Hello, In.Net 7 today, I'll build the ASP.NET Web API, and I'll use the MSTest Unit Testing Project to test the module.
Microsoft's Visual Studio includes the native unit testing library MsTest.
I'll build a calculator service using the add/subtract/multiply/divide method in this tutorial. I'll make a Rest API that the Client uses to correlate to each Method.
Let's begin WebAPI Unit Testing with the.Net 7 MSTest Project.
1. From VS 2022, choose the ASP.Net Core Web API project.
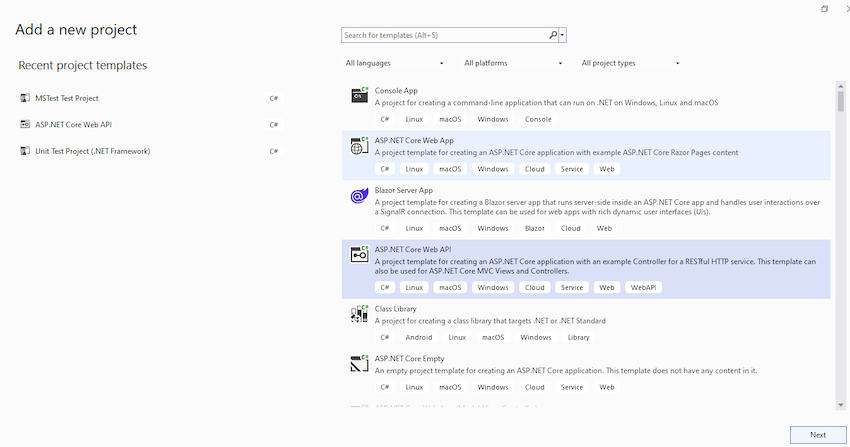
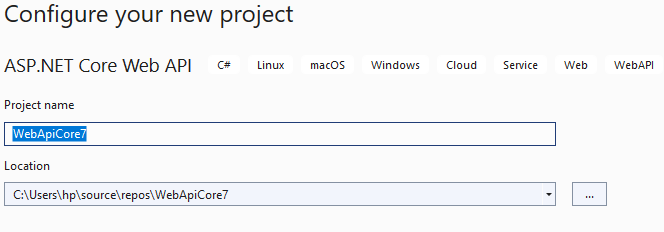
2. Use .Net7 STS
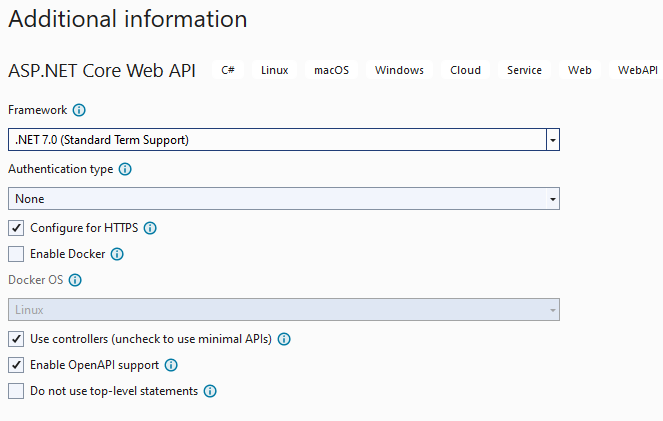
3. Now WEB API project has been created. I must add CalculatorService and ICalculatorService interface to implement the Repository Design Pattern.
public interface ICalculatorService
{
public int Add(int a, int b);
public int Subtract(int a, int b);
public int Multiply(int a, int b);
public int Divide(int a, int b);
}
public class CalculatorService:ICalculatorService
{
public int Add(int a, int b)
{
return a + b;
}
public int Subtract(int a, int b)
{
return a - b;
}
public int Multiply(int a, int b)
{
return a * b;
}
public int Divide(int a, int b)
{
return a / b;
}
}
4. Now Add Service to the Dependency Injection container.
The below three methods define the lifetime of the services,
AddTransient
Transient lifetime services are created each time they are requested. This lifetime works best for lightweight, stateless services.
AddScoped
Scoped lifetime services are created once per request.
AddSingleton
Singleton lifetime services are created the first time they are requested (or when ConfigureServices is run if you specify an instance there), and then every subsequent request will use the same instance.
