
February 11, 2020 11:55 by
Peter
In this blog, we will discuss 3 tag helpers: Label, Textarea and Image Tag Helper. We will also discuss how to use them in application with an example.
Label Tag Helper: The label tag helper is used for text labels in an application. It renders as an HTML label tag.
Textarea Tag Helper: The Textarea tag helper is used for Textarea of description in the application. It renders as an HTML Textarea tag.
Image Tag Helper: The Image Tag Helper renders as an HTML img tag. It is used to display images in the core applications. It provides cache-busting behavior for static image files. It has scr and asp-append-version which is set to true.
Step 1 Create an ASP.NET web application project in Visual Studio 2019.
Step 2 Create a class employee under the Models folder.
using System.ComponentModel.DataAnnotations;
namespace LabelTextareaImageTagHelper__Demo.Models
{
public class Employee
{
[Key]
public int Id { get; set; }
[Required]
public string Name { get; set; }
[Required]
public string Image { get; set; }
[Required]
[MinLength(10)]
[MaxLength(255)]
public string Description { get; set; }
}
}
Step 3 Now open index view from views than Home folder.
Step 4 Add model on top of the view to retrieve property of model class.
@model LabelTextareaImageTagHelper__Demo.Models.Employee
Step 5 Create images folder in wwwroot folder copy and paste some image to display it on browser.
<div class="form-group">
<label asp-for="Name" class="control-label"></label>
<input asp-for="Name" class="form-control" />
</div>
<div class="form-group">
<label asp-for="Image" class="control-label"></label>
<img src="~/images/855211650_154321.jpg" asp-append-version="true" height="150" width="150" class="img-thumbnail" />
</div>
<div class="form-group">
<label asp-for="Description" class="control-label"></label>
<textarea asp-for="Description" class="form-control"></textarea>
</div>
This is how it renders in browser:
<textarea class="form-control" data-val="true" data-val-maxlength="The field Description must be a string or array type with a maximum length of '255'." data-val-maxlength-max="255" data-val-minlength="The field Description must be a string or array type with a minimum length of '10'." data-val-minlength-min="10" data-val-required="The Description field is required." id="Description" maxlength="255" name="Description"></textarea>
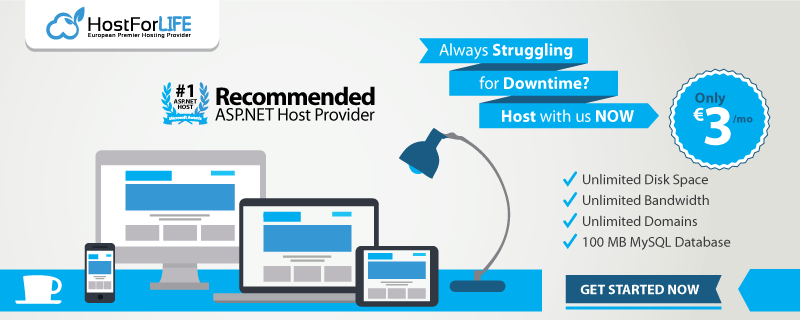