
September 29, 2021 08:20 by
Peter
The HttpRequest class is present in the System.Web namespace. During a web request, the HttpRequest class enables ASP.NET to read the HTTP values sent by a client. The method of this class is used to get the path information and save requests on the disk.
Properties and Methods of HTTPRequest Class
Following are some of the important properties of the HttpRequest class
Path
This property retrieves the virtual path of the current request.
public string Path { get; }
Code retrieves the path of the requested URL.
esponse.Write(“Path is :”+Request.Path);
Here Request.Path retrieves the path of the current URL request. The method Response.Write() displays the retrieved path.
PathInfo
This property retrieves the additional path information of the current request. It retrieves the information only for resources that have a URL extension.
Syntax is,
public string PathInfo { get };
Source code
if (Request.PathInfo == String.Empty) {
Response.Write(”PathInfo property has no info...”);
} else {
Response.Write(“ < br > PathInfo contains: < br > ”+Request.PathInfo);
}
Here String.Empty represents an empty string. The if condition checks whether PathInfo is empty. If there is no path information of the current request, Response.Write() method displays a message stating that the PathInfo property contains no information. If path information is available, the Request.PathInfo retrieves the path and Response.Write() method displays it on the web page.
UserAgent
This property retrieves the user agent string of the client browser. Consider the following example of a user agent string returned by the UserAgent property.
Mozilla/5.0(Windows;U;Windows NT 5.1; en-IN; rv:1.7.2) Geco/20050915 Firefox/2.0.6
In this example, the user agent string indicates which browser the user is using, its version number, and details about the system such as operating system and version. The web server can use this information to provide content that is defined for a specific browser. Also identifies the user’s browser and provides certain system details to servers.
The syntax for using the UserAgent property is,
public string UserAgent { get; }
For example,
Response.Write(“User Agent is :” +Request.UserAgent);
The code UserAgent property retrieves the user agent string of the client browser for the current URL request. This string is displayed on the web page using the Response.Write() method.
UserHostAddress
This property retrieves the host Internet Protocol (IP) address of the remote client who makes the request.
The IP address is a unique address used to identify a computer on a network. This address helps computers on the network to communicate with each other. 102.169.2.1 is an example of an IP address. The property UserHostAddress is used to retrieve the IP address of the client computer.
The syntax for using the UserHostAddress property is,
public string UserHostAddress { get;}
For example,
Response.Write(“User Host Address is:”+Request.UserHostAddress);
The code Request.UserHostAddress retrieves the host IP address of the remote client making the current URL request. This IP host address is displayed on web page using Response.Write() method.
TotalBytes
This property retrieves the number of bytes in the current input stream. The syntax for using the TotalBytes property is,
public int TotalBytes {get;}
Code below demonstrate the use of the TotalBytes property,
if (Request.TotalBytes > 1000) {
Response.Write(“The request is 1 KB or greater”);
}
The if condition checks if the total number of bytes in the input stream is greater than 1000. If the condition evaluates to true, Response.Write() method is displays the message “ The request is 1KB or greater” on the web page.
Following are some of the important methods of the HttpRequest class.
BinaryRead()
This method is used to retrieve the data sent to the server from the client.
The syntax is,
public byte[] BinaryRead(int count)
where count represents the number of bytes to be read. Code shows the use of the BinaryRead() method.
int intTotalBytes=Request.TotalBytes;
byte[] bCount=Request.BinaryRead(intTotalBytes);
In this code, intTotalBytes is declared as an integer variable that stores the total number of bytes in the current input stream. This number is retrieved using Request.TotalBytes. This value is then used by the BinaryRead() method to perform a binary read of the input stream and store the data that is read into a byte array named bCount.
GetType()
This method retrieves the type of the current instance.
Syntax
public Type GetType()
Where, Type is the class that represents various type declarations such as class types, array types, and interface types.
For example,
Response.Write(“<br>Type:” +Request.GetType());
The GetType() method retrieves the Type of the instance and it is displayed using the Response.Write() method.
Expectations of HttpRequest Class
Exceptions are runtime errors that occur due to certain problems encountered at the time of execution of code. When working with ASP.NET using C# , exception handling is achieved using the try-catch-finally construct. The try block encloses the statements that might throw an exception and the catch block handles the exception. The code is finally block is always executed, regardless of whether an exception occurred or not, and this code contains cleanup routines for exception situations.
When calling the BinaryRead() method of the HttpRequest class, an exception of type ArgumentException can possibly occur. This exception occurs when calling a method, if at least one of the arguments passed to the method does not meet the parameter specification.
The Path property of the HttpRequest class retrieves the virtual path of the current request. The UserHostAddress property of HttpRequest class retrieves the host IP address of the remote client making the request. GetType() also retrieves the type of the current instance.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
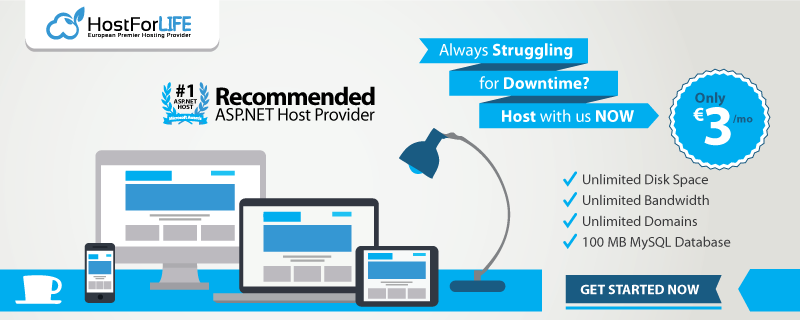