But here we use AddSingleton as per requirement.
builder.Services.AddSingleton<ICalculatorService, CalculatorService>();
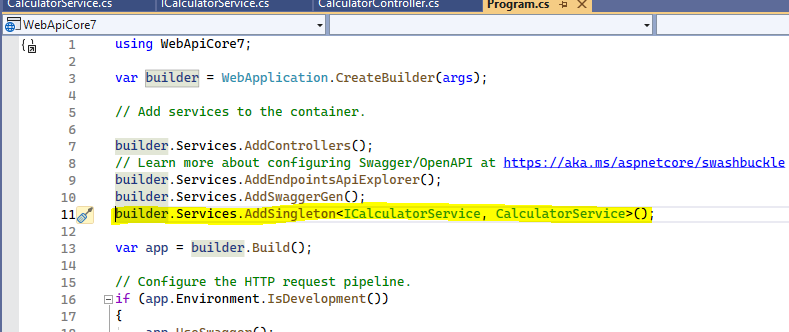
5. Create the CalculatorController and Inject ICalculatorService via Constructor Injection. And created the Add/Subtract/Multiply/Divide API Method.
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
namespace WebApiCore7.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CalculatorController : ControllerBase
{
private ICalculatorService _calculatorService;
public CalculatorController(ICalculatorService calculatorService)
{
_calculatorService = calculatorService;
}
// GET: api/<CalculatorController>
// GET api/<CalculatorController>/5
[HttpGet("Add")]
public int Get(int a, int b)
{
return _calculatorService.Add(a, b);
}
[HttpGet("Subtract")]
public int Subtract(int a, int b)
{
return _calculatorService.Subtract(a, b);
}
[HttpGet("Multiply")]
public int Multiply(int a, int b)
{
return _calculatorService.Multiply(a, b);
}
[HttpGet("Divide")]
public int Divide(int a, int b)
{
return _calculatorService.Divide(a, b);
}
}
}
6. It's time to build and run the Web API project. We will test using the SWAGGER tool.
Swagger is an open-source framework for designing and describing APIs. Swagger's journey started in 2010 when it was developed by Reverb Technologies (formerly called Wordnik) to solve the need for keeping the API design and documentation in sync. Fast forward 6 years, and Swagger has become the de-facto standard for designing and describing RESTful APIs used by millions of developers and organizations for developing their APIs, be it internal or client facing.
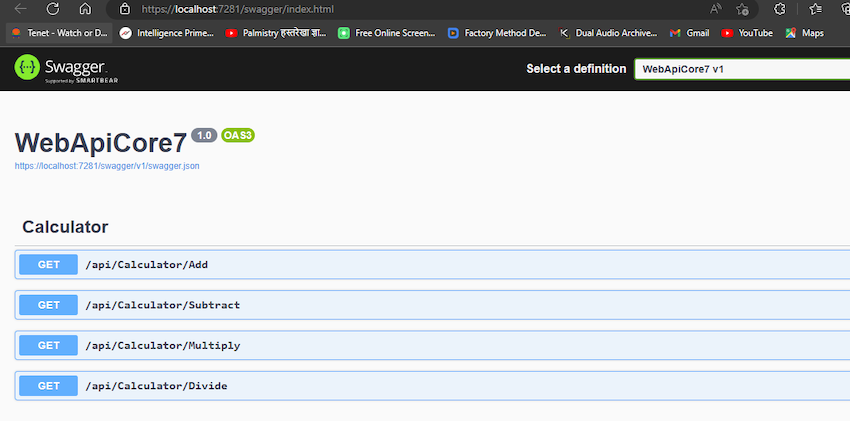
7. Testing ADD API using Swagger
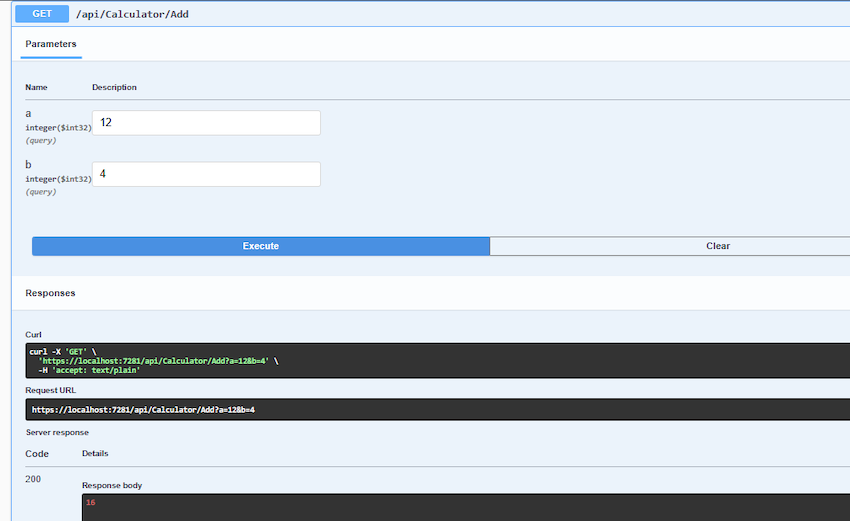
Till Now, we have developed the Web API, which implements the Calculator functionality.
