
January 27, 2021 08:15 by
Peter
While developing a real-time project, we should offer different authorizations to different users. This means, for example, users who are "Admin" ,can access a timesheet, but users who are "HR" can rollout pay changes. Here we are trying to define different roles. A group of users belonging to a role will have same access or authorization. But users from different roles, will have different authorizations.
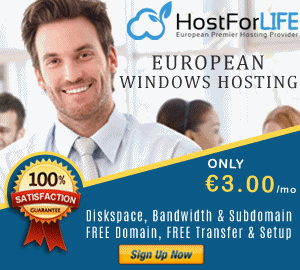
So, before hosting our application, we should define the basic roles in place. We don't expect consumers/end-users to define roles in general. We can achieve that using .Net core identity and entity framework.
Prerequisite
A .Net core project with Identity, Entity framework and language as C#.
In Entity framework , we can define default database table values before creating database model. This concept is called seeding. We are going to use seeding in order to define default users and roles here.
I have a class AppDbContext which inherits from IdentityDbContext. We will need to override OnModelCreating() method to meet seeding. Here is the sample code.
Startup.cs
public void ConfigureServices(IServiceCollection services)
{
#region database configuration
string connectionString = config.GetConnectionString("AppDb");
services.AddDbContextPool<AppDbContext>(options => options.UseSqlServer(connectionString));
services.AddIdentity<User, IdentityRole>()
.AddEntityFrameworkStores<AppDbContext>();
#endregion
}
AppDbContext.cs
public class AppDbContext : IdentityDbContext<User>
{
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
{
}
protected override void OnModelCreating(ModelBuilder builder)
{
base.OnModelCreating(builder);
this.SeedUsers(builder);
this.SeedUserRoles(builder);
}
private void SeedUsers(ModelBuilder builder)
{
User user = new User()
{
Id = "b74ddd14-6340-4840-95c2-db12554843e5",
UserName = "Admin",
Email = "[email protected]",
LockoutEnabled = false,
PhoneNumber = "1234567890"
};
PasswordHasher<User> passwordHasher = new PasswordHasher<User>();
passwordHasher.HashPassword(user, "Admin*123");
builder.Entity<User>().HasData(user);
}
private void SeedRoles(ModelBuilder builder)
{
builder.Entity<IdentityRole>().HasData(
new IdentityRole() { Id = "fab4fac1-c546-41de-aebc-a14da6895711", Name = "Admin", ConcurrencyStamp = "1", NormalizedName = "Admin" },
new IdentityRole() { Id = "c7b013f0-5201-4317-abd8-c211f91b7330", Name = "HR", ConcurrencyStamp = "2", NormalizedName = "Human Resource" }
);
}
private void SeedUserRoles(ModelBuilder builder)
{
builder.Entity<IdentityUserRole<string>>().HasData(
new IdentityUserRole<string>() { RoleId = "fab4fac1-c546-41de-aebc-a14da6895711", UserId = "b74ddd14-6340-4840-95c2-db12554843e5" }
);
}
}
After adding this piece of code, we need to build the application. Now, let's open Package Manager Console . We need to run "add-migration user_role" and "update-database" commands in order to reflect seeding changes in database.
Now lets open SQL query window just to make sure EF has updated User, Role and UserRole table. Here you go.
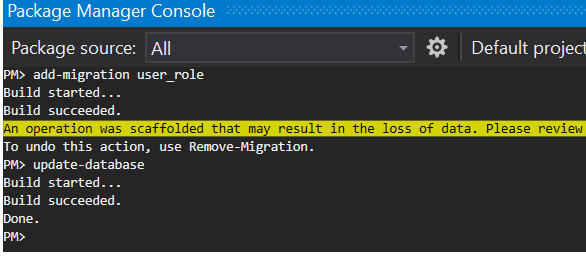
As you can see, we have default users and roles before we deploy our application.
Note
You can see few GUIDs that I have used as ID, you can customize it based on your project design.
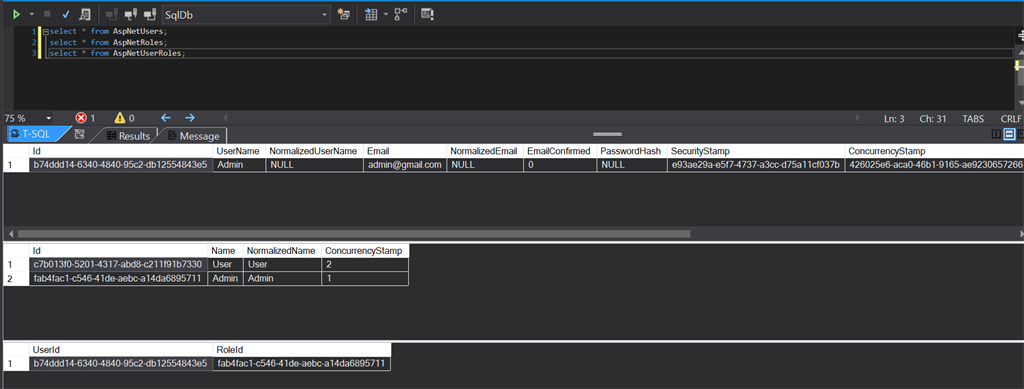
We have succeeded in seeding user and role data. Thanks for coming this far in the article. Please feel free to give your valuable comments/suggestions/feedback.