In.NET Core, access modifiers are essential for specifying how accessible classes, methods, and variables are. One of the main tenets of object-oriented programming (OOP) is encapsulation, which they assist enforce by deciding which sections of your code can access which members. The many kinds of access modifiers that are available in.NET Core will be discussed in this article, along with usage examples.
1. What are Access Modifiers?
In C#, classes, methods, properties, and other members can have their accessibility level specified using keywords called access modifiers. Access modifiers assist guard against accidental changes or misuse of your code by allowing you to manage which areas of your codebase can communicate with a specific class or member.
2. Types of Access Modifiers in .NET Core
There are five main access modifiers in .NET Core:
- Public
- Private
- Protected
- Internal
- Protected Internal
- Private Protected
Let's dive into each of these access modifiers.
3. Public Access Modifier
The public access modifier makes a class, method, or property accessible from any other code in the same assembly or another assembly that references it. It is the most permissive access level.
Example
public class Car
{
public string Color { get; set; }
public void Drive()
{
Console.WriteLine("The car is driving.");
}
}
In this example, the Car class, its Color property, and the Drive() method are all accessible from any other code.
4. Private Access Modifier
The private access modifier restricts access to the containing class only. This means that members declared as private cannot be accessed from outside the class they are declared in.
Example
public class Car
{
private string engineNumber;
public void StartEngine()
{
Console.WriteLine("The engine has started.");
}
}
Here, the engine number field is private and can only be accessed or modified by members of the Car class.
5. Protected Access Modifier
The protected access modifier allows access to the containing class and any class that derives from it. It is useful when you want to allow derived classes to access certain members of the base class.
Example
public class Vehicle
{
protected int speed;
public void Move()
{
Console.WriteLine("The vehicle is moving.");
}
}
public class Car : Vehicle
{
public void Accelerate()
{
speed += 10; // Accessible because Car inherits from Vehicle
Console.WriteLine("The car is accelerating.");
}
}
In this example, the speed field is protected, allowing the Car class to access it.
6. Internal Access Modifier
The internal access modifier limits access to the current assembly. This means that members marked as internal can be accessed by any code within the same assembly, but not from another assembly.
Example
internal class Engine
{
internal void Start()
{
Console.WriteLine("Engine started.");
}
}
Here, the Engine class and its Start() method are internal, meaning they can be accessed by any code within the same assembly but not from other assemblies.
7. Protected Internal Access Modifier
The protected internal access modifier is a combination of protected and internal. It allows access from any code within the same assembly and from derived classes in other assemblies.
Example
public class Vehicle
{
protected internal int speed;
public void Move()
{
Console.WriteLine("The vehicle is moving.");
}
}
In this example, the speed field is accessible within the same assembly and by derived classes in other assemblies.
8. Private Protected Access Modifier
The private protected access modifier is a combination of private and protected. It allows access only within the containing class or derived classes that are within the same assembly.
Example
public class Vehicle
{
private protected int speed;
public void Move()
{
Console.WriteLine("The vehicle is moving.");
}
}
Here, the speed field is accessible only within the Vehicle class and any derived classes in the same assembly.
9. Choosing the Right Access Modifier
Choosing the appropriate access modifier is important for maintaining the integrity and security of your code. Here are some guidelines:
- Use private for members that should only be accessible within the class.
- Use protected when you want derived classes to access members of the base class.
- Use internally for members that should be accessible across the same assembly but hidden from other assemblies.
- Use public for members that need to be accessible from any code.
- Use protected internal when you want to combine the accessibility of protected and internal.
- Use private protection when you want to restrict access to the containing class and derived classes within the same assembly.
10. Conclusion
Access modifiers are an essential part of object-oriented programming in .NET Core. They help you define the visibility and accessibility of your classes, methods, and variables, ensuring that your code is secure, modular, and easy to maintain. By understanding and correctly using access modifiers, you can better control the structure and behavior of your application, leading to more robust and reliable software.
When designing your classes and members, always consider the appropriate access level that aligns with the intended use of your code. This will help you avoid unintended access and maintain a clear and maintainable codebase.
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
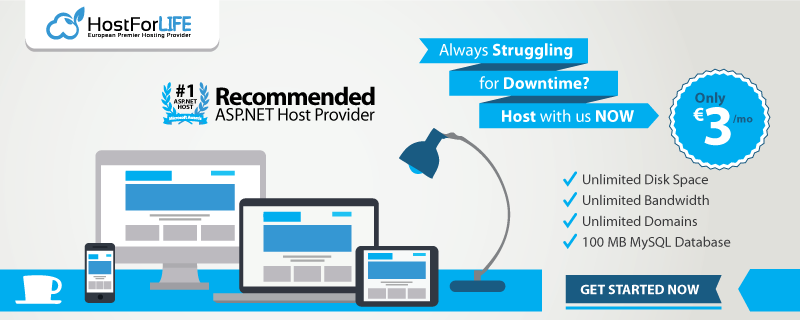