Manual Testing with Postman
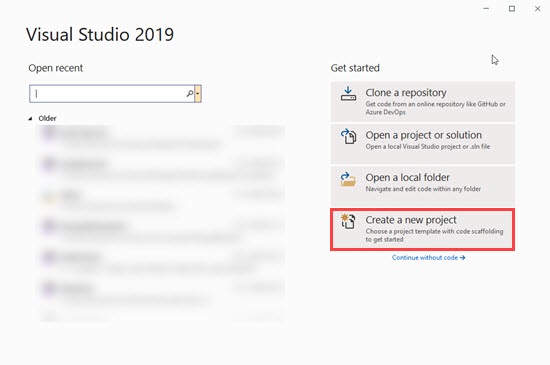
If you are a developer, tester, or a manager, sometimes understanding various methods of API can be a challenge when building and consuming the application.
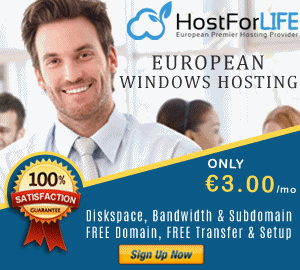
Generating good documentation and help pages for your Web API using Postman with .NET Core is as easy as making some HTTP calls.
Let’s start downloading simple To-do projects from GitHub.
Download and run the below TodoMvcSolution
Download Postman
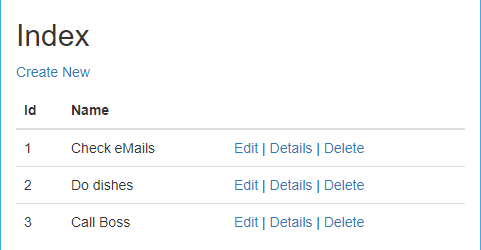
Postman is a Google Chrome application for testing API calls. You can download and install Postman from below web site.
Here are the APIs we can test - Get, Post, Put and Delete for this application.
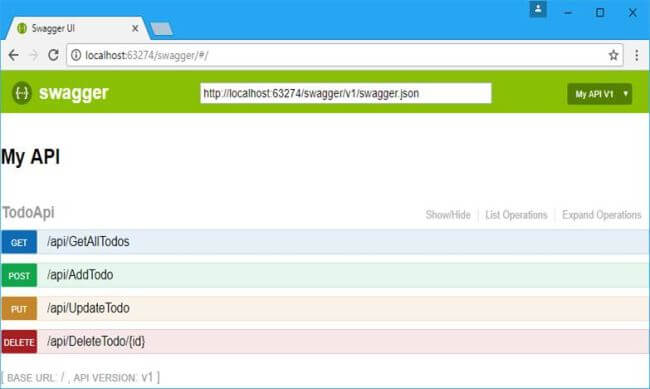
Here are the Web APIs we want to test.
//Copyright 2017 (c) SmartIT. All rights reserved.
//By John Kocer
// This file is for Swagger test, this application does not use this file
using System.Collections.Generic;
using Microsoft.AspNetCore.Mvc;
using SmartIT.Employee.MockDB;
namespace TodoAngular.Ui.Controllers
{
[Produces("application/json")]
[Route("api/Todo")]
public class TodoApiController : Controller
{
TodoRepository _todoRepository = new TodoRepository();
[Route("~/api/GetAllTodos")]
[HttpGet]
public IEnumerable<SmartIT.Employee.MockDB.Todo> GetAllTodos()
{
return _todoRepository.GetAll();
}
[Route("~/api/AddTodo")]
[HttpPost]
public SmartIT.Employee.MockDB.Todo AddTodo([FromBody]SmartIT.Employee.MockDB.Todo item)
{
return _todoRepository.Add(item);
}
[Route("~/api/UpdateTodo")]
[HttpPut]
public SmartIT.Employee.MockDB.Todo UpdateTodo([FromBody]SmartIT.Employee.MockDB.Todo item)
{
return _todoRepository.Update(item);
}
[Route("~/api/DeleteTodo/{id}")]
[HttpDelete]
public void Delete(int id)
{
var findTodo = _todoRepository.FindById(id);
if (findTodo != null)
_todoRepository.Delete(findTodo);
}
}
}
Note - Your local port number may be different than mine. Use your local port number.
http://localhost:63274/api/GetAllTodos // GET
http://localhost:63274/api/AddTodo //POST
http://localhost:63274/api/UpdateTodo //PUT
http://localhost:63274/api/DeleteTodo/5 // DELETE
Testing GET with Postman
Testing GET is very easy. First, we need to set HTTP Action from the drop-down list as GET.
Then, we need to type or paste into the API URL box.
