-required="true", validated a input field that's required.
- Email : using Type="email" property of input field, validate Email address.
- Number : using Type="number" property of input field, validate number field.
There are several form validation directives in AngularJs.
- To show the error message, I even have used Error Name of AngularJs.
- To show error message only if used has been interacted with form, unless Error message are going to be hidden, I even have used $pristine. $pristine : it'll be true If user has not interacted with form yet.
Now, Add 2 new Js file.
module.js
var app = angular.module("myApp", []);
Controller.js
app.controller("myCntrl", function ($scope, $http) {
$scope.Save = function (Valid) {
if (!Valid) {
alert("Invalid form");
return;
} else {
alert("It's Great. Form Submitted");
}
}
});
Modilfy your _Layout.cshtml file. I have included required Js file for angularJs.
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width" />
<title>@ViewBag.Title</title>
@Styles.Render("~/Content/css")
<link href="~/Content/bootstrap.min.css" rel="stylesheet" />
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/Angular/Module.js"></script>
<script src="~/Scripts/Angular/Controller.js"></script>
<style>
.divForm{
margin: 15px 50px;
padding: 0;
width: 30%;
}
</style>
</head>
<body>
@RenderBody()
@Scripts.Render("~/bundles/jquery")
@RenderSection("scripts", required: false)
</body>
</html>
Output:
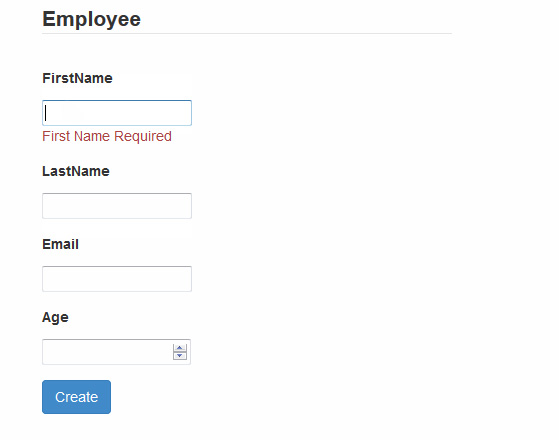
HostForLIFE.eu ASP.NET 5 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
