
April 10, 2023 07:33 by
Peter
ASP.NET Core is a cross-platform, high-performance, open-source framework for building modern, cloud-based, internet-connected applications. One of the core building blocks of an ASP.NET Core application is the ControllerBase class, which serves as the base class for controllers that handle HTTP requests.
What is the ControllerBase class?
The ControllerBase class is a base class for controllers in ASP.NET Core that handles HTTP requests. It provides a set of common properties and methods controllers use to handle HTTP requests and generate HTTP responses. The ControllerBase class does not implement any specific behavior or logic but instead provides a set of common methods and properties that derived classes can use.
The ControllerBase class is defined in Microsoft.AspNetCore.Mvc namespace is part of the ASP.NET Core MVC framework. It is derived from the Controller class and provides a simpler, lighter-weight alternative for building APIs and microservices.
Key Features of the ControllerBase Class
The ControllerBase class provides several key features and functionalities essential for building web applications and APIs in ASP.NET Core. Some of the most important features of the ControllerBase class are:
Action Results
The ControllerBase class provides methods for generating action results that can be returned to the client in response to an HTTP request. These action results include:ViewResult: Renders a view to generate an HTML response.
ViewResult- Renders a view to generate an HTML response
PartialViewResult- Renders a partial view to generate an HTML response
JsonResult- Serializes an object to JSON format and returns it as the response
ContentResult- Returns a string or content as the response
StatusCodeResult- Returns an HTTP status code as the response
RedirectResult- Redirects the request to a different URL
FileResult- Returns a file as the response
ObjectResult- Serializes an object to a specified format (e.g., JSON, XML) and returns it as the response.
Routing
The ControllerBase class provides a set of attributes and methods for configuring routing for controllers and actions. These include:
RouteAttribute- Used to specify the URL pattern for a controller or action
HttpGetAttribute, HttpPostAttribute, HttpPutAttribute, HttpDeleteAttribute- Used to specify the HTTP method for an action
RouteData- A property that provides access to the routing data for the current request
Model Binding
The ControllerBase class provides methods for binding HTTP request data to action parameters. These include:
FromQueryAttribute- Binds data from the query string
FromRouteAttribute- Binds data from the URL segment
FromHeaderAttribute- Binds data from an HTTP header
FromBodyAttribute- Binds data from the request body
Example Usage of the ControllerBase class
To illustrate how the ControllerBase class can be used in practice, let's look at a simple example. Suppose we want to build an API that returns a list of users. We can create a UserController class that derives from the ControllerBase class, like so:
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
[ApiController]
[Route("api/[controller]")]
public class UserController: ControllerBase {
private readonly List < string > users = new List < string > {
"Lalji Dhameliya"
};
[HttpGet]
public IActionResult GetUsers() {
return new JsonResult(users);
}
}
Conclusion
The ControllerBase class is a core building block for controllers in ASP.NET Core and provides a rich set of features and functionalities for handling HTTP requests and generating HTTP responses. By leveraging the capabilities of the ControllerBase class, developers can build powerful, high-performance web applications and APIs that are easy to maintain.
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
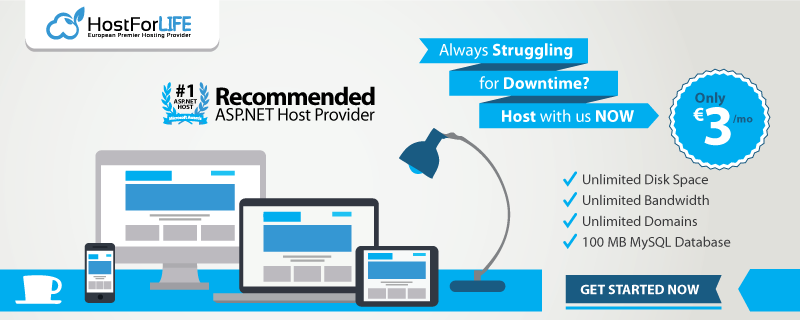