It's time to add the MSUnit Test project to unit test the Calculator Service.
8. Right-click on Solution Explorer and Add Project and select MSTest Test Project.
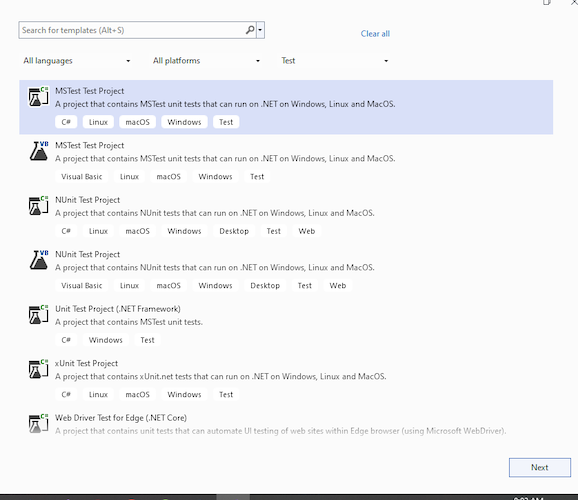
9. Set the Project Name

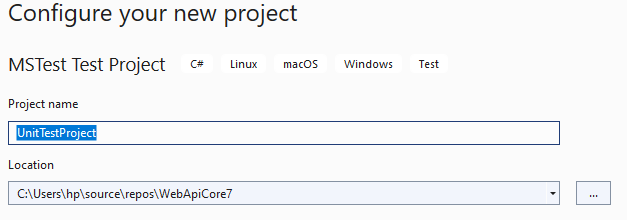
10. Set the Framework as .Net 7 STS
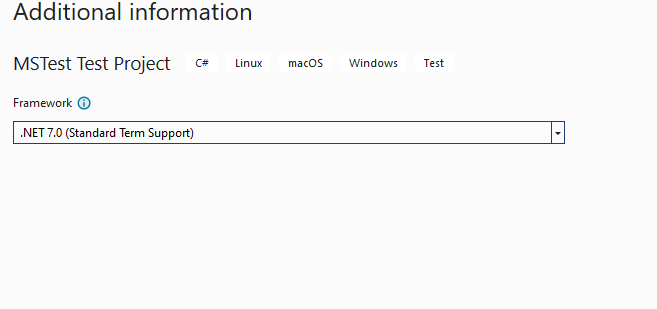
11. Right-click on the UnitTest Project and add the WebAPIcore7 Project dependency As we have to Test the Calculator Service.
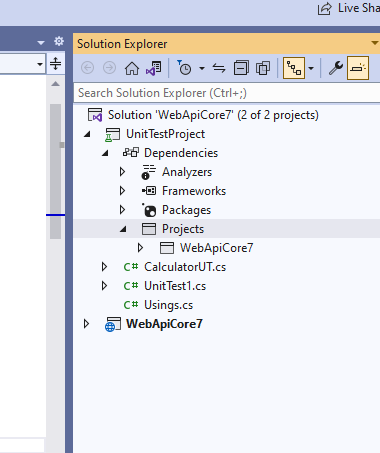
12. Add CalculatorUT Class and Decorate the class with TestClass and Method with TestMethod.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using WebApiCore7;
namespace UnitTestProject
{
[TestClass]
public class CalculatorUT
{
private ICalculatorService _calculatorService;
public CalculatorUT()
{
_calculatorService = new CalculatorService();
}
[TestMethod]
public void AddWithPass()
{
int actual = 5;
int expected = _calculatorService.Add(3, 2);
Assert.AreEqual(actual, expected);
}
[TestMethod]
public void AddWithFail()
{
int actual = 5;
int expected = _calculatorService.Add(3, 3);
Assert.AreEqual(actual, expected);
}
[TestMethod]
public void MultiplyWithPass()
{
int actual = 80;
int expected = _calculatorService.Multiply(20, 4);
Assert.AreEqual(actual, expected);
