
May 23, 2023 07:52 by
Peter
I have explained how to encrypt and decrypt text using the AES Encryption standard in this article.
Open Visual Studio, Create a new console application.
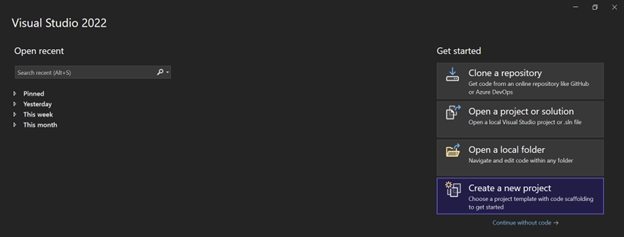
Provide a project name and choose the location to store the project information, and click next.
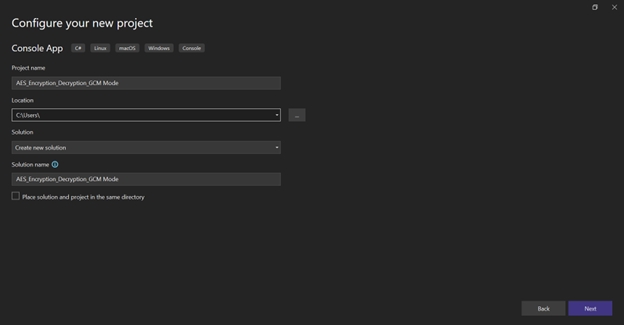
Choose the .Net framework based on your project requirement, then click Create.
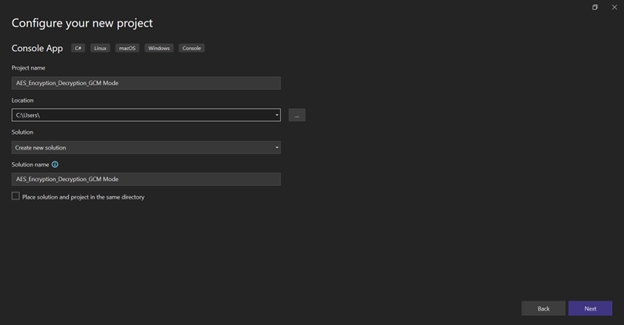
Once the project has been created, then Right Click on the project name, choose to add, and click on the new item.
Add the class file to the existing project.
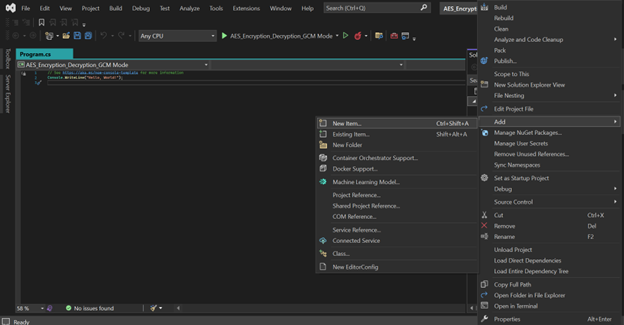
From the C# node, then choose the class definition and provide the class file name like Encrypt and decrypt file.cs.
After we added the class file to our project solution.
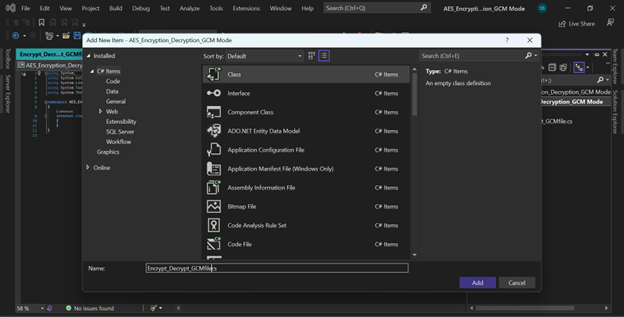
The class file was created successfully after we wrote the code in the class file.
