
September 17, 2014 08:09 by
Peter
Sometimes we need to do fill DropDownList using JQuery & Generic Handler in ASP.NET 4.5.2. You can see this in the example below:
Design Page:
<asp:DropDownList ID=”ddlState” runat=”server” OnPreRender=”ddlState_PreRender”
CssClass=”choose_dropdown”>
<asp:ListItem Value=”0″>SELECT YOUR STATE</asp:ListItem>
</asp:DropDownList>
<asp:DropDownList ID=”ddlProduct” runat=”server”
CssClass=”choose_dropdown”>
</asp:DropDownList>
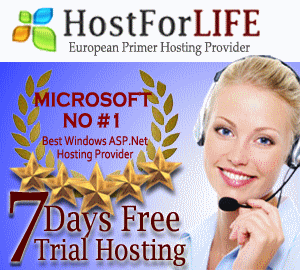
Generic Handler:
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = “application/json”;
int id = Convert.ToInt32(context.Request["id"]);
string sJSON = GetProduct(id);
context.Response.Write(sJSON);
}
public string GetProduct(int id)
{
ProductController objPrductCont = new ProductController();//My Class Name
//GetProductsByStateID(id) = It will Give All Record On The Basis Of ID
List<ProductInfo> objProduct = objPrductCont.GetProductsByStateID(id);
//Converting Object into JSON
System.Web.Script.Serialization.JavaScriptSerializer oSerializer =
new System.Web.Script.Serialization.JavaScriptSerializer();
string sJSON = oSerializer.Serialize(objProduct);
return sJSON;
}
JQuery Script:
$(“select[id$=ddlState]“).change(function () {
var statVal = $(“select[id$=ddlState]“).val();
if (statVal != “SELECT YOUR STATE”) {
var id = statVal = $(“select[id$=ddlState]“).val();// You will get the value of selected item
$.post(“/CallHandler.ashx”, { id: id }, function (result) {
$(“select[id$=ddlProduct]“).empty();
$(“select[id$=ddlProduct]“).removeAttr(“disabled”);
$(“select[id$=ddlProduct]“).attr(“style”, “cursor:default”);
$(“select[id$=ddlProduct]“).append($(“<option></option>”).val(0).html(“SELECT YOUR PRODUCT”));
$(result).each(function (i) {
$(“select[id$=ddlProduct]“).append($(“<option></option>”).val(result[i].Id).html(result[i].ProductName));
});
});
}
else {
$(“select[id$=ddlProduct]“).empty();
$(“select[id$=ddlProduct]“).append($(“<option></option>”).val(0).html(“SELECT YOUR PRODUCT”));
$(“select[id$=ddlProduct]“).attr(“disabled”, “disabled”);
return false;
}
});