
July 16, 2021 05:43 by
Peter
As we all know, security is particularly important for all applications especially APIs as these expose our business logic to be consumed by various clients over the web. We created the .NET API server and then used Postman to call the .NET API endpoints. We attached the certificate to our request in Postman. In today’s article, we will see how to create a .NET client application which makes the call to the same .NET API server endpoint by using a certificate.
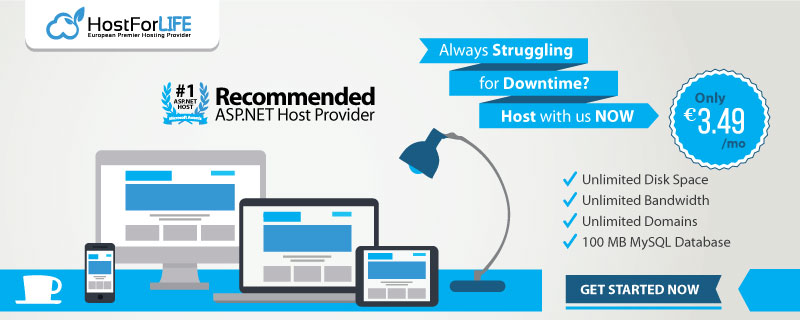
Creating the Client Application
To our existing solution, add a new C# console application project as below,
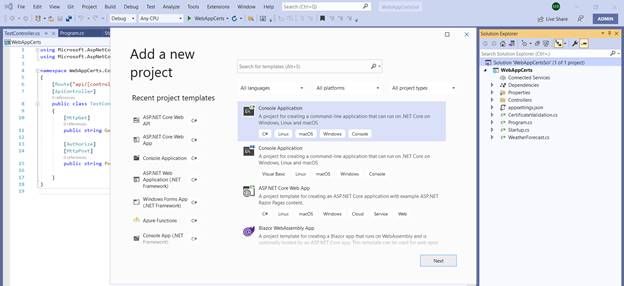
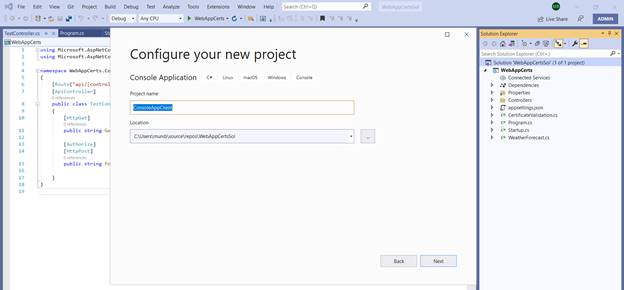
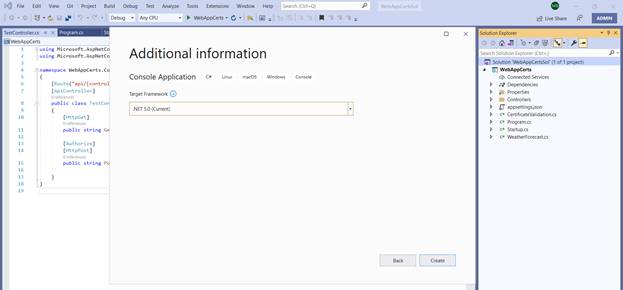
You will see the solution as below,
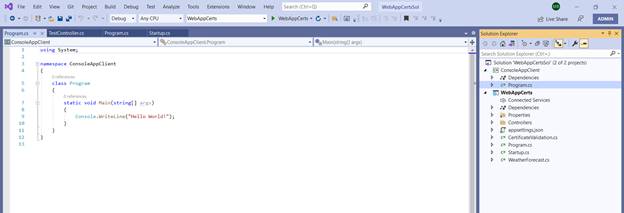
Add the below code to the “Program.cs” file,
using System;
using System.Net.Http;
using System.Security.Cryptography.X509Certificates;
var certificate = new X509Certificate2(@ "C:\CloudItems\Learning\CSharpCorner\Article 62\clientcert.pfx", "Client123");
var handler = new HttpClientHandler();
handler.ClientCertificates.Add(certificate);
using(var httpClient = new HttpClient(handler)) {
using(var response = await httpClient.PostAsync("https://localhost:5001/api/Test", null)) {
var returnValue = await response.Content.ReadAsStringAsync();
Console.WriteLine(returnValue);
}
}
Console.ReadLine();
Here, we see that we provide the client certificate and password to the client who then attaches it to the request. The server then validates the certificate and if all looks good, the request is processed. We run the server application and then the client application and make the request as below. Please note that I ran the server app from Visual Studio and the client application from the command line.
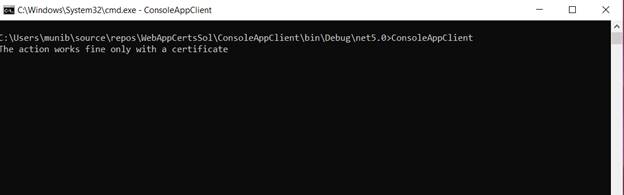
In this article, we looked at how to attach the client certificate we generated in the previous article and make an API call to the server using a .NET 5 client. Happy coding!