
December 21, 2021 06:51 by
Peter
Recently, I was getting this warning for most of the properties which are not specified as nullable. I was not happy with this warning, though I was able to run my .NET 6 application smoothly.
Exact Error:
Non-nullable property ‘propername’ must contain a non-nullvalue when exiting constructor. Consider declaring the property as nullable
Non-nullable property must contain a non-null value when exiting constructor. Consider declaring the property as nullable.
After carefully observing this error message, it makes sense for those properties. In order to minimize the likelihood that our code causes the runtime to throw System.NullReferenceException, we need to resolve this warning.
Therefore, the compiler is giving warning in the solution that the default assignment of your properties (which is null) doesn’t match its state type (which is non-null string
https://docs.microsoft.com/en-us/dotnet/csharp/nullable-references
We can resolve this error in 3 ways.
Solution 1
We can simply make the properties nullable, as shown below:
public class AppSetting {
public string? ReferenceKey { get; set; }
public string? Value { get; set; }
public string? Description { get; set; }
}
Solution 2
We can assign a default value to those properties as shown below:
public class AppSetting {
public string ReferenceKey { get; set; } = “Default Key”
public string? Value { get; set; } = “Default Value”
public string? Description { get; set; } = “Default Description”
}
Alternatively, you can give a reasonable default value for non-nullable strings as string.empty.
Solution 3
You can disable this warning from project level. You can disable by removing the below line from project file csproj or setting.
<Nullable>enable</Nullable>
Reference: https://docs.microsoft.com/en-us/dotnet/csharp/nullable-references#nullable-contexts
These are three ways you can overcome the above warning.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
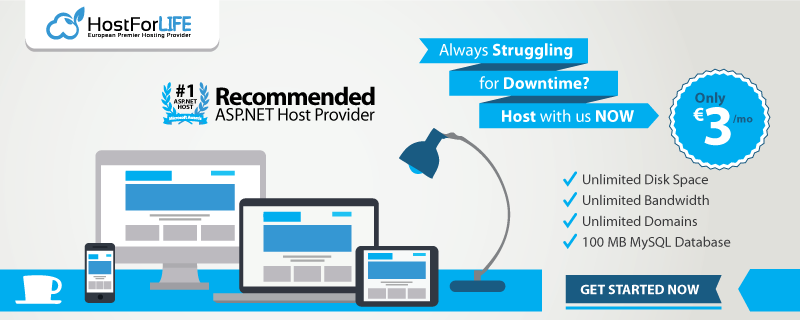