
December 12, 2022 06:59 by
Peter
In this article, we will learn about how to post file and data with the use of .Net Core WebAPI And Angular.
We can better understand this step by step.
Step 1
First we will create one model in Angular Application like,
export interface CreateDocument {
Id: number,
firstName: string,
lastName: string,
uploadedPhotoorDocName: string[]
}
Step 2
Now first of all selected file put into file array like
2.1 Create File Array,
public filecontent: File[] = [];
2.2 Angular Html template bind event,
<input id="file" class="form-control" type="file" multiple name="uploadedDocumentFile" [(ngModel)]="createDocument.uploadedDocumentFile" (change)="onFileSelected($event)" />
2.3 Selected File put into filecontent array when user select multiple files,
onFileSelected(e: any) {
for (var i = 0; i < e.target.files.length; i++) {
this.filecontent.push(e.target.files[i]);
}
}
Step 3
All file and model data put into FormData object
3.1 First create FormData object like,
let myFormData: FormData = new FormData();
3.2 Now one by one push all model value into FormData Object
myFormData.append("Id", "1001");
myFormData.append("firstName", "xyz");
myFormData.append("lastName", "abc");
3.3 All push selected file into FormData Object like
let files: File[] = this.filecontent;
for (let i = 0; i < files.length; i++) {
let file: File = files[i];
myFormData.append("files", file, file.name); // the filed name is `files` because the server side declares a `Files` property
}
3.4 If pass array value in FormData object then convert as JSON.stringify() array like
let docname = ["testing.doc", "testing1.doc", "testing2.doc"];
myFormData.append("uploadedPhotoorDocName", JSON.stringify(docname));
Step 4. Call API Form Angular
4.1 Pass Formdata into service.
this.servicename.CreateOrUpdate(myFormData).subscribe((dataResult: CommonResponse) => {
if (dataResult && dataResult.status == true) {}
});
4.2 Service call API like,
createOrUpdate(createDocument:FormData): Observable<CommonResponse> {
return this.http.post<CommonResponse>(`${environment.apiUrl}` + this.getUrl + "/Save",createDocument)
.pipe(
catchError(this.handleError('Error', []))
);
}
Step 5
Now we implement POST API .NET Core
5.1 First of all create model,
public class DemoModel {
public int Id {
get;
set;
}
public string ? FirstName {
get;
set;
}
public string ? LastName {
get;
set;
}
public string[] UploadedPhotoorDocName {
get;
set;
}
public IList < IFormFile > Files {
get;
set;
}
}
[HttpPost]
[Route("SaveSiteDocument")]
public IActionResult SaveSiteDocument([FromForm] DemoModel demoModel) {
string webRootPath = new CommonHelper(_webHostEnvironment).GetRootPath();
string saveFilePath = webRootPath + "//" + basePath + "//" + siteName + "_" + demoModel.FirstName;
SiteDocumentServices siteDocumentServices = new SiteDocumentServices();
siteDocumentServices.CreateDirectory(saveFilePath);
for (int i = 0; i < demoModel.uploadedPhotoorDocName.Length; i++) {
string strDocumentFile = demoModel.UploadedPhotoorDocName[i];
string fileName = System.IO.Path.GetFileName(strDocumentFile);
bool result = siteDocumentServices.SaveFile(strDocumentFile, saveFilePath, fileName);
demoModel.Id = demoModel.Id;
demoModel.FirstName = demoModel.FirstName;
demoModel.LastName = demoModel.LastName;;
demoModel.UploadedPhotoorDocName = demoModel.UploadedPhotoorDocName[i];;
_siteDocumentManager.SaveSiteDocument(siteDocumentModel);
}
var response = new {
Message = "records saved successfully",
Status = true,
HasException = false,
data = message
};
return (IActionResult) Ok(response);
}
Conclusion
Nowadays some applications require post data with some documents, Images. So this article is very helpful to save data and file from database and store files in server folder.
Enjoy Coding !!!!
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
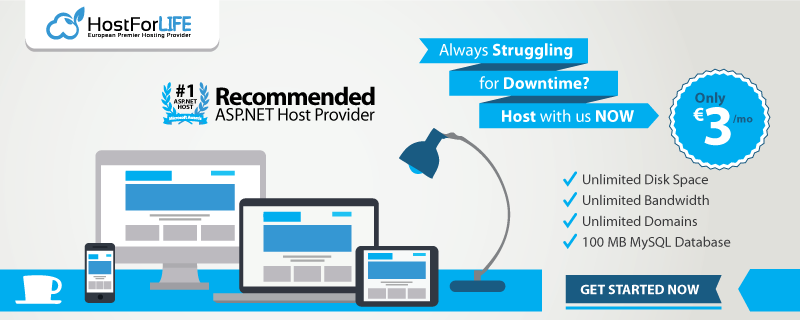