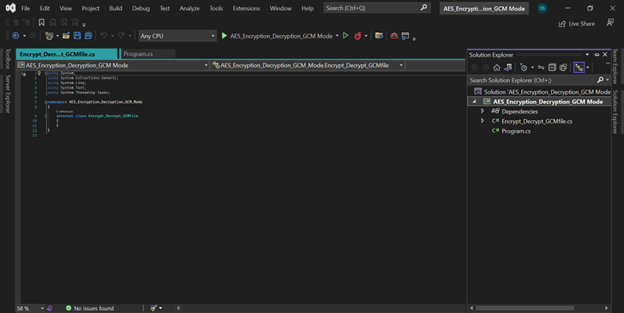
Use the below code to encrypt the given text input.
public static string EncryptString(string plainText)
{
byte[] array;
using (Aes aes = Aes.Create())
{
aes.Padding = PaddingMode.PKCS7;
aes.KeySize = 256;
aes.Key = new byte[32];
aes.IV = new byte[16];
aes.Padding = PaddingMode.PKCS7;
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
using (MemoryStream memoryStream = new MemoryStream())
{
using (CryptoStream cryptoStream = new CryptoStream((Stream)memoryStream, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter streamWriter = new StreamWriter((Stream)cryptoStream))
{
streamWriter.Write(plainText);
}
array = memoryStream.ToArray();
}
}
}
return Convert.ToBase64String(array);
}
Use the below code to decrypt the given text input.
public static string DecryptString(string cipherText)
{
byte[] buffer = Convert.FromBase64String(cipherText);
using (Aes aes = Aes.Create())
{
aes.Padding = PaddingMode.PKCS7;
aes.KeySize = 256;
aes.Key = new byte[32];
aes.IV = new byte[16];
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (MemoryStream memoryStream = new MemoryStream(buffer))
{
using (CryptoStream cryptoStream = new CryptoStream((Stream)memoryStream, decryptor, CryptoStreamMode.Read))
{
using (StreamReader streamReader = new StreamReader((Stream)cryptoStream))
{
return streamReader.ReadToEnd();
}
}
}
}
}
Then open the "Program.cs" file to consume the encryption and decryption method.
using System;
using Encrypt__Decrypt__AES_Operation__file;
namespace EncryptionDecryptionUsingSymmetricKey
{
class Program
{
public static void Main(string[] args)
{
while (true)
{
ProcessEncryptDecrypt();
}
}
public static void ProcessEncryptDecrypt()
{
int iChoice = 0;
string strPwd = string.Empty;
var encryptedString = string.Empty;
Console.WriteLine("Enter your choice:");
Console.WriteLine("1.Decryption 2.Encryption 3.Exit ");
Console.WriteLine("...............");
iChoice = Convert.ToInt32(Console.ReadLine());
if (iChoice == 1)
{
Console.WriteLine("Enter the Password:");
strPwd = Convert.ToString(Console.ReadLine());
encryptedString = AesOperation.EncryptString(strPwd);
Console.WriteLine($"encrypted string : {encryptedString}");
}
else if (iChoice == 2)
{
Console.WriteLine("Enter the Password:");
strPwd = Convert.ToString(Console.ReadLine());
var decryptedString = AesOperation.DecryptString(strPwd);
Console.WriteLine($"decrypted string : {decryptedString}");
}
else
{
Environment.Exit(0);
}
}
}
}
After the successful implementation of the above code, run the application; the output seems like the below screenshot.
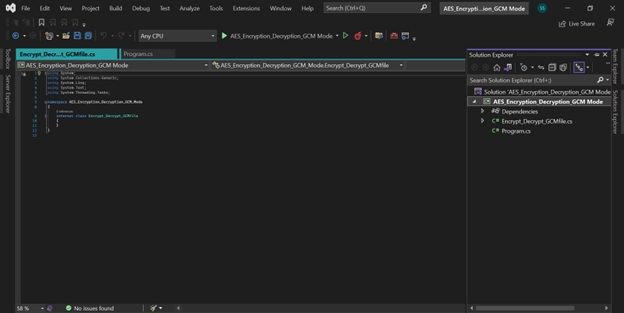
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
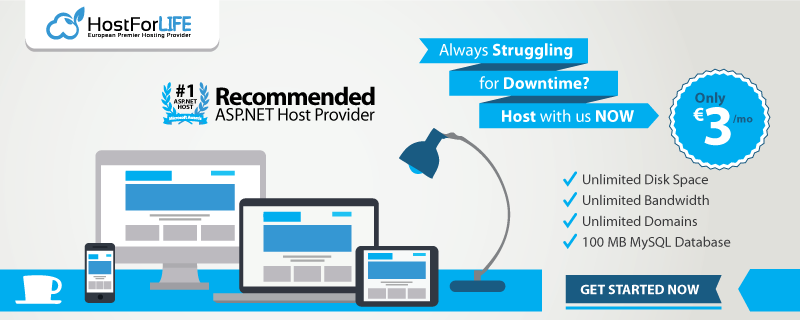