
November 16, 2021 09:01 by
Peter
React.js + .NET is an amazing stack to create scaleable and high performance full stack applications. A common scenario is when we need to send files to a server in order to save them in a cloud service like Amazon S3 or Azure blob storage.
In this article, you will learn how to send files to .NET API from a React.js application.
First, In .NET we will create and endpoint with this
[HttpPost("ImportFile")]
public async Task < IActionResult > ImportFile([FromForm] IFormFile file) {
string name = file.FileName;
string extension = Path.GetExtension(file.FileName);
//read the file
using(var memoryStream = new MemoryStream()) {
file.CopyTo(memoryStream);
}
//do something with the file here
}
If you want to try this endpoint you can use Postman with the following settings:
With this endpoint, we can read the file, all its properties and do something else like save it in Amazon S3.
Now, let's implement the client code.
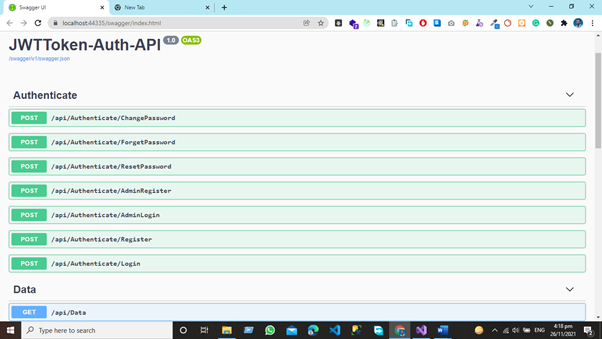
import React, { useState } from "react";
import axios from "axios";
export const FileUpload = () => {
const [fileSelected, setFileSelected] = useState();
const saveFileSelected= (e) => {
//in case you wan to print the file selected
//console.log(e.target.files[0]);
setFile(e.target.files[0]);
};
const importFile= async (e) => {
const formData = new FormData();
formData.append("file", fileSelected);
try {
const res = await axios.post("https://localhost:44323/api/importfile", formData);
} catch (ex) {
console.log(ex);
}
};
return (
<>
<input type="file" onChange={saveFileSelected} />
<input type="button" value="upload" onClick={importFile} />
</>
);
};
FormData helps us to send information with Content-Type:multipart/form-data and send files in HtmlForm format.
Also, you can add a format filter in the input file if you need it.
<input type="file" accept=".jpg, .png" onChange={saveFileSelected} />
With this approach, you can upload files to the server in a simple and save way.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
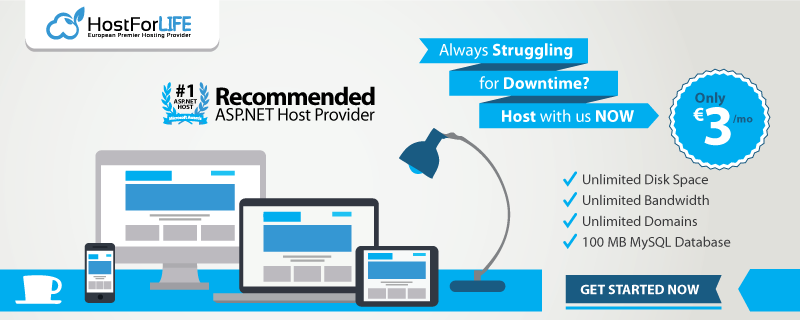