}
}
}
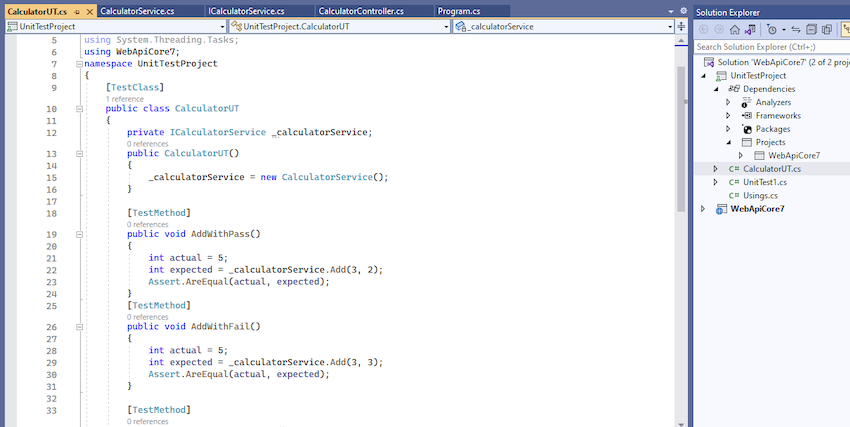
13. It's time to build and test our unit test cases.
Hit the test button on the top menu. Test showing in Green color are passed.
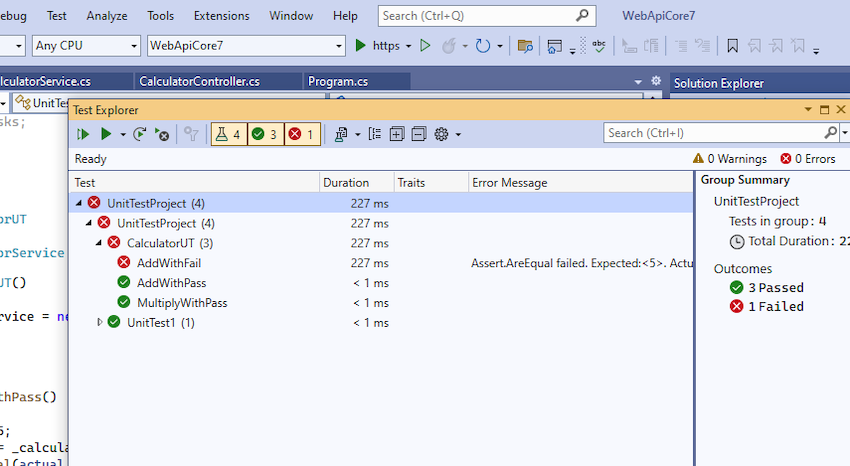
14. Those method that are tested are shown in the API project like this(Green/Red color).
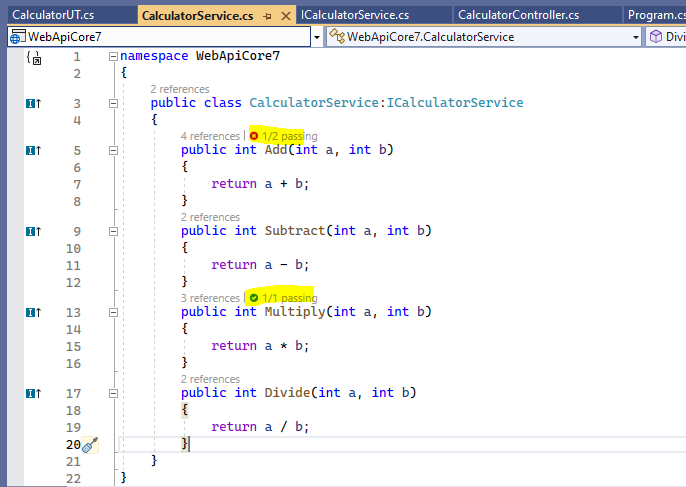
Unit testing helps identify all kinds of issues with the software at a very early stage. Software developers can then work on those issues first before progressing any further. The main advantage of this is when the issues are resolved at an early stage, no other part of the software is impacted.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
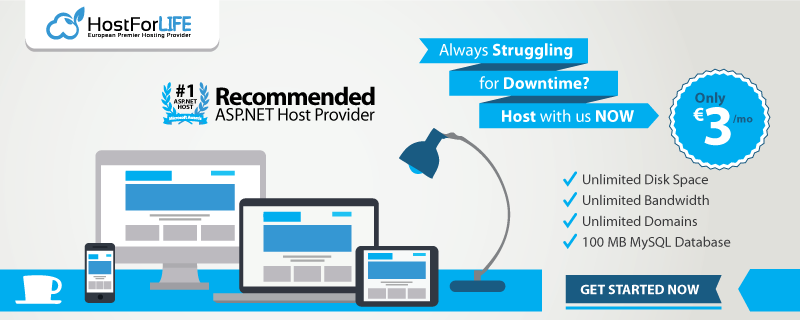