Then, click the blue SEND button.
If the GET is successful, we see the status: 200 OK.
Testing POST with Postman
First, we need to set Http Action from the dropdown list as POST.
Then, we need to type or paste into the API URL box.
AddTodo API accepts a Todo object in JSON format. We need to pass a new Todo JSON data.
To pass JSON data we need to Select Body Tap.
Select the Raw
Select JSON(Application/JSON) as text format.
Write or paste your Todo JSON data.
Then, click the blue SEND button.
If the POST is successful, we see the status: 200 OK.
You will see Status:200 for success and the return value in the Return Body tab. We sent Publish Postman Todo item with id=0 and we received id=5 as result.
Testing PUT with Postman
First, we need to set HTTP Action from the dropdown list as PUT.
Then, we need to type or paste into the API URL.
UpdateTodo API accepts a Todo object in JSON format. We need to pass an existing Todo JSON data.
To pass JSON data we need to Select Body Tab
Select the Raw format
Select JSON(Application/JSON) as text format.
Write or paste your Todo JSON
Then click the blue SEND
If the PUT is successful, we see the status: 200 OK.
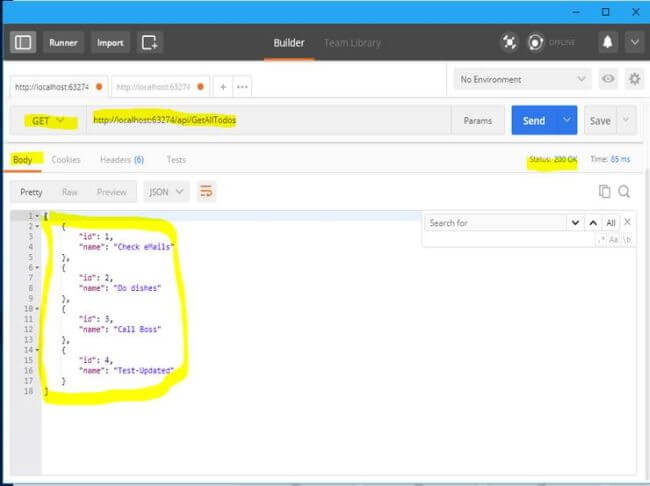
You will see Status:200 for success and the return value in the Return Body Tab. We sent Publish Postman Todo item with "name": "Publish Postman-In progress" and we receive an updated todo result.
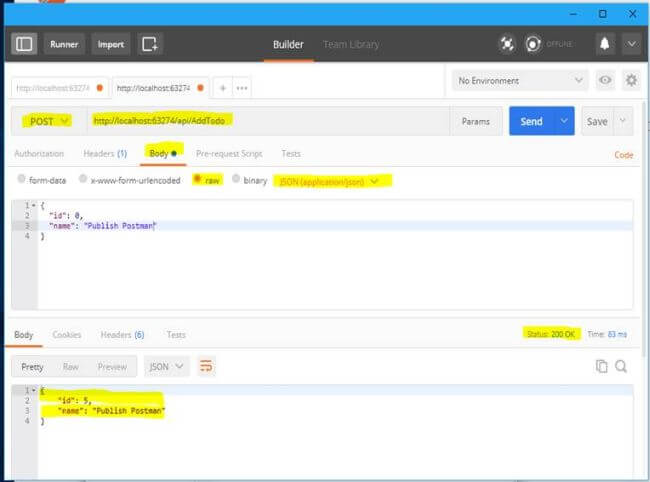
Testing DELETE with Postman
First, we need to set Http Action from the dropdown list as DELETE.
Then, we need to type or paste into the API URL box.
DeleteTodo/5 API accepts an id on the We need to pass an existing Todo with an Id value.
Then, click the blue SEND button.
If the Delete is successful, we see the status: 200 OK.
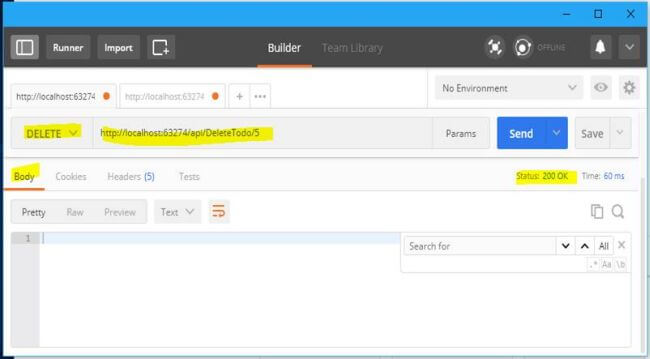
HostForLIFE ASP.NET Core 5.0.2 Hosting
HostForLIFE is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.