
April 28, 2025 08:46 by
Peter
In a world where software needs to be message-driven, elastic, resilient, and responsive, traditional thread-based and monolithic designs frequently break under complexity or stress. Reactive systems excel in this situation, and Akka.NET makes it not only feasible but also pleasurable to design them in C# with.NET 9. Originally created for the JVM and then transferred to.NET, Akka.NET is an open-source toolkit built on the actor paradigm. It makes it simple to create distributed, concurrent, and fault-tolerant systems.
This post will explain why Akka.NET is the greatest option for developing reactive systems in.NET 9 and provide a step-by-step demonstration of a practical example.
Why Choose Akka.net for Reactive Systems?
Akka.NET is an open-source .NET framework that implements the actor model — a proven approach to building concurrent systems. Each actor is an independent unit that processes messages asynchronously and maintains its own internal state.
Core Features of Akka.NET
Asynchronous by default: Actors process messages concurrently without locking.
- Resilient architecture: Supervision strategies handle failures gracefully.
- Scalable: Easily scale horizontally using Akka.Cluster and Cluster.Sharding.
- Decoupled components: Built on message passing, not shared state.
- Built-in support for persistence, routing, and remote messaging.
Akka.NET allows you to build applications that are not only scalable and efficient but also fault-tolerant by design.
Step-by-Step: Building a Reactive System with Akka.NET
Let’s create a simple reactive banking system where.
- Users can deposit, withdraw, and check their balance.
- Each operation is handled as a message by an actor.
Step 1. Create a New Console App
dotnet new console -n AkkaReactiveBank
cd AkkaReactiveBank
Step 2. Add Akka.NET NuGet Package
dotnet add package Akka
Step 3. Define Actor Messages
Create a new file BankMessages.cs.
public record Deposit(decimal Amount);
public record Withdraw(decimal Amount);
public record CheckBalance;
These represent the commands sent to the actor.
Step 4. Create the Bank Actor
Create a new file BankActor.cs.
using Akka.Actor;
using System;
public class BankActor : ReceiveActor
{
private decimal _balance;
public BankActor()
{
Receive<Deposit>(msg => HandleDeposit(msg));
Receive<Withdraw>(msg => HandleWithdraw(msg));
Receive<CheckBalance>(_ => HandleCheckBalance());
}
private void HandleDeposit(Deposit msg)
{
_balance += msg.Amount;
Console.WriteLine($"[Deposit] Amount: {msg.Amount}, New Balance: {_balance}");
}
private void HandleWithdraw(Withdraw msg)
{
if (_balance >= msg.Amount)
{
_balance -= msg.Amount;
Console.WriteLine($"[Withdraw] Amount: {msg.Amount}, Remaining Balance: {_balance}");
}
else
{
Console.WriteLine("[Withdraw] Insufficient funds.");
}
}
private void HandleCheckBalance()
{
Console.WriteLine($"[Balance] Current Balance: {_balance}");
}
}
Step 5. Set Up the Actor System in the Program.cs
Replace the content of the Program.cs.
using Akka.Actor;
class Program
{
static void Main(string[] args)
{
using var system = ActorSystem.Create("BankSystem");
var bankActor = system.ActorOf<BankActor>("Bank");
bankActor.Tell(new Deposit(1000));
bankActor.Tell(new Withdraw(200));
bankActor.Tell(new CheckBalance());
Console.ReadLine();
}
}
Step 6. Run the Application
dotnet run
Output
[Deposit] Amount: 1000, New Balance: 1000
[Withdraw] Amount: 200, Remaining Balance: 800
[Balance] Current Balance: 800
You’ve just created a reactive banking system with Akka.NET in .NET 9.
European Best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
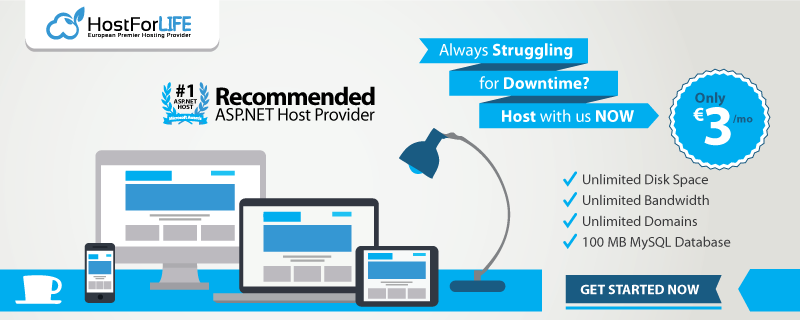